Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial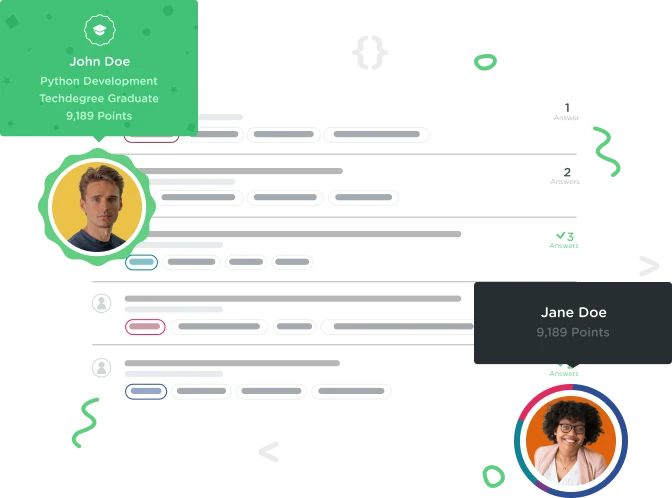
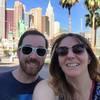
Thomas McNish
10,893 PointsI'm still confused about initializers and classes
I think I understand Structs fairly well (I'm still a beginner), and I understand the concept of Classes, but I'm confused about the syntax and I'm particularly confused about initializers. It's getting muddy for me when I try to figure out passing in arguments and creating instances. Here's the problem I'm stuck on.
In the editor you've been provided with a struct named Location that models a coordinate point using longitude and latitude values.
For this task we want to create a class named Business. The class contains two constant stored properties: name of type String and location of type Location.
In the initializer method pass in a name and an instance of Location to set up the instance of Business. Using this initializer, create an instance and assign it to a constant named someBusiness.
I've gotten some of the basic code in, but I don't know where to go from here. Can anyone help me better understand initializers and methods? Here's what I've gotten so far.
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = Location(latitude: 33.9, longitude: 49.8)
}
}
Thank you!
3 Answers
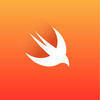
Steven Deutsch
21,046 PointsHey Thomas McNish,
The purpose of an initializer is to prepare an object for use. In order for an object to be ready for use, all of its properties must have values. When we call the initializer method, we pass it the specific arguments that we have specified as its parameters in the initializer declaration. These arguments are used to assign values to the properties of that object.
The problem with your code is this:
You are passing an instance of location as the second argument of the initializer, however, you never use the argument that you pass in. Instead, you are creating a separate instance unrelated to the argument you passed in and are assigning it to the location property.
class Business {
let name: String
let location: Location
// Notice how you use name but location never gets used in the body
init(name: String, location: Location) {
self.name = name
// this is where you create a new instance instead of using location
self.location = Location(latitude: 33.9, longitude: 49.8)
}
}
What you want to do is this:
struct Location {
let latitude: Double
let longitude: Double
}
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location
}
}
// Now when we call this initializer, we pass in an instance of Location as the 2nd argument
// Here I create the instance of Location directly inside the initializer call
let someBusiness = Business(name: "AnyString", location: Location(latitude: 20.0, longitude: 20.0))
Hope this clears a few things up, Good Luck!
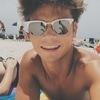
garyguermantokman
21,097 PointsThe initializer in the Business class is close.
class Business {
let name: String
let location: Location
init(name: String, location: Location) {
self.name = name
self.location = location // pass in the paramater in the init function
}
}
Think of the init() method as a way to give stored properties (without default values) values. Later when you "Using this initializer, create an instance and assign it to a constant named someBusiness."
// Create a new constant to hold an instance of Business
let someBusiness = Business(name: "Apple", location: Location(latitude: 4332.0, longitude: -3243.0))
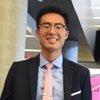
Paul Je
4,435 PointsThe object is the class, or struct; for now just know that it's the entity that you're referring to which you can prepare the properties (var or constants) for battle (quite literally) via initializing the properties and naming them (or equating) to the initialized values that you'd want to pass in your end values into. Think of it as an output based on the type of the properties from the properties themselves. Hope this made sense... Good luck!!!
Thomas McNish
10,893 PointsThomas McNish
10,893 PointsThanks Steven, that was very helpful, and I think I understand what you're saying. However, I do have one more question that could use some clarification. There's a line in the instructions that says "In the initializer method pass in a name and an instance of Location to set up the instance of Business."
I thought that we created an instance of Business outside of the class, when we assigned it to the constant someBusiness. How do you pass in an instance (of Location in this example) in an initializer method?
EDIT: Steven Deutsch thank you so much! That was very helpful. I feel a lot more confident about these concepts now. I really appreciate you breaking it down for me like that. I was definitely hitting a wall, and I now feel like I can move forward.
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsFirst off, I had to correct the typo. For some reason I was missing the Business type name before the call to my initializer.
If we take a look at the initializer method for Business, it looks like this:
This means when we call the initializer (like any other function), we have to pass it the values for its parameters.
This means we will be passing it two arguments, a string for the name, and an instance of the Location type for the location.
I could have done it this way as well:
Hope this helps.
Steven Deutsch
21,046 PointsSteven Deutsch
21,046 PointsNo problem Thomas McNish it's all about breaking down walls. You will face them countless times when learning development. Every week I still face a few that make it seem like it's almost impossible to continue, but eventually things work out.