Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial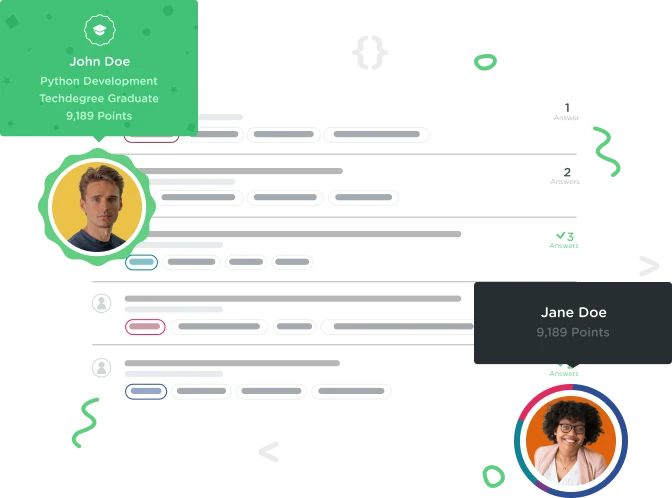

Michael Sawyer
2,086 PointsI'm on the 3rd objective in the Method Overloading section of the C# objects course and need some help solving it.
The question is "Add another method named EatFly that takes two integer parameters and returns a boolean value. Name the parameters distanceToFly and flyReactionTime. Return true if the frog’s tongue is longer or equal to than the distance to the fly and its reaction time is faster or equal to than the fly’s reaction time."
My code is below...I don't believe I needed to add the public readonly int flyReactionTime or add it to the Frog constructor and initialize it but I did to see if that was the hold up but it wasn't. I watched the video several times and I'm not sure where I'm going wrong so if someone could explain it better I would appreciate it, thanks!
namespace Treehouse.CodeChallenges { class Frog { public readonly int TongueLength; public readonly int ReactionTime; public readonly int flyReactionTime;
public Frog(int tongueLength, int reactionTime, int flyReactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
FlyReactionTime = flyReactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime)
{
return TongueLength >= distanceToFly;
return ReactionTime <= flyReactionTime;
}
}
}
5 Answers

andren
28,558 PointsThe main problem with your code is the fact that you are using two return statements, once a return statement is hit the code exits the function returning whatever you specified, that means that no code placed in a function after a return statement is hit will ever run.
On top of that even if two returns where allowed the task asks you to return true only if both of those statements are true, with the way your code is written you would return true if either of them were correct.
The solution is actually pretty simple, write a single statement that checks that both of the values are true, using the && operator and return the result of that, like this:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime) {
return TongueLength >= distanceToFly && ReactionTime <= flyReactionTime;
}
}
}

Andrew Goddard
40,807 PointsHi everyone - out of interest, why was it necessary to create a second EatFly method? My first instinct was to just extend the first method but that was wrong. Was it just that the question was giving a chance to practice creating methods or is there something more fundamental that meant the second method was necessary?

nfs
35,526 PointsWell, I believe I have found a better solution than the one provided by andren. Here's my solution:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public readonly int ReactionTime;
public Frog(int tongueLength, int reactionTime)
{
TongueLength = tongueLength;
ReactionTime = reactionTime;
}
public bool EatFly(int distanceToFly)
{
return TongueLength >= distanceToFly;
}
public bool EatFly(int distanceToFly, int flyReactionTime) {
return EatFly(distanceToFly) && ReactionTime <= flyReactionTime;
}
}
}
As the Code Challenge was about Method overloading, I think the intention behind the question was the answer I provided above. But the test passes with this code too
return TongueLength >= distanceToFly && ReactionTime <= flyReactionTime;
because they are actually the same thing, except one uses method overloading, while the other doesn't. Hope that makes sense. Thank you @andren, I was stuck on this one for quite a while. 
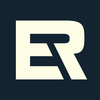
Eric Ridolfi
19,417 PointsWhy would the frog's reaction time be less than or equal to and not greater than or equal to or just equal to the fly's reaction time? If the frog's reaction time is less than the fly's, the fly would get away?

andren
28,558 PointsI had a similar thought the first time I went though the task, but thinking about it a bit more I realized that the task's logic is correct. The reaction time measures how long it takes for something to react. Having a smaller reaction time means you react faster, meaning the lower your reaction time the better.
If the fly had a faster reaction time than the frog then it could get away before the frog even notice it was present. That's why the frog has to have a faster (smaller) reaction time than the fly.

Michael Sawyer
2,086 PointsThanks, I appreciate it! I thought I had that originally and it told me I was wrong.