Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial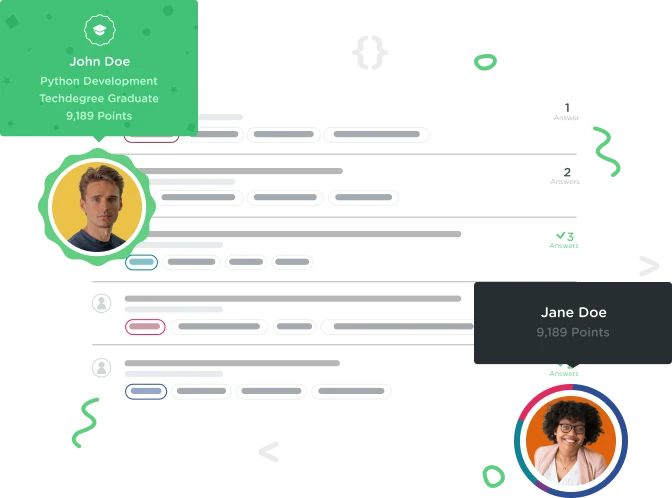

Tai Do
2,312 PointsIm stuck! I don't understand what the question is asking!!!!!
I've created a tool to help manage lines at tech conferences. The organizers would like to split the attendees into two lines during the registration process. I've added notes, examples and a new lesson in the Example.java tab.
Fix the getLineNumberFor method to return a 1 if the first character of lastName is between A and M or else return 2 if it is between N and Z.
================================================================================================
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
4 Answers

Boban Talevski
24,793 PointsWell, in short, in ConferenceRegistrationAssistant.java
, you need to write the code for the method getLineNumberFor
.
What that code needs to do, is check the first character of the passed String lastName
(we assume that character is in uppercase). If that character is between A-M, the method should return 1, and if it's between N-Z it should return 2. Comparing chars is much like comparing numbers, so >= and <= can be used to compare and conclude if a certain character falls within one of the specified ranges and act accordingly. I'm assuming you don't want the exact code to do this, but I can provide that if you want.
Another note is that Example.java
is just there for reference, you don't need to write any code there, but you can check the examples to see how chars are compared and see the actual use of the method getLineNumberFor
you would implement in ConferenceRegistrationAssistant.java
.
Updated with code:
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
char lastNameFirstChar = lastName.charAt(0);
if (lastNameFirstChar >= 'A' && lastNameFirstChar <= 'M') {
lineNumber = 1;
} else {
lineNumber = 2;
}
return lineNumber;
}
I checked and this works to complete the challenge, although it can be done in a different way. Let me explain:
We are getting the first letter of the last name as a char variable named lastNameFirstChar
by using the method charAt(0)
on the string lastName
which is passed as a parameter to our method getLineNumberFor
. So for lastName
"Anderson" we are getting the char 'A', for lastName
"Johnson" we are getting the char 'J' etc.. I guess you get the idea.
Then we are checking if that letter (variable lastNameFirstChar
) is equal or greater than A (alphabetically is equal or comes after A) AND (by using && in the code) that the same letter is equal or lower than M (alphabetically is equal or comes before M). If that's the case, it means the first letter was indeed between A and M alphabetically, so we set the lineNumber
variable to the value of 1 as that's what our task requested for the method to do.
Now, my code is saying in all other cases (the else code block) set the lineNumber variable to 2 which assumes if it isn't between A-M, then it must be between N-Z. You could do the same check and write another if statement instead of the else statement and check if the letter is indeed between N-Z, in which case, if true, you would set the lineNumber
variable to 2 and there would be no else statements.
In the end of method, we are returning the value of the variable lineNumber
which can be either 1 or 2 depending on which of the conditions in the if/else statement was met and to what value the variable lineNumber
was set. So we return 1 if the first letter was between A-M and we return 2 if it was between N-Z as the task requested.

Heather Malloch
2,141 Points public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lastName.charAt(0) <= 'M') {
lineNumber = 1;
} else {lineNumber = 2;
}

Jon-Ryan Maloney
5,704 PointsGood answer, Heather, but the system wants return statements.

Heather Malloch
2,141 PointsThis passed, maybe I missed the return when copying, oops!
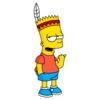
Will Hunting
13,726 PointsI would like to see exact code if possible as I am also struggling to get my head around this one,,

Boban Talevski
24,793 Pointsupdated the original answer with code
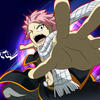
Sangam Thapa
5,669 Pointspublic class ConferenceRegistrationAssistant {
/**
- Assists in guiding people to the proper line based on their last name. *
- @param lastName The person's last name
-
@return The line number based on the first letter of lastName / public int getLineNumberFor(String lastName) { int lineNumber = 0; / lineNumber should be set based on the first character of the person's last name Line 1 - A thru M Line 2 - N thru Z
*/ char firstLetter= lastName.charAt(0); char forComparision='M'; if(firstLetter <= forComparision){ lineNumber+=1; }else{ lineNumber+=2; } return lineNumber; }
}

Morgan Overstreet
2,779 PointsString lastNameLetter = lastName.substring(0, 1); if (lastNameLetter <= "M") { lineNumber = 1; } else { lineNumber = 2; }
Why doesn't this code work?
Tai Do
2,312 PointsTai Do
2,312 PointsSorry for selecting best answer late. I thought I had done it already, but I did not. My apologies.