Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial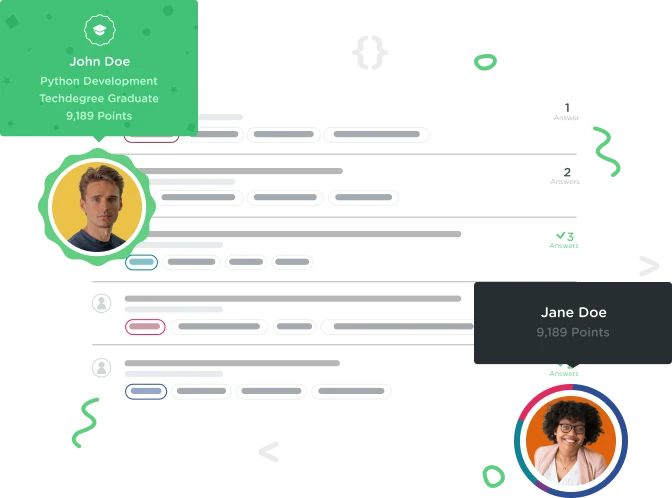

Ngqabutho Moyo
2,538 PointsI'm stuck on the challenge
I got 6 failures. What can I do to rectify?
from flask import Flask, g, render_template, flash, redirect, url_for
from flask.ext.login import LoginManager, login_user, logout_user, login_required, current_user
from flask.ext.bcrypt import check_password_hash
import models
import forms
DEBUG = True
PORT = 8000
HOST = '0.0.0.0'
app = Flask(__name__)
app.secret_key = 'let this be the secret key'
login_manager = LoginManager()
login_manager.init_app(app)
login_manager.login_view = 'login'
@login_manager.user_loader
def load_user(userid):
try:
return models.User.get(models.User.id == userid)
except models.DoesNotExist:
return None
@app.before_request
def before_request():
"""Connect to database before each request"""
g.db = models.DATABASE
g.db.connect()
g.user=current_user
@app.after_request
def after_request(response):
"""Disconnect from database after each request"""
g.db.close()
return response
@app.route('/register', methods=['GET', 'POST'])
def register():
form = forms.RegisterForm()
if form.validate_on_submit():
flash('Registered successfully', 'success')
models.User.create_user(
email= form.email.data,
password=form.password.data
)
return redirect(url_for('index'))
return render_template('register.html', form=form)
@app.route('/login', methods=['GET', 'POST'])
def login():
form = forms.LoginForm()
if form.validate_on_submit():
try:
user = models.User.get(models.User.email == form.email.data)
except models.DoesNotExist:
flash("Your email or password don't match", "error")
else:
if check_password_hash(user.password, form.password.data):
login_user(user)
flash('Logged in successfully', 'success')
return redirect(url_for('index'))
else:
flash("Your email or password doesn't match", "error")
return render_template('login.html', form=form)
@app.route('/logout')
@login_required
def logout():
logout_user()
flash('Logged out successfully', 'success')
return redirect(url_for('index'))
@app.route('/taco', methods=['GET', 'POST'])
@login_required
def taco():
form = forms.TacoForm()
if form.validate_on_submit():
models.Taco.create(user=g.user._get_current_object(),
protein=form.protein.data,
shell=form.shell.data,
cheese=form.shell.data,
extras=form.extras.data.strip()
)
flash('Taco has been added', 'success')
return redirect(url_for('index'))
return render_template('taco.html', form=form)
@app.route('/')
def index():
tacos = models.Taco.select().limit(10)
return render_template('index.html', tacos=tacos)
if __name__ == '__main__':
models.initialize()
try:
with models.DATABASE.transaction():
models.User.create_user(
email='moyongqaa@gmail.com',
password='password'
)
except ValueError:
pass
app.run(debug=DEBUG, host=HOST, port=PORT)
from peewee import *
import datetime
from flask.ext.login import UserMixin
from flask.ext.bcrypt import generate_password_hash
DATABASE = SqliteDatabase('taco.db')
class User(UserMixin, Model):
email = CharField(unique=True)
password = CharField(max_length=100)
class Meta:
database = DATABASE
def get_tacos(self):
return Taco.select().where(Taco.user == self)
def get_stream(self):
return Taco.select().where(Taco.user == self)
@classmethod
def create_user(cls, email, password):
try:
cls.create(
email=email,
password=generate_password_hash(password)
)
except IntegrityError:
raise ValueError('User already exists')
class Taco(Model):
user = ForeignKeyField(rel_model=User, related_name='tacos')
protein = CharField(default=None)
shell = CharField()
cheese = BooleanField(default=False)
extras = TextField()
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Taco], safe=True)
DATABASE.close()
from flask_wtf import Form
from wtforms import StringField, PasswordField, BooleanField, TextAreaField
from wtforms.validators import DataRequired, Length, EqualTo, ValidationError, Email
from models import User
def email_exists(form, field):
if User.select().where(User.email == field.data).exists():
raise ValidationError('User with that email already exists')
class RegisterForm(Form):
email = StringField('Email', validators=[DataRequired(), Email(), email_exists])
password = PasswordField('Password', validators=[DataRequired(), Length(min=8), EqualTo('password2', message='Passwords must match')])
password2 = PasswordField('Confirm Password', validators=[DataRequired()])
class LoginForm(Form):
email = StringField('Email', validators=[DataRequired(), Email()])
password = PasswordField('Password', validators=[DataRequired()])
class TacoForm(Form):
protein = StringField('Protein', validators=[DataRequired()])
shell = StringField('Shell', validators=[DataRequired()])
cheese = BooleanField('Cheese')
extras = TextAreaField('Any extras?')
<!doctype html>
<html>
<head>
<title>Tacocat</title>
<link rel="stylesheet" href="/static/css/normalize.css">
<link rel="stylesheet" href="/static/css/skeleton.css">
<link rel="stylesheet" href="/static/css/tacocat.css">
</head>
<body>
{% with messages=get_flashed_messages() %}
{% if messages %}
<div class="messages">
{% for message in messages %}
<div class="notification">
{{ message }}
</div>
{% endfor %}
</div>
{% endif %}
{% endwith %}
<div class="container">
<div class="row">
<div class="u-full-width">
<nav class="menu">
{% if current_user.is_authenticated() %}
<a href="{{ url_for('logout') }}" title="Log out">Log Out</a>
<a href="{{ url_for('taco') }}" title="Create taco">Create Taco</a>
{% else %}
<a href="{{ url_for('login') }}" title="Log in">Log In</a>
<a href="{{ url_for('register') }}" title="Sign up">Sign Up</a>
{% endif %}
</nav>
{% block content %}{% endblock %}
</div>
</div>
<footer>
<p>An MVP web app made with Flask on <a href="http://teamtreehouse.com">Treehouse</a>.</p>
</footer>
</div>
</body>
</html>
{% extends 'layout.html' %}
{% block content %}
<h2>Tacos</h2>
{% if tacos.count() %}
<table class="u-full-width">
<thead>
<tr>
<th>Protein</th>
<th>Cheese?</th>
<th>Shell</th>
<th>Extras</th>
</tr>
</thead>
<tbody>
{% for taco in tacos %}
<!-- taco attributes here -->
{% endfor %}
</tbody>
</table>
{% else %}
<!-- message for missing tacos -->
{% endif %}
{% endblock %}
2 Answers
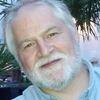
Jeff Muday
Treehouse Moderator 28,722 PointsTacoCat is a wonderful challenge of Flask full-stack application techniques! There are quite a few components that go together to make this complete. Hint -- this is a GOOD APP for your portfolio to show off to prospective clients/employers. It demonstrates mastery of the most important aspects of full-stack programming. I heartily encourage you to write it well and add your own spin to it.
Kenneth was hungry for tacos when he came up with the challenge. Rumor has it he was thinking of this place near "Treehouse Island" in Portland-- don't quote me on this ;-)
https://i.imgur.com/uWB85Hf.png
Not having the chance to run your code, I spotted you are using OLD versions of the library imports. These will definitely produce errors. Make sure to change your imports to reflect the ones shown below.
from flask_login import (LoginManager, UserMixin, login_user, login_required,
logout_user, current_user)
from flask_bcrypt import generate_password_hash, check_password_hash

Ngqabutho Moyo
2,538 PointsI tried. Still no luck
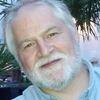
Jeff Muday
Treehouse Moderator 28,722 PointsTroubleshooting apps can be difficult. I recommend working in a good IDE that has better error identification-- for example Pycharm EDU/Community or Wingware Personal edition. I use the Wingware Pro, but the Personal edition is free and has great tools for web apps.
I saw a number of errors in your code:
the imports (I mentioned above) MUST be changed
there were a number of indentation issues, I expect you know how to locate and fix these.
Your program will run... but then you have a runtime error you have to fix
To fix that runtime error, you will have to modify "layout.html"
You have parenthesis with current_user.is_authenticated()
but that is a property rather than a function so the parenthesis are a syntax error.
{% if current_user.is_authenticated() %}
It should be this:
{% if current_user.is_authenticated %}

Ngqabutho Moyo
2,538 Pointsremoving parentheses adds a failure, unfortunately
boi
14,242 Pointsboi
14,242 PointsBeware the Tacocat challenge.