Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial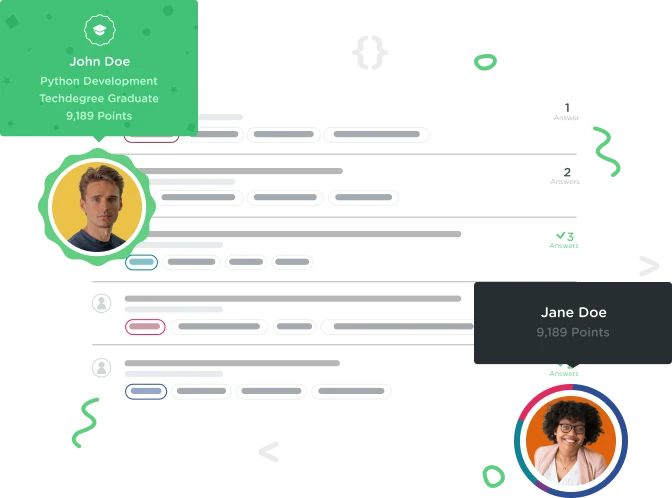

marcihoward3
5,226 PointsI'm stuck. I don't have a question. I'm "tapping out". What am I doing wrong?
What am I doing wrong here? 30 minutes on the question means I clearly don't understand something essential to finding the answer.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
var printNum = 0;
Console.Write("Enter the number of times to print \"Yay!\": ");
var entry = Int32.Parse(Console.ReadLine());
try
{
var input = Int32.Parse(entry);
}
catch (FormatException)
{
Console.WriteLine("You must enter a whole number.");
continue;
}
for (int i = 0; i < entry; i++){
Console.WriteLine("Yay!");
}
}
}
}
2 Answers

srikarvedantam
8,369 PointsI am noticing a couple of issues with the posted code:
var input = Int32.Parse(entry);
The "entry" argument is already of type "Int32" (see it's earlier declaration / definition that uses String parsing, which is read from Console, to Int32), but it is not of type "String". And, "Int32.Parse(...)" doesn't support parsing an Int32 value to Int32 value again (as there is no need)!
continue;
The above statement should be used in the context of a "loop". And, there is no enclosing loop here. So, this usage is incorrect.

srikarvedantam
8,369 PointsHow about the following solution?:
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
String userInput = Console.ReadLine();
int numTimes = 0;
try {
numTimes = int.Parse(userInput);
}
catch(FormatException) {
Console.WriteLine("You must enter a whole number.");
}
for(int i = 0; i < numTimes; ++i) {
Console.WriteLine("Yay!");
}
}
}
}

marcihoward3
5,226 PointsI appreciate your help, very much. I was stuck and could not make sense of what I was missing. Seeing it, helps me to understand where I made the error (and highlighted a concept that I hadn't understood (and, of course, didn't realize I didn't...).
Thanks again!