Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial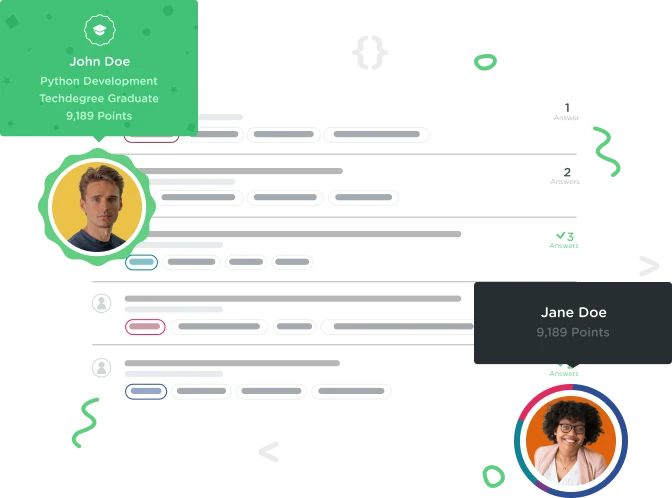

gerardo keys
14,292 PointsI'm struggling to understand some of these things.
A lot of these are new concepts even for the people that have already gone through js/promises/async-await etc. It may be simple but I'm not understanding what this object does:
const db = {
sequelize,
Sequelize,
models: {}
};
Why does it need two sequelize instances? Why is the models object empty and why is it there at all? I don't see this explained much.
db.models.Movie = require("./models/movies")(sequelize);
Why does this have (sequelize) at the end? I've never seen this format before.
const Sequelize = require("sequelize");
module.exports = sequelize => {
class Movie extends Sequelize.Model {}
Movie.init(
{
title: Sequelize.STRING,
description: Sequelize.STRING,
year: Sequelize.NUMBER
},
{ sequelize }
);
return Movie;
};
In the part that shows module.exports = sequelize =>
, again this may be a simple question but where does the lowercase sequelize argument come from as the imported module is Sequelize with a capital S. I see no other imports with lowercase sequelize.
4 Answers
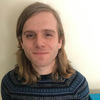
sradms0
Treehouse Project Reviewer1.) Why does it need two sequelize instances?
const db = {
sequelize,
Sequelize,
models: {}
};
There is actually only one Sequelize
instance in this db
object.
That instance is contained in a separate variable, sequelize
.
The instance is instantiated in the same file, index.js
, right above the db
object:
const sequelize = new Sequelize({
dialect: 'sqlite',
storage: 'movies.db',
// logging: false
});
2.) Why is the models object empty and why is it there at all?
The models
object is empty upon the instantiation of db
.
After this line is run, the Movie
model is added.
db.models.Movie = require('./models/movie.js')(sequelize);
3.) Why does this have (sequelize) at the end?
db.models.Movie = require('./models/movie.js')(sequelize);
This is just a nice shorthand version of returning the result of the function which is called from ./models/movie.js
.
You can think of it in two parts:
a.) require('./modles/movie.js')
calls the function from ./models/movie.js
b.) sequelize
, the instance is passed to that that function: (sequelize)
An alternative to writing this line could be something like this:
const Movie = require('./models/movie.js');
db.models.Movie = Movie(sequelize);
4.) I see no other imports with lowercase sequelize.
...
Movie.init(
{
title: Sequelize.STRING,
description: Sequelize.STRING,
year: Sequelize.NUMBER
},
{ sequelize }
...
Line 7 in ./models/movie.js
has a lowercase 's', which is an instance of Sequelize
.
Hope this has helped :)
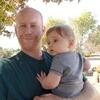
Jordan Kittle
Full Stack JavaScript Techdegree Graduate 20,148 PointsI was also confused by everything you were. I think this step went a little too far a little too fast with many things unexplained.
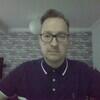
Brian Wright
6,770 PointsI took a bit of time away from this exercise to try and fully understand what we are creating. This is my undestanding:
- we have 'sequelize' which is an object representation (instance) of the actual database to which we pass any data required to find and connect to it.
- we have 'Sequelize' which is the package object which we use create the db instances and any models now or in future.
- we have a Model which is an object representation of a table in the actual database which to which we pass the fields and the database instance it will be attached to.
The sequelize instance contains a models object which holds all the models we create. as sequelize.models.
What this section of the course has done is to create one main db object containing all 3 of the above items { 1: a database instance, 2: the main Sequelize Object 3: the models from sequelize.models }
We also have the following files: 1: index.js - this file creates the db instance, the db object containing the 3 items above. It also adds any models we create to db.models.
2: movie.js - this creates a defines a model having be passed the database instance we want it to be connected to. This is a template for any future models we want to add. We can create a new js file like this with different table row information and add it to db.models in index.js. eg:
db.models.Movie = require('./models/movie.js')(sequelize);
db.models.Actor= require('./models/actor.js')(sequelize);
3: app.js the entry point for the app where we have the db object to allow us to create, update and delete to and from the model and any future models. We can have access to any and all future models i.e tables in the actual database, through db,models, e.g:
const { Movie, Actor, Director, Review } = db.models;
Hope there is some useful information in here as I found it important to get a good understanding of what sequelize is actually doing.
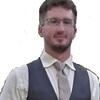
Sean Paulson
6,669 PointsYes it took me a bit to wrap my head around all of this. It exposed my lack of knowledge and all it is is... Modules. Just go back and study modules and why node uses common JS modules and not ECMAScript 6. Read some of the links in the teachers notes.
also here are some reading materials that helped me. https://2ality.com/2014/09/es6-modules-final.html https://addyosmani.com/writing-modular-js/ https://nodejs.org/api/modules.html
gerardo keys
14,292 Pointsgerardo keys
14,292 PointsYes it has helped. Thank you :)
Dat Pham Quoc
Full Stack JavaScript Techdegree Graduate 19,301 PointsDat Pham Quoc
Full Stack JavaScript Techdegree Graduate 19,301 PointsIs it necessary to include the uppercase "Sequelize" in "db" object and why if it is ? I tried to remove it and everything still work the same.
Brad Chandler
15,301 PointsBrad Chandler
15,301 PointsDat Pham Quoc it isn't "necessary" to export Sequelize in the db object in the code we write in this app.
However, they explain that when we do, we are "exposing the Sequelize package wherever" models are imported to your code so you wouldn't have to require it at any time you imported db. The Sequelize object would be available under db.Sequelize.
Mark Westerweel
Full Stack JavaScript Techdegree Graduate 22,378 PointsMark Westerweel
Full Stack JavaScript Techdegree Graduate 22,378 PointsNot all heroes wear capes. THANK YOU