Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial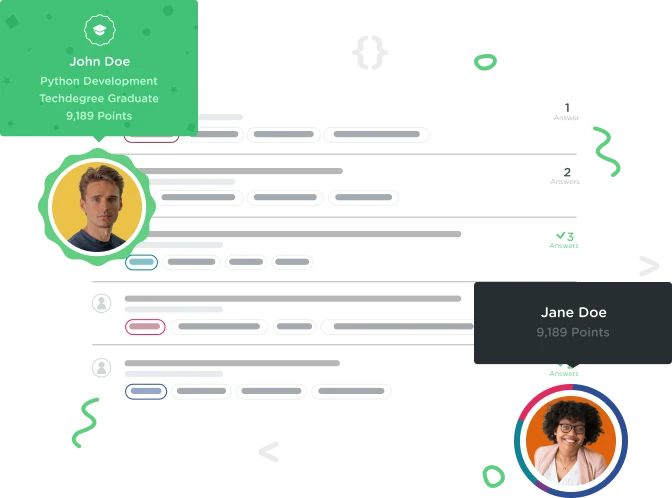

Enemuo Felix
1,895 PointsI'm really desperate to understand this code
function printList( list ) {
var listHTML = '<ol>';
for (var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML); //the listHTML is written to the document with the print function. The print function is declared below.
}
function print(html) {
document.write(html);
}
Actually, I copied the above code somewhere here , and i will like to understand it more than anything. Someone here have explained it for me before but i couldn't get but gave up , only to come across it again.
My question is, how did the call of the print
function run the whole code. I mean i can't see the connection with the previous codes above it? And why is html used as the argument, Is it not suppose to be listHTML? Katie Wood . Anyone?
2 Answers
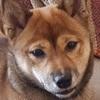
Katie Wood
19,141 PointsHi there,
One important thing to remember is that the argument of a function and the variable passed in do not have to have the same name. You're right that listHTML is the name of the variable, but it is passed to the function on the 7th line of the code you have there. In the print function, the parameter is basically declaring a new variable using whatever name is in the parentheses - the value will be set to whatever is passed in, and will persist until the function finishes. In some programs the name is the same just to make it easier to follow, but it doesn't need to be.
In this case, calling print() wouldn't run the whole code - all it does is print whatever is passed in, which in this case is the contents of the listHTML variable.
When a script is run, it will automatically run any code in the file, unless it is inside a function or a class (something that must be called or instantiated). Any other code will just run sequentially.
In this video, playlist.js is what runs when you run the application - it declares the playlist, adds items to it, then calls printList() using the playlist array. printList() then calls print(). So you start here:
//playlist.js
var playlist = [];
playlist.push('I Did It My Way');
playlist.push('Respect', 'Imagine');
playlist.unshift('Born to Run');
playlist.unshift('Louie Louie', 'Maybellene');
printList( playlist ); //when we get here, it calls printList in the other file
then we go here:
//helper.js
function printList( list ) {
var listHTML = '<ol>';
for (var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML); //calls print
}
and finally:
function print(html) {
document.write(html);
}
It's worth noting that helper.js is only there to separate the functions for organizational purposes - you could technically have all of it in one file if you wanted. It's just important to know how to separate the code because it becomes really important when managing larger projects.
Hope this helps!

markmneimneh
14,132 PointsHello
I think the code you listed does not show the whole picture. Please try the following ... it should give you an idea. There is no magic here.
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<button type="submit" id="myButton"></button>
<script src="app.js"></script>
</body>
</html>
app.js
function printList( list ) {
var listHTML = '<ol>';
for (var i = 0; i < list.length; i += 1) {
listHTML += '<li>' + list[i] + '</li>';
}
listHTML += '</ol>';
print(listHTML); //the listHTML is written to the document with the print function. The print function is declared below.
}
function print(html) {
document.write(html);
}
const list = ['Mark', 'Jim', 'jimmy']
document.getElementById('myButton').addEventListener('click', printList(list))
I hope this answers your question. If so, please mark the question as answered.
Thanks
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThank you Katie for showing up. So if I got you correctly , the function of the code was only called before the declaration. By joining the first two code you posted (playlist.js and helper.js), So this code below are the same (declaring before calling)?
Katie Wood
19,141 PointsKatie Wood
19,141 PointsLooks like you have the right idea - if we add just a little bit to the code you have there, you'd have the full functionality of the two files:
You're right in saying that, on the helper.js file, print() was called higher up in the file than it was declared - when a function actually runs depends on when it is called, rather than where it is in the file.
Enemuo Felix
1,895 PointsEnemuo Felix
1,895 PointsThanks, I got It!
Katie Wood
19,141 PointsKatie Wood
19,141 PointsGreat!