Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial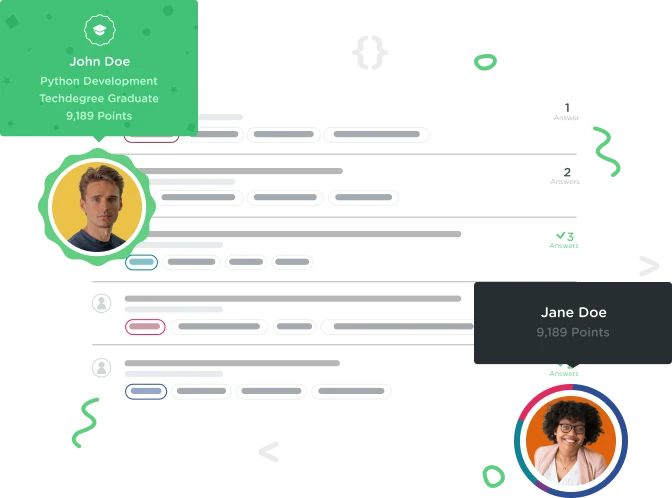
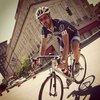
Sean Craig
5,178 PointsIm struggling with how to initialize the array for the grocery shopping list.
I cant figure out what I'm doing wrong trying to initialize the array.
#import "UIViewController.h"
@interface ViewController : UIViewController
@property (strong, nonatomic) NSArray *shoppingList;
@end
#import "ViewController.h"
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Add your code below!
NSArray *shoppingList = [[NSArray alloc] initWithObjects:@"toothpaste", @"bread", @"eggs", nil];
}
@end
4 Answers
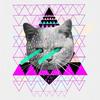
Stepan Ulyanin
11,318 PointsHi! First of all you already have the shoppingList property declared in the interface of the controller, so in your implementation it would be something like:
- (void)viewDidLoad {
[super viewDidLoad];
// Add your code below!
self.shoppingList = [[NSArray alloc] initWithObjects:@"toothpaste", @"bread", @"eggs", nil];
}
Other than that, the array initialization seems fine!
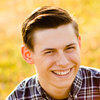
Caleb Kleveter
Treehouse Moderator 37,862 PointsI'm not quite sure what you mean.
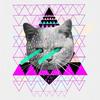
Stepan Ulyanin
11,318 PointsAccording to the apple reference:
Although itβs best practice for an object to access its own properties using accessor methods or dot syntax, itβs possible to access the instance variable directly from any of the instance methods in a class implementation. The underscore prefix makes it clear that youβre accessing an instance variable rather than, for example, a local variable.
When you use the _variable
, it is the instance variable and it is not recommended to access it directly unless you have the real reason to
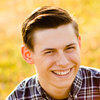
Caleb Kleveter
Treehouse Moderator 37,862 PointsInteresting, good to know.

Mahesh Babu
3,402 PointsHi,
You have declared a property of type shoppingList, so all you have to do is use _ shoppingList variable, but i would definitely recommend self.shoppingList as that is the standard syntax unless you are using an initializer , you should not use the _shoppingList.
I would definitely let the standard syntax go off the box and do the literal syntax nice and clean,
self.shoppingList = @[@"toothpaste", @"breads",@"eggs"];
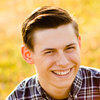
Caleb Kleveter
Treehouse Moderator 37,862 PointsTry this:
_shoppingList = [[NSArray alloc] initWithObjects:@"toothpaste", @"bread", @"eggs", nil];
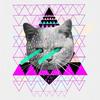
Stepan Ulyanin
11,318 PointsWhy would you mess up with the instance variable in this case? I mean isn't it a bad practice to mess around with the instance variable unless you are writing the initialization method?