Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial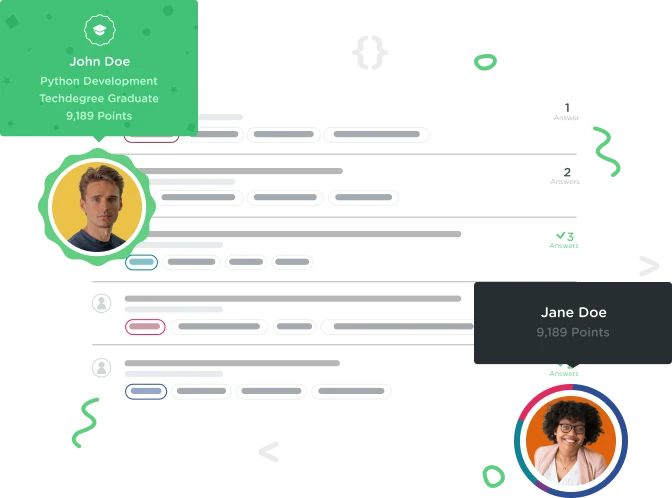
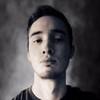
Brandon Evans
8,154 PointsI'm simply stuck on this challenge and am not sure why I'm getting a SyntaxError What's the correct answer?
I can't figure out why I'm getting a parse error with my code and just want to know what the correct answer is:
var secret = prompt("What is the secret password?");
do ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
} while ( secret === "sesame" ) {
document.write("You know the secret password. Welcome.");
}
var secret = prompt("What is the secret password?");
do ( secret !== "sesame" ) {
secret = prompt("What is the secret password?");
} while ( secret === "sesame" ) {
document.write("You know the secret password. Welcome.");
}
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>JavaScript Loops</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
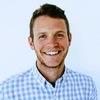
Nick Hericks
Full Stack JavaScript Techdegree Graduate 20,704 PointsYou're so close! Here are a few things that I noticed:
1) Remember that when using a do...while loop, it can only have one condition, and it needs to be placed after the 'while' part of the loop. The syntax looks like this:
do
statement (code to run)
while (condition);
2) Note that after the condition, there are no more code statements as part of the do...while loop, so it should end with a semicolon. Any code statements written in your file that are below the do...while loop will only run after the condition of the loop has been met, so you can list the document.write() method as a separate code statement below the loop.
3) Also, you only need to call the prompt() method once, and it should be located inside the loop. So you should declare the secret variable before the loop, but not assign it a value until inside the loop.
So to bring it all together it should look something like this:
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
More on do...while loops at the MDN site here.
I remember being confused by these same things not too long ago, and it can feel like there are so many little things to learn about each individual piece of JavaScript, but after you use them a few times, they stick and it gets much easier.
Hope that's helpful! Keep on coding!

Alfonso Montano
2,970 Pointsdo...while loops are confusing.
do
// statement
while (condition);
var secret;
do {
secret = prompt("What is the secret password?");
} while ( secret !== "sesame" );
document.write("You know the secret password. Welcome.");
This code basically says: Prompt the user for the secret password then check if the secret password is correct. If password is not correct ask again.
If you're interested in learning more about do...while loops MDN is a great resource. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/do...while
Brandon Evans
8,154 PointsBrandon Evans
8,154 PointsWow, thank you so much! I was pretty far off lol. The do/while loops really seem to have a hard time sticking with me. I really appreciate the help!