Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial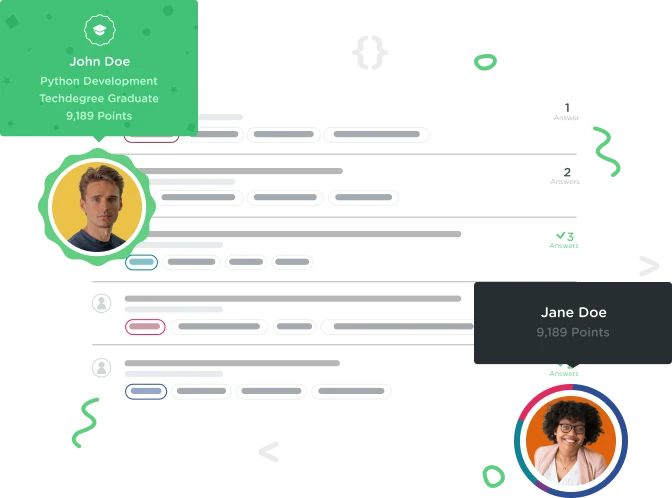
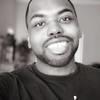
Alphonso Sensley II
8,514 PointsIm stuck!! Ive been working on this one for past day, but can't seem to switch the colors correctly. Please Help!
I see the instruction that say "In the implementation of the method, simply change the colors" but I can't figure out how to do this. Do I need to call switchColor after I create an instance of WifiLamp?? Any help will be greatly appreciated!
// Declare protocol here
protocol ColorSwitchable {
func switchColor (_ color: Color)
}
enum LightState {
case on, off
}
enum Color {
case rgb(Double, Double, Double, Double)
case hsb(Double, Double, Double, Double)
}
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
init(state: LightState, color: Color) {
self.state = state
self.color = color
}
func switchColor (_ color: Color) {
}
}
let lampUser = WifiLamp(state: .off, color: .hsb(1.0, 1.0, 1.0, 1.0))
1 Answer
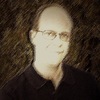
Jason Anders
Treehouse Moderator 145,858 PointsHey Alphonso,
You're on the right track, but there are a some issues with the code:
- First, you altered the
init
method, and the challenge did not ask you to. Challenges are very specific and picky, so if it didn't tell you to do something... don't. So, you will need to reset the challenge to restore the original pre-loaded code. - You don't have any code in the overriding function? In the
WifiLamp
class, the function needs to do something. In this case, the challenge say to "simply change the colors" so you need to assignself.color
the value ofcolor
coming in from the parameters passed into the function. - The function is like a variable and needs to come before the
init
method. So, you just need to move it above theinit
. - The challenge didn't ask you to call the function. This goes back to the first point... so just delete that line of code.
Below is the corrected code for the Class. Once you correct the above, everything else looks good and the code will pass. :)
class WifiLamp: ColorSwitchable {
let state: LightState
var color: Color
func switchColor (_ color: Color) {
self.color = color
}
init() {
self.state = .on
self.color = .rgb(0,0,0,0)
}
}
Keep Coding!
Alphonso Sensley II
8,514 PointsAlphonso Sensley II
8,514 PointsHey Jason,
Thank you so much. That really helped. I started over after reading your instruction and got it! I like to understand what each section of code is doing instead of just getting the right answer. Your steps helped me gain that understanding! Thank you!