Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial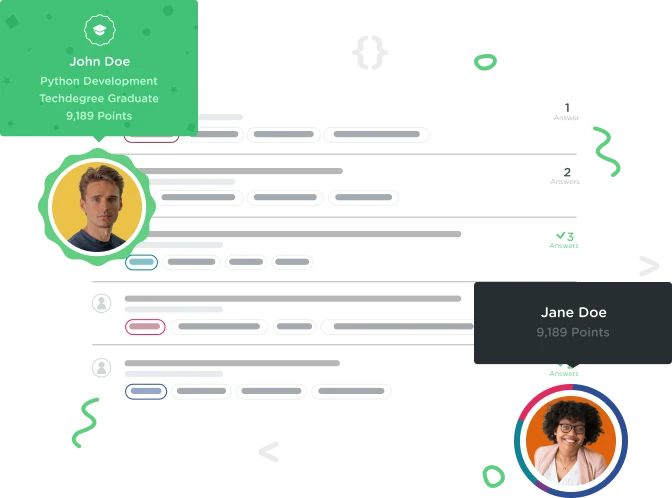

kristoffer tølbøll
1,337 PointsI'm still having trouble understandig the static keyword, what difference does it make to a variable?
I'm still having trouble understandig the static keyword, what difference does it make to a varible? how does it differ from a non-static variable?
3 Answers
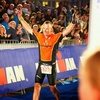
Steve Hunter
57,712 PointsHi Kristoffer,
This is a tricky concept to get your head round, I agree!
The static
keyword, when used on a member variable is associated with the class
not the objects/instances of the class. So, you can use it when no objects exist.
public class Steve{
public static String name = "Steve";
}
Here's a class definition. In your code you can access this variable:
// somehwere in your code
System.out.println(Steve.name);
You call it off the class name, hence the capitalized Steve
.
The variable is common across all instances too. So, you can do this:
public class Steve{
public static String name = "Steve";
public static int howMany = 0;
public Steve(){
// I'm a constructor
howMany++;
}
}
So, here in the constructor, we increment the howMany
variable. This will count how many instances of Steve
exist (one's enough, trust me!). This means every instance of Steve
will contain the same value in howMany
So:
Steve oneSteve = new Steve();
Steve twoSteve = new Steve();
Steve threeSteve = new Steve();
Every instance here will have the value 3 held in howMany
. oneSteve.howMany
and threeSteve.howMany
will both hold 3. This means you can count instances of your class, or how many times the constructor has run, anyway.
I hope that helps!
Steve.
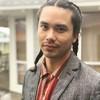
Jay Reyes
Python Web Development Techdegree Student 15,937 PointsGreat answer and humor, Steve!
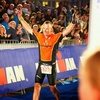
Steve Hunter
57,712 PointsThanks!

Anil kumar N
658 Pointsi cleared my doubt too. Now, thats called a real team :)
Oziel Perez
61,321 PointsOziel Perez
61,321 PointsTo further elaborate on the answer, Say you have oneSteve.property = true, and twoSteve.property = false. Those two variable exist in different parts of memory because they are part of two separate objects. However, "static" means that there is only one reference of the variable, in this case 'howMany'. So calling oneSteve.HowMany, twoSteve.howMany, or access it off the class as Steve.howMany, it will be the same variable store in the same memory reference, which is why you can access this variable without instantiating a class as an object.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsGood input.

kristoffer tølbøll
1,337 Pointskristoffer tølbøll
1,337 Pointsgood answer thanks!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHalisson Alves
14,149 PointsHalisson Alves
14,149 PointsThanks a lot Steve Hunter ... Now it's very clear for me with your explanation!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem, Halisson - glad to help.
Steve.