Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial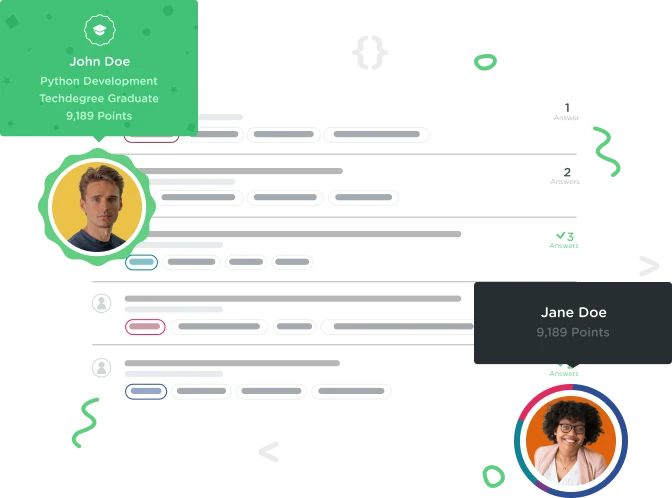
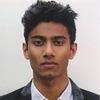
Samuel Kleos
Front End Web Development Techdegree Student 12,780 PointsI'm quite confused. Does this 'mouseover' event 'bubble up' or is it 'captured' from the div?
This tutorial episode provides the following code to add event-handling functionality to all list items within a div
. It does this by adding a mouseover
event listener to the div
containing a ul
with the list items:
const taskList = document.querySelector('.list-container ul');
taskList.addEventListener('mouseover', (event) => {
if(event.target.tagName === 'LI'){
event.target.textContent = event.target.textContent.toUpperCase();
}
})
Does this event 'bubble up' or is it captured?
It seems to me that if you've listened for events on the whole ul element and then transformed a single HTML element (<li>) within it, you'll have to 'capture' an event that has occurred on a single list item rather than the whole div, before then transforming the list item.
I'm failing to understand why is it that if the event bubbles up, the callback function is selectively applied to the <li> element when it is the <li> event that needs to be selected for.
Am I correct in assuming that the event.target.tagName
segments the actual events that 'bubble' up and is not just a way to select the list item objects you want to transform in the DOM?
1 Answer

Nick Hettinger
6,469 PointsThe event bubbles up. The event passes from the child element (LI) and bubbles up to the parent (UL) which has the event listener attached to it. Instead of the LI element having the event listener, it has been delegated to the parent. Your confusion seems to come from the concept of delegation, which enables you to have a parent element listen for an event instead of its child. Bubbling enables delegation. My understanding comes from this article:
https://www.freecodecamp.org/news/event-delegation-javascript/
As for your other question, because of delegation the event listening happens at the parent level. This is where your confusion comes from. The listening happens at the parent level but within the event handler the event object is referring to the child level because that is the actual event target. It is the event object that is responsible for referring to the specific LI that a user mouses over. By my understanding of your question, you have it backwards. In your words, "event.target.TagName
... is just a way to select the list item objects you want to transform in the DOM". The event bubbles up to the parent even without that code being present. The event object exists regardless if you call it into the event handler. Any "segmenting", as in picking out a specific LI element, happens within the event handler when the event has already bubbled up to the parent. I hope that I didn't misunderstand your question.
Rich Donnellan
Treehouse Moderator 27,696 PointsRich Donnellan
Treehouse Moderator 27,696 PointsQuestion updated with code formatting. Check out the Markdown Cheatsheet below the Add an Answer submission for syntax examples, or choose Edit Question from the three dots next to Add Comment to see how I improved the readability.