Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial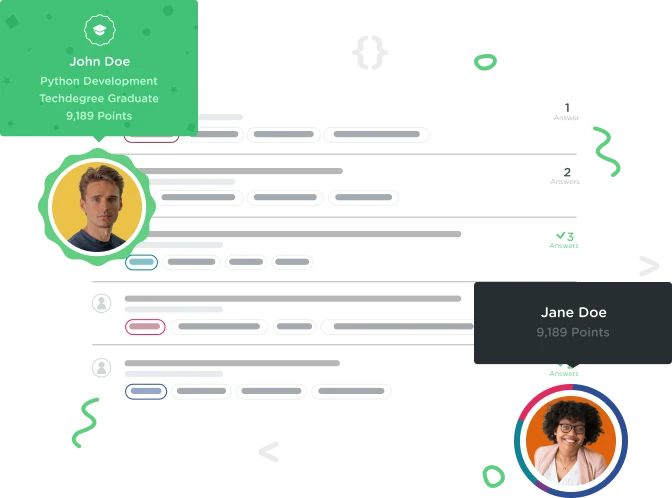

Carolina Estrada
1,285 PointsI'm still confused on what toString() does
When you add an object to return under the toString() method this overrides what exactly? and Where?
2 Answers

Lauren Moineau
9,483 PointsHi Carolina. The toString() method is part of the Object Class. As every class is a subclass of the Object class, any class inherits that method.
What does it do? Its aim is to give a string representation of an object (an instance of that class). The original toString() method returns:
getClass().getName() + '@' + Integer.toHexString(hashCode());
For example:
com.company.Dog@4554617c
where
- com.company is the package
- Dog is the class
- 4554617c is the hash code converted into hex String. The hash code is an Integer representation of the object, that is unique to that object (You could actually use it to compare 2 objects and check whether they're equal or not).
As you can see, com.company.Dog@4554617c is not very readable. That's why it's recommended we override ("re-write") that original toString() method when we create a new class so we can make it return something more readable, as Craig does in the video:
@Override
public String toString() {
return "Treet: \"" + mDescription + "\" - @" + mAuthor;
}
So that calling treet.toString() now returns: Treet: "Want to be famous? Simply tweet about Java and use the hashtag #treet. I'll use your tweet in a new Treehouse course about data structures." - @craigdennis. We now have a more human-readable representation of that treet object.
Hope that helps :)

Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsNow every class in Java is a child of the Object class; Object contains toString() method which Java classes automatically inherit.
The main purpose of toString() method is to generate a String or text representation of a Java Class; in other words to provide meaningful and informative details that a person can read and understand about that Java class.
Whenever you access an Object in a String context (e.g. when using printf, println) the toString() method is invoked from under the covers to print out Object in textual format.
Consider the below code with me:
We declare a Class Girl with name, height and diet fields; we initialize these fields with values on the Girl's constructor. Inside the Girl Class, we override toString() method and set what the desired output should be when the Girl Class happens to be called in a String context.
Inside the main method we try to print out the Girl class using System.out.println()
We get this result thanks to us overriding toStringMethod() inside the Girl class
My name is carolina, I am 6 feet tall and I am a vegetarian
public class Main {
public static void main(String[] args) {
// creating a new instance of a Girl Class
Girl carolina = new Girl();
//printing out the Girl Class in text
System.out.println(carolina);
//Output will be "My name is carolina, I am 6 feet tall and I am a vegetarian"
}
//declaring a new nested class called Girl
public static class Girl {
private String name;
private int height;
private String diet;
//initialize the above field with random values
public Girl() {
name = "carolina";
height = 6;
diet = "vegetarian";
}
//Override toString() to set the desired text output of our Girl class
@Override
public String toString() {
return ("My name is " + name + ", I am " + height + " feet tall " + "and I am a " + diet);
}
}
}
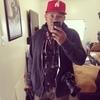
Bryan Martinez
2,227 PointsGreat explanation! Thank you
Tonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsTonnie Fanadez
UX Design Techdegree Graduate 22,796 PointsLauren Moineau thanks for the great answer, you are on point.
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsYou're welcome Tonnie Fanadez :)
Bryan Martinez
2,227 PointsBryan Martinez
2,227 PointsGreat explanation! Thank you for taking the time to explain it.
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsThank you Bryan Martinez :) I'm glad you found it useful.