Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial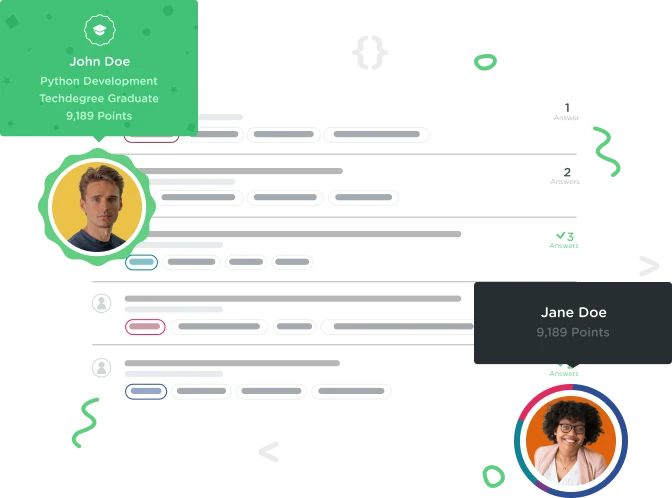

Strange Learning
Treehouse Guest TeacherI'm producing the proper morse string in the Workspace with a few different trials and can't figure out my error.
It would be great to have a more helpful error than "Bummer: Try Again"
Any help would be appreciated :)
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def convert(self, c):
if c is '.': return 'dot'
elif c is '_': return 'dash'
else: return None
def __str__(self):
return '-'.join(list(filter(lambda l: l is not None, [self.convert(l) for l in self.pattern])))
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
3 Answers

diogorferreira
19,363 PointsI completely agree, I wish the errors were a little more specific instead of "Bummer!" - I'm not really sure why your code doesn't pass, I've tried it on both VSCode and the challenge and it passes perfectly for me. Maybe just an indentation error? Try pasting it back in to the challenge.
This is just a simplified version of your code if you want to try I just removed the filter and the list as a list comprehension returns the list anyways - it should pass aswell.
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def convert(self, c):
if c is '.': return 'dot'
elif c is '_': return 'dash'
def __str__(self):
return '-'.join([self.convert(l) for l in self.pattern])
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
Hope it helps.
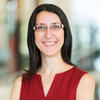
Louise St. Germain
19,424 PointsHi Michael,
The issue is that the code that is original to the challenge is indented with spaces, and the code that you added to it is indented with tabs. I tried converting all your tabs to spaces in your original code, then copied that into the challenge (did not change any of the actual code), and it passed!
Python is really sensitive to the tabs/spaces thing (especially in the challenges, where part of the code is already started), so that's something to watch out for. You might want to consider setting up your code editor so that tabs are automatically converted to spaces for Python, to avoid these kinds of headaches! :-)
Hope this helps!

Strange Learning
Treehouse Guest TeacherThank you all! Got it working doing the old copypasta.
Strange Learning
Treehouse Guest TeacherStrange Learning
Treehouse Guest TeacherWait, wait, wait, so I don't need to filer the Nones? Darn! I thought I did...
Or does this code make the assumptions that we're not going to have any 'naughty' cases, ie: 'dot-dot-foo-bar'?