Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial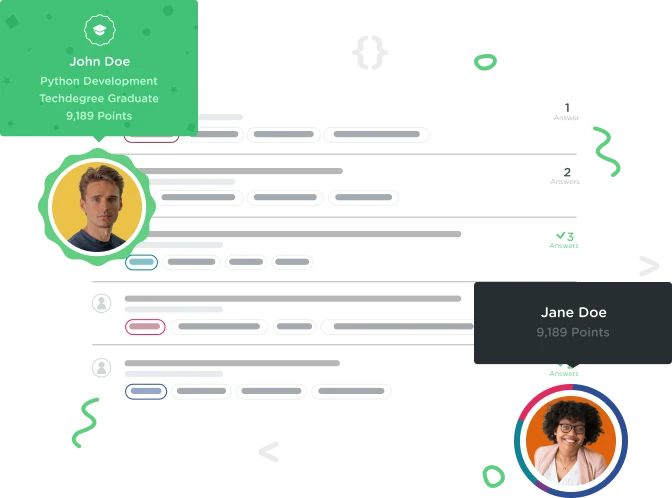

Cristian Gerardo Hernandez Barrios
28,382 PointsI'm stuck again
We have a page with 3 buttons. Our goal is to apply the spinElement callback to btn1, btn2, and btn3. The spinElement function should be triggered on the click event. Modify the code in the app.js file to add the event listener to every button.
This is my error Bummer! Did you use addEventListener with the 'click' event on btn1?
I put the addEventListener to my buttons, i dont know what is the error. Please help i think is something little
const btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
function spinElement(event) {
//Applies spinning animation to button element
btn1.addEventListener ('click', event => {
event.target.className = "spin";
});
btn2.addEventListener ('click', event => {
event.target.className = "spin";
});
btn3.addEventListener ('click', event => {
event.target.className = "spin";
});
}
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<link rel='stylesheet' href='styles.css'>
</head>
<body>
<section >
<button id='button1'>Button 1</button>
<button id='button2'>Button 2</button>
<button id='button3'>Button 3</button>
</section>
<script src='app.js'></script>
</body>
</html>
4 Answers
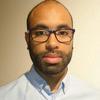
Ricardo Martins
16,909 Pointsconst btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
const spinElement = event => {
//Applies spinning animation to button element
event.target.className = "spin";
};
btn1.addEventListener ('click',spinElement);
btn2.addEventListener ('click', spinElement);
btn3.addEventListener ('click', spinElement);

Matthew Caloger
12,903 PointsThe spinElement() method adds the class "spin" to the given element from event.
Create all three event handlers outside of the spinElement() method, then within the event handlers, call the spinElement, passing along the event handler's event object.
const btn1 = document.getElementById("button1");
const btn2 = document.getElementById("button2");
const btn3 = document.getElementById("button3");
function spinElement(event) {
//Applies spinning animation to button element
event.target.className = "spin";
}
btn1.addEventListener ('click', event => {
spinElement(event)
});
btn2.addEventListener ('click', event => {
spinElement(event)
});
btn3.addEventListener ('click', event => {
spinElement(event)
});

Cristian Gerardo Hernandez Barrios
28,382 PointsThank yo, it's works

Joe Beltramo
Courses Plus Student 22,191 PointsYou need to add spinElement
as the callback to each button. Do not modify the spinElement
method.
You are calling the addEventListener
correctly just with the wrong method in the wrong place.
buttonElement.addEventListener('click', methodNameGoesHereAsCallback);
Hope that helps

Cristian Gerardo Hernandez Barrios
28,382 PointsThanks for the help :D

Robert O'Toole
6,366 Pointsanother good way outside of this course to do something like this is to use a forEach loop.
you can't add an event listener to multiple or all buttons at once without a forEach loop. so in this case you would define all the buttons with const buttons=querySelectorAll('button') then do something like this
buttons.forEach(button=>button.addEventListener('click', then the function name goes here));
this will allow for the same function to handle ALL the buttons in a single line of code rather than this rather tedious way.
Mike Siwik
Full Stack JavaScript Techdegree Student 8,483 PointsMike Siwik
Full Stack JavaScript Techdegree Student 8,483 PointsWrong, you're missing a lot of syntax