Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial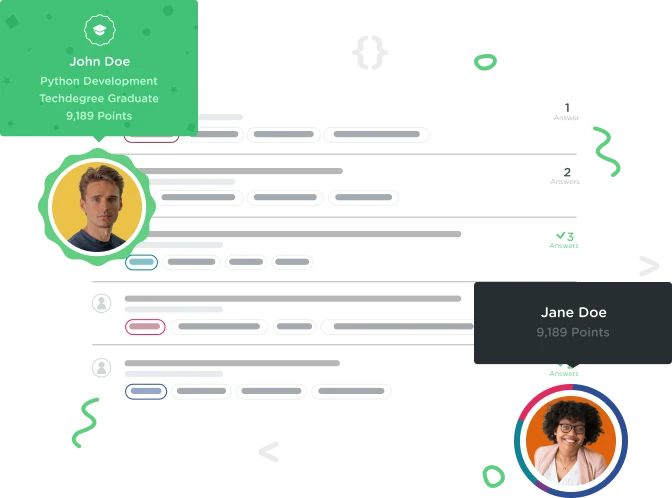

Conner Mack
7,309 Pointsim on challenge task 4 of 4
not sure whats wrong here
public class Forum {
private String topic;
public Forum(String topic){
this.topic = topic; // <--- same here :)
}
public String getTopic() {
return topic;
}
/* Uncomment this when you are prompted to do so
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = firstName; // <--- your code will be different here
this.lastName = lastName; //<---- and here
}
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost(User author, String title, String description){
this.author = author; //<--- your code will be different here
this.title = title; // <---- and here
this.description = description; //<---- aaand here
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
String firstName = args[0];
String lastName = args[1];
User author = new User(firstName, lastName); //<----- all cruicial points marked with comments
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "Programming is awesome", "Cuz it makes us create new stuff");
forum.addPost(post);
}
}
1 Answer
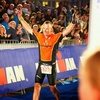
Steve Hunter
57,712 PointsHi there,
I checked your code and it passes the challenge - you must have a glitch at your end.
Steve.