Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial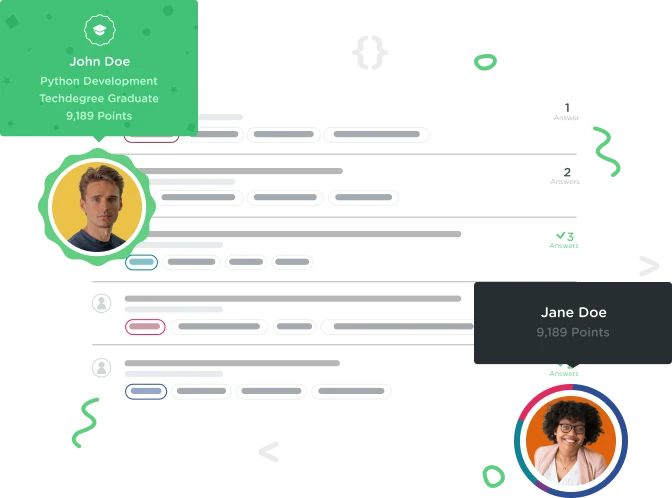

Lerena Holloway
9,855 PointsI'm stuck
Not exactly sure what's the issue here...it works just fine in my command line, but there must be one thing that's throwing it off.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
new_dict = {}
string = string.split(' ')
string = string.strip()
string = string.lower()
for word in string:
new_dict.update({word: string.count(word)})
return new_dict
2 Answers
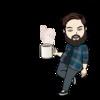
Philip Schultz
11,437 PointsHey Lerena, Remeber when you use the split method you're turning the string into a list. Also, you don't need to put quotations in the parenthesis of the split function.
This is how I did the challenge.
def word_count(some_string):
some_list = some_string.lower().split()
some_dict = {}
for word in some_list:
some_dict[word] = some_list.count(word)
return some_dict
Notice how I used lower() and split() on the same line. If you do it this way remember that you have to use lower() first then the split(). You don't need to strip anything.
Then I created an empty dict (you did too good job).
then I looped through our new list and assigned it to a value in the dict. Let me know if you have questions and I'll try to answer the best I can.
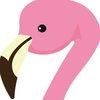
Dave StSomeWhere
19,870 PointsIf it runs in your command, what do you get if you run (adding extra spaces between words):
print(word_count("I do not like it sam I am"))
- The split() needs to be fixed (no value is needed), it can also be combined with lower() - you don't need separate lines
- The strip() is unnecessary an can be removed

Lerena Holloway
9,855 PointsThanks so much! I was able to rearrange the methods and drop the strip and it worked.
Lerena Holloway
9,855 PointsLerena Holloway
9,855 PointsWow, thanks so much! I never knew that I had to put the lower method before the split method like that. That's all I needed to change and it ran. Thanks!!