Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial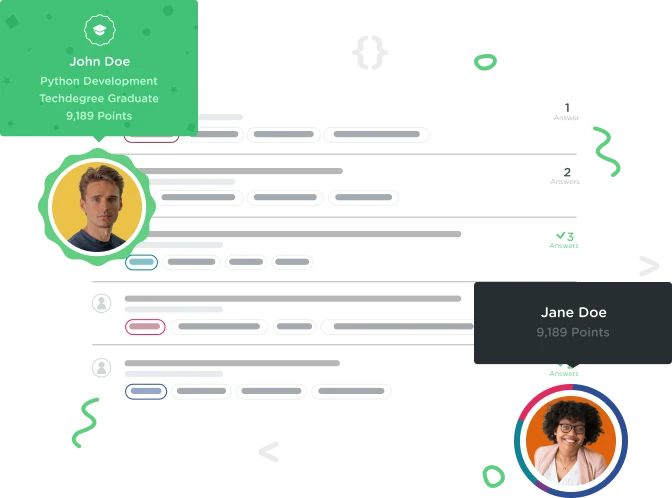

Tim Arwine
5,406 PointsI'm running workspaces code (Java Basics) in Intelli J, but getting nothing but errors. What am I missing?
I've installed Intelli J. It's working. But not with the code from our earlier course - Java Basics. I must be missing something simple. Please help.

Tim Arwine
5,406 PointsJeremy. Yes. Could you show me how to use the scanner object instead. Thank you!

anil rahman
7,786 PointsI showed you in my answer already, explaining everything.

Tim Arwine
5,406 PointsThank you both (anil and Jeremy). The first part with the System.out.printf worked like a charm! Wonderful. I'm still confused on the second part. I am not sure where how (and where) to integrate the Scan object code you gave me into my own. I'm working on the video/lesson that deals with readLine. Here's the code I'm starting with for reference: import java.io.Console; public class Introductions {
public static void main(String[] args) {
Console console = System.console();
// Welcome to the Introductions program! Your code goes below here
String firstName = console.readLine("What is your name? ");
System.out.printf("Hi, My name is %s. I'm a programmer.\n", firstName);
System.out.printf("%s is learning to program in Java. Yippeee!\n", firstName);
} }
10 Answers
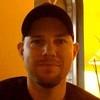
Jeremy Hill
29,567 Pointsimport java.util.Scanner;
public static void main(String[] args) {
//Console console = System.console();
Scanner input = new Scanner(System.in);
// Welcome to the Introductions program! Your code goes below here
//String firstName = console.readLine("What is your name? ");
System.out.println("What is your name?");
String firstName = input.nextLine();
System.out.printf("Hi, My name is %s. I'm a programmer.\n", firstName);
System.out.printf("%s is learning to program in Java. Yippeee!\n", firstName);

anil rahman
7,786 PointsYes Jeremy is correct when using the console object in ide's it usually brings back a nullPointerException.
So when using the printf method using console like this:
console.printf("name is %s",name);
Switch it to:
System.out.printf("name is %s",name);
To read data from user input use the Scanner object.
Scanner s = new Scanner(System.in); //the scanner object
String input = s.nextLine(); //read data entered and save in a variable
System.out.printf("You typed %s",input); //output to screen
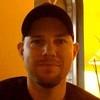
Jeremy Hill
29,567 PointsThe code I last wrote will work in your IDE, but not the code challenges, FYI.

Tim Arwine
5,406 PointsJeremy, Thank you so much. Your code worked perfectly. Brilliant. I'm off and running! (And yes. For the challenges, I'll have to use the old code.)

anil rahman
7,786 Pointsimport java.util.Scanner;
public class MyStory {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
System.out.println("Enter your name: "); //i have split these lines into two, prompt first and get input after
String name = input.nextLine();
System.out.println("Enter an adjective: ");
String adjective = input.nextLine();
System.out.printf("%s is very %s", name, adjective);
}
}
before you used this:
String name = System.out.println("Enter your name: "); //prompting and getting value
//but you can't do this with scanner
//i have split these lines into two,
System.out.println("Enter your name: "); //prompt first
String name = input.nextLine(); //and get input after

Tim Arwine
5,406 Pointsanil, THANK YOU SO MUCH. It works. I see. Your explanation really clarifies it. I was so confused. Anyway, bottom line. Different objects have VERY different behaviors. Thank you again.
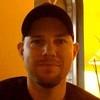
Jeremy Hill
29,567 PointsOkay first import your Scanner object like this:
import java.util.Scanner;
Then create a Scanner object:
Scanner userInput = new Scanner(System.in);
Now you can use the object in conjunction with System.out to print to the console like this:
String word = userInput.nextLine();
System.out.println("The word or line stored in the variable is: " + word);
// or
System.out.printf("The word or line stored in the variable is: %s ", word);
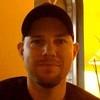
Jeremy Hill
29,567 PointsAnd you can even use .next() instead of .nextLine() if you prefer, depending on what the user is inputting.
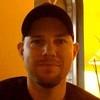
Jeremy Hill
29,567 Pointsanil had the code for it, just make sure that you import the Scanner class.

anil rahman
7,786 Pointsi didn't bother adding the import because when using intellij the first thing it does is say i'll import all needed statements for you if you are missing it. No one will try add them in manually when using these types of ide's.
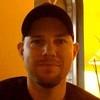
Jeremy Hill
29,567 PointsGood deal! If you have any more problems or questions let me know :)

Tim Arwine
5,406 PointsI definitely will. Thank you!
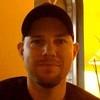
Jeremy Hill
29,567 PointsI just thought of something else that you might like to use in your ide.
Run this code in your ide:
import javax.swing.JOptionPane;
public class Example {
public static void main(String[] args) {
String firstName = JOptionPane.showInputDialog(null, "What is your first name?", "First Name",JOptionPane.PLAIN_MESSAGE);
String lastName = JOptionPane.showInputDialog(null, "What is your last name?", "Last Name",JOptionPane.PLAIN_MESSAGE);
JOptionPane.showMessageDialog(null, "Your name is: "+firstName+" "+lastName, "Your Name",JOptionPane.PLAIN_MESSAGE);
}
}

Tim Arwine
5,406 PointsJeremy,
Thank you very much. What or where exactly should I use this? Is this what we use instead of the readline method (to gather imput from user)?
Because, once again, I am having trouble getting the code in the Java Basics examples to work in the ide. I'm on the section on user input:
Here's what I have and I'm getting multiple errors galore. Please help.
import java.util.Scanner;
public class MyStory {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
/* Some terms:
noun - Person, place or thing
verb - An action
adjective - A description used to modify or describe a noun
Enter your amazing code here!
*/
String name = System.out.println("Enter your name: ");
String adjective = System.out.println("Enter an adjective: ");
System.out.printf("%s is very %s", name, adjective);
}
}
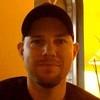
Jeremy Hill
29,567 PointsUse anil's example, just remember that when using a scanner object that when you are getting input from the user you must use a System.out.println() statement to prompt the user for input before the line that stores the input. The Console.readLine() allows you to prompt the user and get input in one line but the Scanner object doesn't work like that- It requires two lines of code.

Tim Arwine
5,406 PointsJeremy, THANK YOU. Both you guys really helped me. I never would have thought one (is it method or object?) behaves so different to another, but it is a great lesson for the future. Thank you again! I really appreciate you guys willingness to help me over the humps.
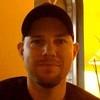
Jeremy Hill
29,567 PointsNo problem Tim, glad to help :)
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsIt may be the Console object. I never have luck with that in my own ide. If you are using the Console object try using the Scanner object in replace of that. I can show you if you have never used it before- I think it works better anyway.