Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial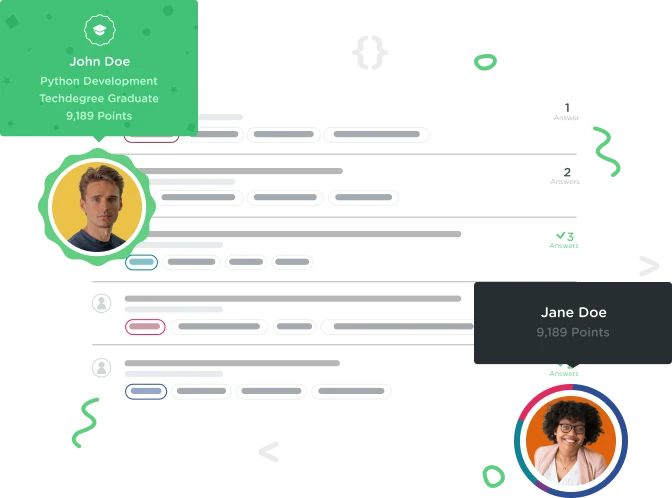

sabath rodriguez
11,055 Pointsim so lost, you guys should post how to videos on how to solve it after X amount of tries. I want to know what to do.
func removeVowels(value: String) -> String {
let valueLowerCased = value.lowercased()
var tempString = ""
for array in valueLowerCased.characters {
if array == ( "a" || "e" || "i" || "o" || "u" ) == true {
tempString.append(array)
}
}
return tempString
}
// Enter your code below
extension String {
func transform(_ value: (String) -> String) -> String {
return value(self)
}
func removeVowels(from value: String) -> String {
let valueLowerCased = value.lowerCased()
var tempArray: [String] = []()
for array in valueLowerCased.characters {
if array == ()
}
}
}
1 Answer

Shade Wilson
9,002 PointsHey Sabath,
This is one of the harder challenges in my opinion, so don't feel bad. You're actually closer than you think! Also, Google is your friend,. It certainly helped me get through this challenge again just now so I could help you with your problem.
It seems like you were able to get part 1. Part 2 is where is gets tricky. Your if statement has two problems: there's two equality checks (==), one after array
and one before true
. You should only ever have one: it will either return true or false, so you wouldn't need to check again even if Swift let you. Second, or statements in Swift do not work like how you wrote yours:
if array == ( "a" || "e" || "i" || "o" || "u" ) // Don't do this!!
How you have to write this is:
if array == "a" || array == "e" || array == "i" // etc...
As you can imagine, writing a statement like this is horribly inefficient for stringing together multiple or statements. Instead, what you should do it take all the cases you want to check for (all the vowels) and put them in an array to themselves:
let vowels: [Character] = ["a", "e", "i", "o", "u"]
Now you want to use a for in
loop like you tried to cycle through value.characters
(you don't have to change the letters to lower case for this challenge, and you probably don't want to). Within this loop, check to see if each character is in the vowels
array with:
if vowels.contains(character) // this returns a Bool, make sure you select whether you want true or false!
And you're absolutely right about wanting to append the characters that aren't vowels to a new array, but it'll have to be a Character array to work with other character arrays. You'll then have to convert your array to a string using String()
. Once you return that you should be good to go! I should mention that this method isn't the only way this could've been done. Pretty much all problems you run into while coding can be approached in many different ways, so keep that in mind.
Gabbie Metheny
33,778 PointsGabbie Metheny
33,778 PointsTreehouse doesn't explicitly tell you what to do if you can't get it, but people are really good about helping out in the forum! I don't know Swift, but I bet someone will show up soon who can help you out.
In the meantime, you can click on Higher Order Functions here or at the top of your post and you'll get a page with everyone who's had problems on the same code challenge. Should be some good stuff in there!