Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial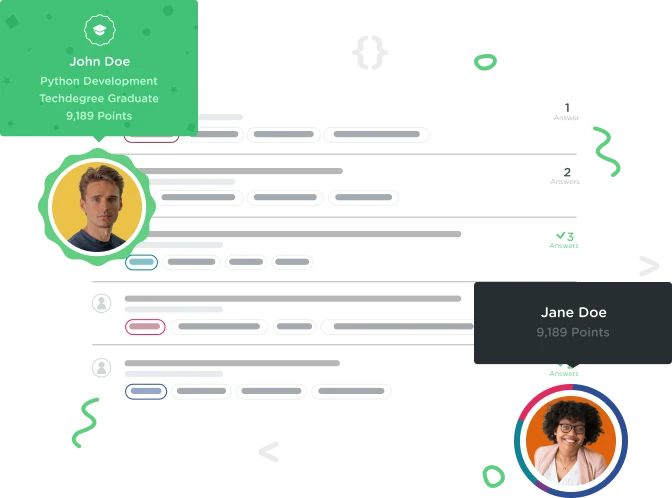
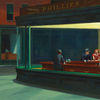
Anthony Grodowski
4,902 PointsI'm stuck on the challange. Can someone give me a hint or if I've done something wrong?
The whole idea is that the loop will go trough every word of the string and then try to find the same one in the string. I don't know how to solve adding to the dict problem. My code will put same keys and values several of times. Is there a way to delete duplicated items of the final dict? Please show me every mistake I've done and some hints how to complete the challange!
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(smth):
index = 0
blah = 0
new_dict = {}
new_dict_2 = {}
words = smth.lower()
words = words.split()
while words:
try:
if words[blah] == words[index+1]:
new_dict_2.update({words[0]: index+2})
index += 1
continue
else:
new_dict.update({words[index]: 1})
index += 1
continue
except IndexError:
if blah >= len(new_dict):
blah += 1
index2 = 1
index = index2
index2 += 1
continue
else:
break
return new_dict.update(new_dict_2)
1 Answer
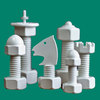
Steven Parker
231,269 PointsHere's a few hints to help get you back on track:
- you can do this without nesting loops
- a loop that tests something that does not change will never end
- you must first create a dictionary variable before you can append onto it
- the last thing the function should do is return the new dictionary
- (this won't cause an error, but) "continue" is not needed at the end of a loop body
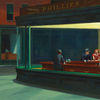
Anthony Grodowski
4,902 PointsThanks Steven! First 4 hints I've found into my code by myself (check out updated code) just after posting this question, but 5th is not clear to me. How doesn't it cause an error? After a few loops time index will be greater than the number of items inside the list, so it will cause an IndexError as far as I'm concerned... Another thing is - how is it possible that "continue" isn't recquired after "except IndexError" part? I need to reset my values there to tun this code again properly. Please check out updated code, it's almost working but if I for example test it with "I do not like it Sam I Am" string, in new_dict i get {'i': 1, 'do': 1, 'not': 1, 'like': 1, 'it': 1} and in new_dict_2 {'i': 7} which is clearly not right and these lists combined by update function return None.
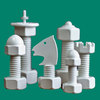
Steven Parker
231,269 PointsI don't think I understand the strategy implemented here, but here's a few more hints:
- for "
while words:
", how will "words" change in the loop to make this test useful? - you can do this more simplly, you only need one new dictionary
- you probably don't want any hard-coded indexes (like "
words[0]
")
It sounds like you're already testing outside of the challenge. You might try adding some "print"s to show the values of the variables at different points. You might discover that what's happening is different from your intentions.
And the default operation for a loop is to continue, so you don't need an explicit one unless there are other steps you need to avoid.
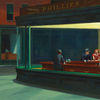
Anthony Grodowski
4,902 PointsThe strategy is:
- The loop searchs for recurring words. If there's one it creates a new_dict_2. Overall new_dict_2 is a dict with these words that are recurring (value assigned to the key is greater than 1).
- If the word isn't recurring in the string it is being nested in new_dict.
- In each loop it adds 1 to the index so the loop will compare next word to the first one, looking for the same word.
- If the loop went trough whole dict comparig first item to the rest, it moves on to compare second item to the rest (that's why I'm putting "blah" variable and increasing the index each time it gets to the IndexError) - that's why in my opinion "while words" (while True) is neccessary, cause I need to use "continue" and "break"
- Whole loop ends, when blah is equal or greater than number of items in the dict, cause it reached the point where all of the possible comparisions have been considered.
- At the end I'm updating new_dict with new_dict_2 to substitute words with value 1 with recurring words.
Now I'm struggling to obtain at the end a value greater than 1. Even if the words are reccuring it still shows 1... Also don't know how to swap these hard-coded indexes. I'm worried that I got the whole concept wrong and the code simply cannot be valid.
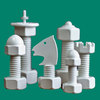
Steven Parker
231,269 PointsWell, it's probably not the easiest concept to implement. And it's a bit more complicated that you need for this task. Some ideas that might make things simpler:
- there are ways to tell if a dictionary contains a key that don't cause exceptions
- you only need to either create a new key (with a count of 1) or add to the count of an existing key
- you can keep track of the counts this way using only one new dictionary
- one iteration through the word list should give you the totals you need
See if those ideas lead you to a simpler (and successful!) solution.
Dave StSomeWhere
19,870 PointsDave StSomeWhere
19,870 PointsHint #1 - try running your code locally or in a workspace and post your results with your code. I think the first mistake is that you haven't worked on debugging your code which seems to be a great way to learn.