Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial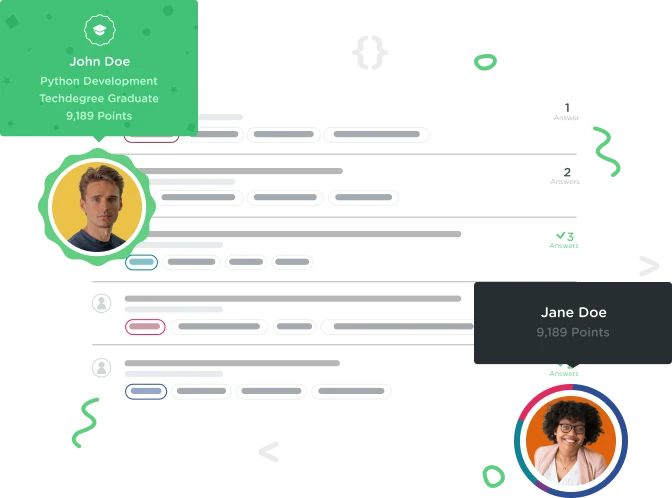

Carla Barrios
636 PointsI'm stuck in this challenge. Can someone tell me what am I doing wrong?
For this first task, create a new private method named normalizeDiscountCode. It should take the discount code that is passed into the method and return the uppercase version. Call it from the current applyDiscountCode method and set this.discountCode to the result.
private String ApplyDiscountCode(String discountCode){
this.discountCode = normalizeDiscountCode(String discountCode);
return discountCode.toUpperCase();
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = discountCode;
}
private String ApplyDiscountCode(String discountCode){
this.discountCode = normalizeDiscountCode(String discountCode);
return discountCode.toUpperCase();
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

simeon
1,549 PointsI was really stuck on this challenge as well ;) What I'm thinking to spot in your code are a couple of the following points:
First it looks like the last method is indeed misspelled. It should be private String normalizeDiscountCode (String discount)
Secondly, the task asks us to call normalizeDiscountCode inside the applyDiscountCode method. Also set that call equal to this.discountCode.
I myself did not change the applyDiscountCode into a void method, but left it returning the discountCode, but I guess that is just a matter of what you want.
Hope it helps and enables you to continue with the challenges!
public String applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
return discountCode.toUpperCase();
}
Teacher Russell
16,873 PointsTeacher Russell
16,873 PointsIs it possible you've misnamed your function? Should be private String normalizeDiscountCode.