Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial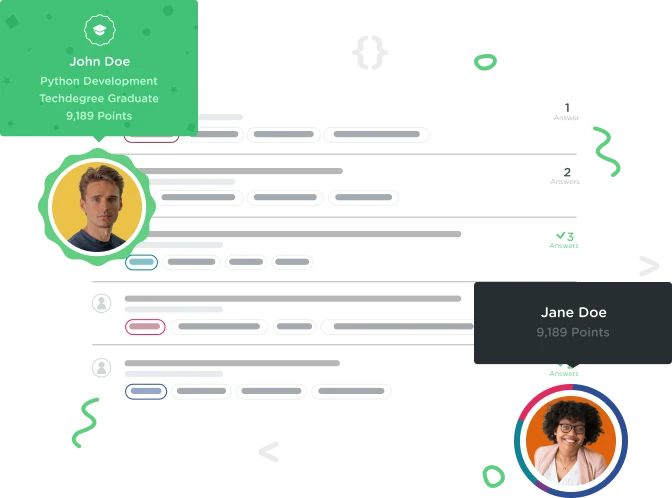

Chris Loach
4,024 PointsI'm really struggling to get to grips with what I'm doing wrong.. Please help!
I'm struggling with replacing the plant type & status using the double curly brackets..
With all the plants passed into the index.html template, it's time to put them to use. Inside of index.html there is a <div> tag with the plant type and status inside of it. Wrap this div inside of a Flask for loop. {% for plant in plants %} before the div and then {% endfor %} after it. Replace plant type and status with the info from the database using double curly brackets.
from models import db, Plant, app
from flask import render_template, url_for, request, redirect
@app.route('/')
def index():
plants = Plant.query.all()
return render_template('index.html', plants=plants)
@app.route('/form', methods=['GET', 'POST'])
def form():
if request.form:
new_plant = Plant(plant_type=request.form['type'],
plant_status=request.form['status'])
db.add(new_plant)
db.commit()
return redirect(url_for('index'))
return render_template('form.html')
@app.route('/delete')
def delete():
return redirect(url_for('index'))
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8000)
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///plants.db'
db = SQLAlchemy(app)
class Plant(db.Model):
id = db.Column(db.Integer, primary_key=True)
plant_type = db.Column(db.String())
plant_status = db.Column(db.String())
{% extends 'layout.html' %}
{% block content %}
<form action="{{ url_form('form') }}" method="POST">
<label for="type">Plant Type:</label>
<input type="text" name="type" id="type" placeholder="Plant type">
<p>Plant Status:</p>
<label for="good">GOOD</label>
<input type="radio" name="status" id="good">
<label for="ok">OK</label>
<input type="radio" name="status" id="ok">
<label for="bad">BAD</label>
<input type="radio" name="status" id="bad">
</form>
{% endblock %}
{% extends 'layout.html' %}
{% block content %}
<h1>Our Plants:</h1>
{% for plant in plants %}
<div>
<h2>{{ plant_type }}</h2>
<p>{{ plant_status }}</p>
</div>
{% endfor %}
{% endblock %}
1 Answer
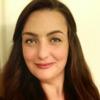
Jennifer Nordell
Treehouse TeacherHi there, Chris Loach ! You are super close. You're sending in plants
which is the result of all plants. Each plant has three fields: an id, a plant_type, and a plant_status. Remember that these are part of the plant
. So instead of just plant_type
and plant_status
you would need plant.plant_type
and plant.plant_status
.
Hope this helps!