Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial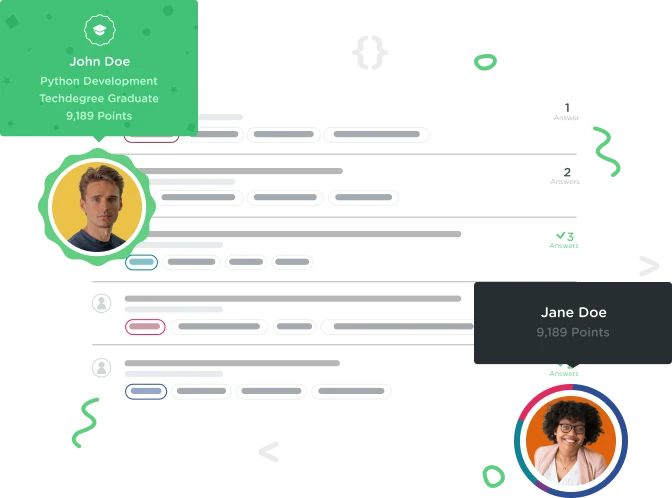
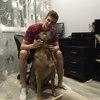
Arthur Kharzamanov
5,414 PointsI'm stuck on a challenge, please help!
I don't understand why system.out is not showing the 1 Yoda PEZ Dispenser. I added the following code to shoppingcart.java and have uncommented cart.addItem(dispenser) :
public void addItem(Product item) { System.out.printf("1 %s.\n", item.getName()); }
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item) {
System.out.printf("1 %s.\n", item.getName());
}
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.\n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
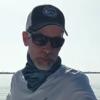
Corey Johnson
Courses Plus Student 10,192 PointsLooks like you are very close. All i did to complete the challenge was to uncomment the line "cart.addItem(dispenser);" in Example.java.
Then in ShoppingCart.java.. i copied the existing addItem method... and changed it to the below:
public void addItem(Product item) {
System.out.printf("Adding %d of %s to the cart.\n", 1, item.getName());
}
Hope this helps Arthur!
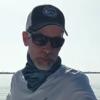
Corey Johnson
Courses Plus Student 10,192 PointsHello Colby,
You would have to check for 0 instead. The primitive int type variable will default to 0 instead of null if it is not initialized. So instead, your code should look like this:
public void addItem(Product item, int quantity) {
if(quantity != 0){ // or maybe > 0 would be even better
System.out.printf("Adding %d of %s to the cart.\n", quantity, item.getName());
} else {
quantity = 1;
System.out.printf("Adding %d of %s to the cart.\n", 1, item.getName());
}
}
But the challenge wants you to use a different addItem method that only takes a Product object as a parameter.
Hope this helps.

Colby Wise
3,165 PointsThat does. Last question...a bit more of a noob question. How do you insert your code snippet like that? Mine is all orange etc where as yours methods, args, etc are clear/ color coded
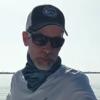
Corey Johnson
Courses Plus Student 10,192 PointsNo worries. For Java.. you use 3 back ticks and then the word java. The back tick is below the tilde on your keyboard. So you do 3 back ticks then "java" and then your java code.. and then close with 3 back ticks. You can find more info by clicking the Markdown Cheatsheet at the bottom of this page.
Let me know if i can help with anything else Colby.
Colby Wise
3,165 PointsColby Wise
3,165 PointsHey Corey - thanks. Any idea why using a [null] check in the original addItem method doesn't work or isn't a better solution. Code below: