Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial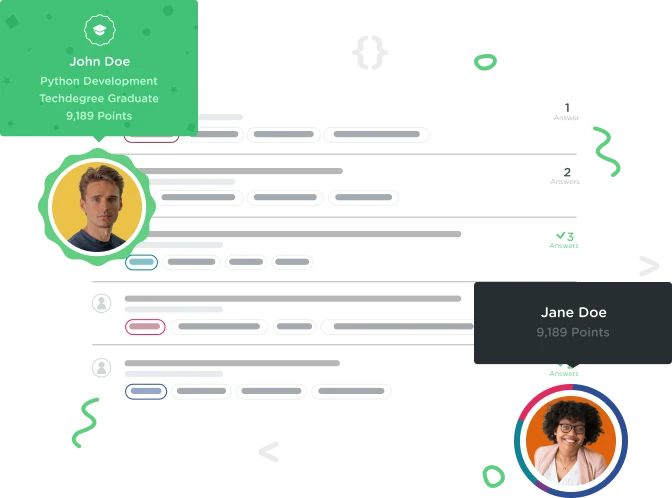

Matthew Thompson
3,554 PointsI'm really close to this working, but can't seem to get the return portion correct
I've used the python terminal to test the code up to the return portion and they all seem to be working with the exception of the return. Any help would be appreciated. Thanks!
import random
pass_num = (10)
def random_num(pass_num):
new_number = random.randint(1, random_num)
return
2 Answers
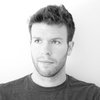
Matthew Rigdon
8,223 PointsI'll show you the correct code first, and then explain why it works:
import random
pass_num = 10 # don't need the parenthesis
def random_num(number):
new_number = random.randint(1, number)
return new_number
random_num(pass_num) # this actually puts your pass_num variable into the function you defined
Now I will explain a few things. Your function was defined correctly, but it is a good habit to name the input value something other than a variable that already exists. I used number (instead of your variable pass_num). This is to avoid thinking that pass_num is the only value that is meant to be placed inside your function. You could place anything in the function that you would like.
Next, remember that in this case, your function needs to return a value. Key word - your function. Make sure you have the return command written inside of your function. You also need to tell it what to return, which is the new variable you created new_number.
Last part. Just defining a function doesn't do anything unless you use it. To use the function, you must call it (the last line I wrote) and you want to send pass_num into the function.
NOTE: 'pass_num' will automatically take the place of 'number' inside the function. 'number' is kind of like a placeholder that waits to have a real value input, and once it does, it will change update all 'number' variables inside the function automatically.

Tatiana Jamison
6,188 PointsTwo things:
You're not currently using the parameter that you pass to the function (pass_num). Did you mean to use that instead of random_num in the call to randint?
Return will return the value following its call. So right now you're instructing the program to return nothing, which is what it does. Try this instead:
return new_number
You can also simplify a bit by putting the expression to be evaluated directly in the return statement.

Matthew Thompson
3,554 PointsI'm trying to get the random_num to use the number 10 as the argument. I think I might be thinking about the process backwards. Ideally I want to return a random number between 1 and 10.
import random
pass_num = (10)
def random_num(new_number): new_number = random.randint(1, random_num) return new_number

Matthew Thompson
3,554 PointsThank you for offering to help! I really appreciate your work.
Matthew Thompson
3,554 PointsMatthew Thompson
3,554 PointsThank you so much for taking the time to offer such a thoughtful response. I appreciate your help.