Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial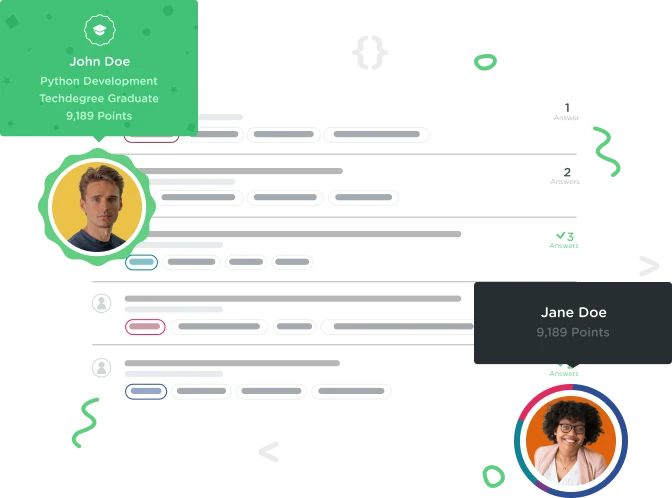

Joe Steffy
3,012 PointsI'm stuck again. What am I missing?
I'm stuck again. What am I missing?
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive() {
drive(MAX_BARS);
}
public void drive(int laps) {
mBarsCount -= laps;
drive(1);
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
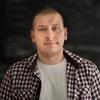
Gergő Bogdán
6,664 PointsHi,
Not sure what is your issue, but this code:
public void drive() {
drive(MAX_BARS);
}
public void drive(int laps) {
mBarsCount -= laps;
drive(1);
}
can cause stack overflow, because you are recursively invoking the drive(1) method and you don't have a stop condition. So if you invoke drive()
, whicl will invoke drive(8)
, then mBarsCount -= 8
will be executed and the drive(1)
method is invoked AGAIN, it enters in drive(int laps)
method, executes mBarsCount -= 1
at this moment mBarsCount
has value -9 AND invokes drive(1)
AGAIN and in the next step you will have mBarsCount
value -10 and so on.
I don't know exactly what you want to implement, but I suppose you need to stop, lets say when the mBarsCount
will be less or equal to 0 (mBarsCount <= 0
):
public void drive(int laps) {
mBarsCount -= laps;
if (mBarsCount <= 0) {
return;
} else {
drive(1);
}
}

Craig Dennis
Treehouse TeacherHINT: Using method signatures, add a new default method called drive that performs just 1 lap and takes no arguments.
Highlighting mine!