Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial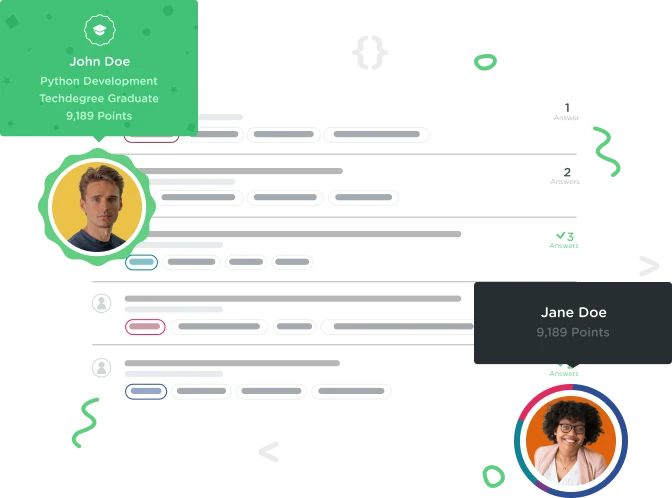
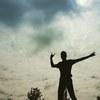
Maxmillan Muchena
2,478 PointsI'm stuck on disemvowel challenge. Need help
I do not know how I can improve my code that it removes all the vowels if they are being repeated. I think the for loop is the problem but I do not know what condition I would use for a while loop.
def disemvowel(word):
list_of_words=list(word.lower())
for i in (list_of_words.copy()):
while true
try:
list_of_words.remove("a")
list_of_words.remove("e")
list_of_words.remove("i")
list_of_words.remove("o")
list_of_words.remove("u")
except ValueError:
pass
x= ''.join(list_of_words)
print (x)
word=str(input("Enter a word "))
disemvowel(word)
2 Answers
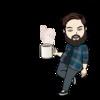
Philip Schultz
11,437 PointsHey Maxmillan, Here is how I solved the problem
def disemvowel(word):
new_word = ''
vowels = ["a", "e", "i", "o", "u"]
for letter in word:
if letter.lower() not in vowels:
new_word += letter
word = new_word
return word
First, I created a new_word variable so I could create the word given without the vowels. Remember that strings are immutable (That is why you have to build a new string variable); there isn't a remove function for strings. Also, notice how I made the vowels into a list, this way it could be easily looped through. Let me know if you have any questions.
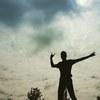
Maxmillan Muchena
2,478 Pointswell explained indeed .Thank you.
Maxmillan Muchena
2,478 PointsMaxmillan Muchena
2,478 PointsThanks it worked and I understand the logic behind it.Could you also assist me in finding the reason why my code only removes some of the vowels and how I can improve it. Its failing to remove all vowels if they are repeated .
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsHey Max, When you use the .remove method in a list, it will only remove the first instance of that value then it will quit. Also, where you are making the word into a list at the very top of your code, you're making a list of lowercase letters. When you do this, then your list is only capable of checking for lowercase vowels and it doesn't remember what letters were uppercase. Notice in my code where I'm using the .lower() method. I'm not changing the value of the string by using it in the if statement. You can read the if statement I made like this - If the lowercase version of this letter is not in the list of vowels then do this.
If you wanted to turn the string into a list and check it that way, you could do so like this.