Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial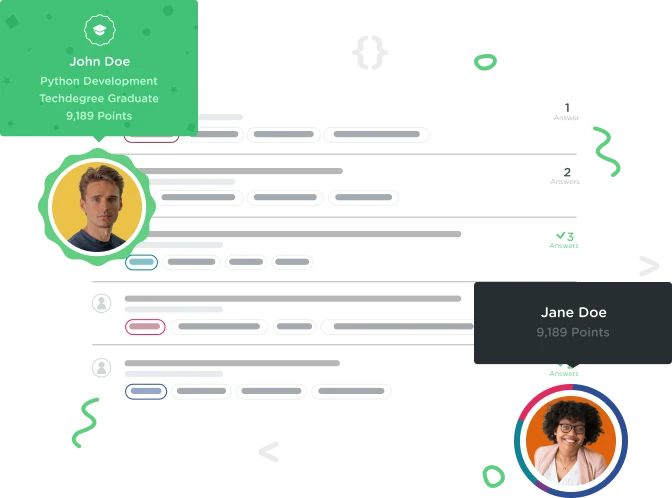

George Columbu
2,076 PointsI'm really confused about the function in this code
Hello, I'm confused why do i need a function to do something when the button is clicked instead of just telling it what to do with the code inside the function directly. And why doesn't the function have any name in the first place? I mean what is the point of this function right here, if he doesn't even call it. Thank you.
2 Answers
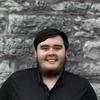
Michael Hulet
47,913 PointsGuil may not call that function directly, but JavaScript calls that function whenever the button is clicked. It doesn't have a name because it doesn't need one, but it's perfectly alright if you want to give it one, too
The addEventListener
method takes 2 arguments:
- The event to listen for
- A function that JavaScript runs when the event happens
So, in total, this snippet:
myButton.addEventListener("click", () => {
myHeading.style.color = myInputText.value;
});
adds an event listener on myButton
that will call the anonymous function passed as the 2nd argument whenever the button is clicked. That function will set myHeading
's color
to be the value
of myInputText
.
You can also name this function, if you want to. This snippet is still totally valid and does the same thing:
const clickHandler = () => {
myHeading.style.color = myInputText.value;
};
myButton.addEventListener("click", clickHandler);
The only difference between the 2 snippets I've posted so far is that the function that's called whenever myButton
is clicked is now named clickHandler
Now, let's look at the case where we don't use a function for the click handler. This is what I think you're visualizing:
myButton.addEventListener("click");
myHeading.style.color = myInputText.value;
Now, if we run this code, 2 things will happen as soon as the page loads:
- JavaScript will throw a
TypeError
because not enough arguments were passed toaddEventListener
-
myHeading
'scolor
will be set to an empty string
Notably, this will also cause nothing to happen whenever the button is clicked. That's because when code is in the global scope like this, JavaScript will read the file from top to bottom and execute every command immediately, as soon as the file is loaded. Putting this line:
myHeading.style.color = myInputText.value;
into a function and passing that function to addEventListener
captures that line of code and tells JavaScript not to run it immediately, but to hang onto it and run it whenever the button is clicked. That's why addEventListener
takes 2 arguments. It needs to know:
- What event to listen for
- What code to run whenever that event happens The way you do that is by telling it the event to listen for as a string, and then passing the code you want to run whenever that event happens as a function that it can call when the event happens

George Columbu
2,076 Pointsthank you sir, this was a perfect answer for me