Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial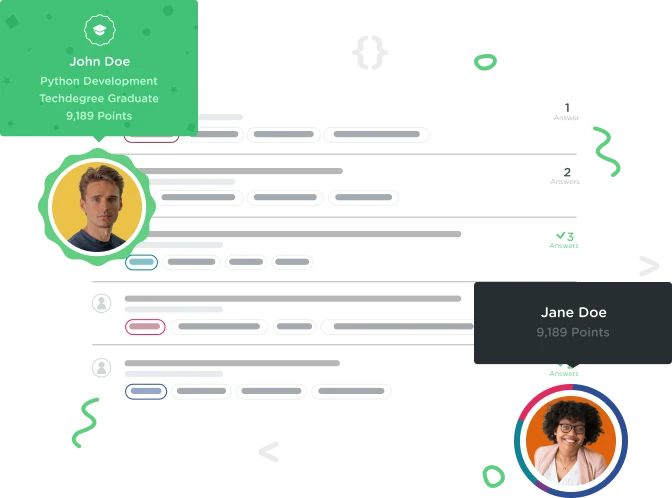

Steve Anderson
4,850 PointsI'm stuck on the first task. I'm trying to select the nested <li> elements and nothing works. Do I use querySelector?
I've tried all the major document methods and the best I can do is select all the <a> tags on the page. Am I supposed to add id's to the index.html elements that I want?
let navigationLinks = document.getElementsByTagName('nav ul li');
let galleryLinks;
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Nick Pettit | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Nick Pettit</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="index.html" class="selected">Portfolio</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="img/numbers-01.jpg">
<img src="img/numbers-01.jpg" alt="">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="img/numbers-02.jpg">
<img src="img/numbers-02.jpg" alt="">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="http://twitter.com/nickrp"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="http://facebook.com/nickpettit"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© 2016 Nick Pettit.</p>
</footer>
</div>
<script src="js/app.js"></script>
</body>
</html>
3 Answers
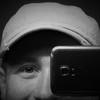
Ryan Rankin
8,261 PointsSo for this one since you're trying to select all of the links within the nav you're going to want to use the querySelectorAll() method. Like this:
let navigationLinks = document.querySelectorAll('nav a');
Good Luck and Happy Coding!

Steve Anderson
4,850 PointsThank you so much!

Peter Larson
6,412 PointsI found elsewhere that you can use something like this as well:
let navigationLinks = document.getElementsByTagName('nav')[0].getElementsByTagName('a');
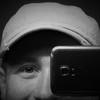
Ryan Rankin
8,261 PointsYeah, there are multiple ways of accessing the different elements. For the most part you can use whatever you feel comfortable with. "querySelector" and "querySelectorAll" are a little more flexible, and this specific challenge was obviously looking for "querySelectorAll". The flexibility within JavaScript and the different routes you can take to solve a problem I happen to enjoy, but also lead to a lot of frustration when I'm attempting to learn. All of the different methods that can be applied start to clutter my brain and it makes it difficult to remember what works best or which one I prefer the most. So, I just continue to practice over and over again until things begin to click and stick.