Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial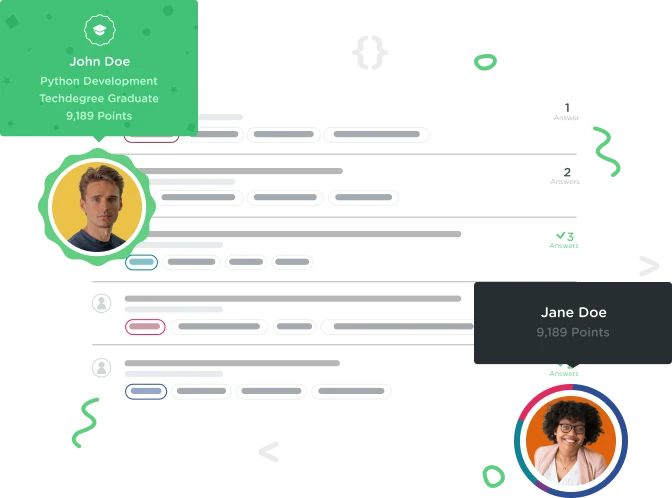

Justin Vinson
713 PointsI'm struggling to solve a NullReferenceException error involving the audioSource variable. Please help!
Watched this video and checked my code several times. Any idea as to what is going wrong?
Here's the exact code loaded as a script under the Bird Calls child of the Bird game object:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RandomSoundPlayer : MonoBehaviour
{
private AudioSource audioSource;
[SerializeField]
private List<AudioClip> soundClips = new List<AudioClip>();
[SerializeField]
private float soundTimerDelay = 3f;
private float soundTimer;
// Use this for initialization
void Start()
{
audioSource.GetComponent<AudioSource>();
}
// Update is called once per frame
void Update()
{
// increment a timer to count up to restarting
soundTimer = soundTimer + Time.deltaTime;
// if the timer reaches the delay
if (soundTimer >= soundTimerDelay)
{
// ... reset the timer...
soundTimer = 0f;
// ... choose a random sound... (soundClips.Count generates maximum for random selection range :)
AudioClip randomSound = soundClips[Random.Range (0, soundClips.Count)];
// ... and play the sound
audioSource.PlayOneShot (randomSound);
}
}
}
And this is the error I receive every three seconds as the script attempts to play the random sound:
NullReferenceException: Object reference not set to an instance of an object RandomSoundPlayer.Update () (at Assets/Scripts/RandomSoundPlayer.cs:40)
the line it references is:
audioSource.PlayOneShot (randomSound);
the tricky thing is at launch I get a Start error as well:
NullReferenceException: Object reference not set to an instance of an object RandomSoundPlayer.Start () (at Assets/Scripts/RandomSoundPlayer.cs:19)
referring to my GetComponent line:
audioSource.GetComponent<AudioSource>();
I assume the problem has something to do with the AudioSource component having a blank AudioClip field but I can't seem to resolve the problem as placing anything in the field does not change the error (also nothing is placed in the field in the video walkthrough).
Any help would be greatly appreciated! Thank you!
1 Answer

Seth Kroger
56,413 PointsSince audioSource
isn't a serialized field it will be uninitialized when you hit the the Start method. Instead of initializing it in Start() you are calling a method from it. Hence the null reference error. You should be initializing it with GetComponent instead:
void Start () {
audioSource = GetComponent<AudioSource> ();
}