Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial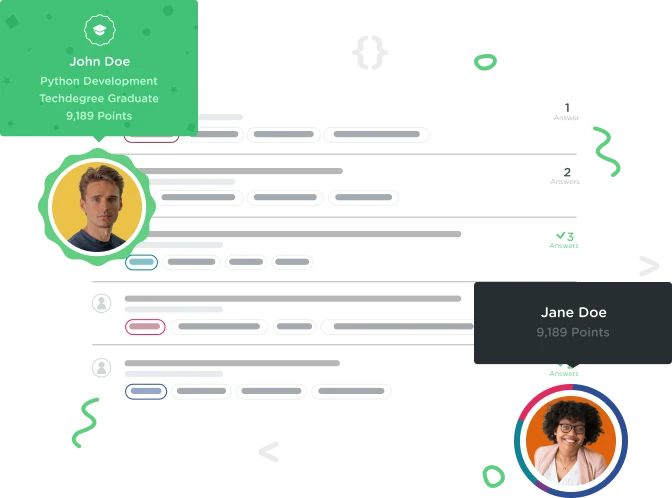

Andrés Lezama
554 PointsI'm stuck.
Hi, can somebody please give me the answer to "Overload Methods"? I'm trying to complete the second task but I'M STUCK.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int lapsAmount) {
lapsDriven++;
barCount--;
lapsDriven += lapsAmount;
barCount -= lapsAmount;
}
public void drive1();
}
3 Answers

Oleksiy Slisenko
7,002 PointsDear Andrés !
When You overload method You have to use the same name of a function, but with different parameter list, e.g.:
Original method:
public void drive() {
lapsDriven++;
barCount--;
}
and, overloaded method:
public void drive(int lapsAmount) {
lapsDriven += lapsAmount;
barCount -= lapsAmount;
}
Notice, that the function name drive is the same in both implementations, but the code and parameter list are defferent. Good luck !

Oleksiy Slisenko
7,002 PointsP.S. Sorry ! I wrote functions, but had in mind methods !!! :-)
And, of course, both methods got to be implemented under the same object.

Andrés Lezama
554 PointsHi jenna, thanks for the answer. So it should be public void(), but I dont know what goes inside the code block to call the first method.
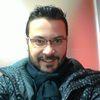
Rafael Miranda
17,121 PointsHi Andrés,
if you want to call the first method in the second just call it like:
public void drive1(){
drive();
}
Jenna Dercole
30,224 PointsJenna Dercole
30,224 PointsHi Andres,
When overriding methods, the first thing you want to check for is that both methods' names match. Your last method should be named "public void drive() {}" -- you can remove the 1. The question is asking you to override the first drive() method, this time without parameters. When you define the second method, nothing should be inside the parentheses, which is what you have--good. Then, in the body of the second method, call your first drive() method passing in '1' as a parameter there.
Try that and let me know if that fixes your error.