Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial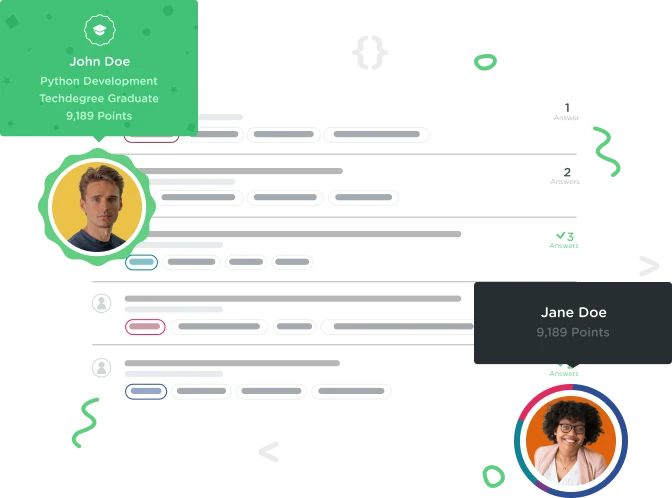

Camille C
5,158 PointsI'm ridiculously stuck and I can't even explain. I can't do the for loop right or check statement right. Please help.
Can't figure it out. I have been through the video about 3 times.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
private int counter;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter(char letter) {
for (char tile : tiles.toCharArray()) {
counter = 0;
if (hasTile(tile) == hasTile(letter)) {
counter++;
}
}
return counter;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers

Thomas Nilsen
14,957 PointsAh - I forgot to declare "counter".
Also, we shouldn't declare the counter inside the loop. That will reset it every time the loop runs
the code should look like this
public int getCountOfLetter(char letter) {
int counter = 0;
for (char tile : tiles.toCharArray()) {
if (tile == letter) {
counter++;
}
}
return counter;
}

Thomas Nilsen
14,957 PointsYou're overthinking this;
Why use hasTile in your if-statement?
This is enough:
public int getCountOfLetter(char letter) {
for (char tile : tiles.toCharArray()) {
counter = 0;
if (tile == letter) {
counter++;
}
}
return counter;
}

Camille C
5,158 PointsWow. Thank you. I wish I would have uploaded a shot of when I tried it this way as well so I could see where I had went wrong, but I am truly grateful for your help. Thanks again :) .
Actually I think there is a bug somewhere. It said well done (like it had before but I thought it may have been because I was on my phone) and then took me back to resubmit the answer and now says "Bummer! Hmmm, the tiles were "sreklahc" and I passed in 'r' expected 1, but I got 0". I've sent a request for possible bug fix issue.
Camille C
5,158 PointsCamille C
5,158 PointsOmg yes! The only thing I did different was I initialized it as "private int counter;" within the class and then set it to "0" outside of the for loop. Beautiful. Onward!