Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial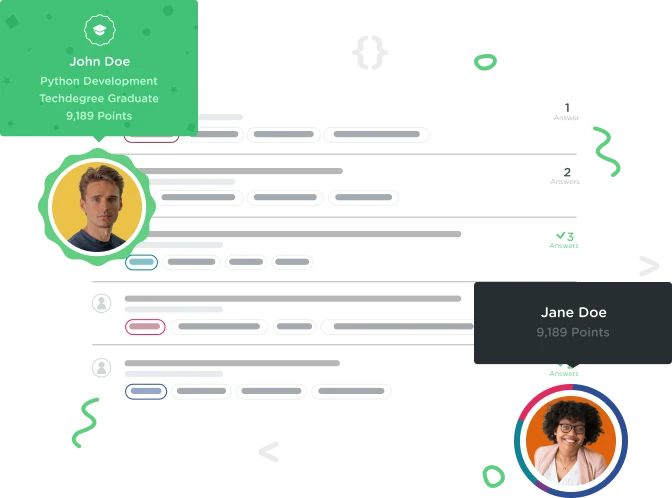

Juan Prieto
976 PointsIm stuck in the second part. Help me!
I want to know how to solve this, but no with the answer . Could you help to find the logic to solve this? I was thinking to use "for" to loop in every single letter that matches tile.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
public int getCountOfLetter ( char letter){
getCountOfLetter =0;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
1 Answer

Manish Giri
16,266 PointsYou're on the right track. Use the for loop to go through the characters in tiles
. For each character that matches letter
, increment count
by 1. Finally, return count
at the end.
Juan Prieto
976 PointsJuan Prieto
976 Pointspublic int getCountOfLetter ( char letter){ getCountOfLetter = 0; for ( char tile : char letter){ if (char tile = char letter){ getCountOfLetter +=; }
return getCountOfLetter;
Am I wrong?
Manish Giri
16,266 PointsManish Giri
16,266 PointsYes, there are quite a few things you need to fix.
Method names and variable names
First, the name of the method is
getCountOfLetter
. But if you look in your code, you have -getCountOfLetter = 0;
, and the last line -return getCountOfLetter;
. This is like saying assign0
to the method name, and return the method name itself from within the method. Instead of that, create anint
variable in the method, to which you add the count. Then return this variable.For Each Loop
Next, the way for-each loop works in Java is you first have an array (any collection) of things to begin with. Then you use a temporary variable to loop over the collection, one at a time. So, if
array
was your collection, you could create the loop like this -Now look at your for-each loop -
char tile : char letter
Both these elements are just individual characters, there's no collection to iterate over.
Type Declaration
When you use variables in a method, you declare their types only once, the first time you create the variables. After that, you just use the variable name itself. For example, consider -
char tile
. If your code uses this variable in a hundred places, you wouldn't writechar tile
a hundred times. Instead, the first time you declare the variabletile
, you write it's type aschar
, and then on, you just writetile
.Like in your code, you have -
public int getCountOfLetter ( char letter){
This says that your method is expecting an argument calledletter
of typechar
. So from here on out, you just need to writeletter
, notchar letter
.Fix these things, and give it a shot!