Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial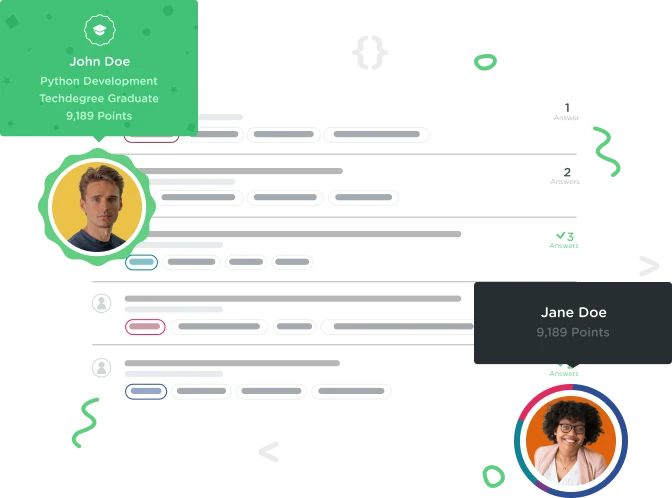
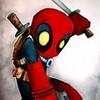
Alex Medalla
1,028 Pointsim stuck on this
could anyone explain this to me?
public class Tweet {
private String text;
public Tweet(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public static final int MAX_CHARS = 140;{
}
4 Answers
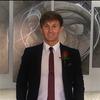
Liam Clarke
19,938 PointsOK lets break down the question, you have done this is the previous videos, it is just asking for a lot at once.
- Add a new constant that defines the max chars allowed and set it 140. Use proper case for constants. (A general rule for creating constants is to put them in full caps like below.)
int MAX_CHARS = 140;
- Ensure it is accessible off the class,
public int MAX_CHARS = 140;
- and that it cannot be changed.
public static final int MAX_CHARS = 140;
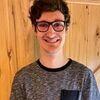
Trent Christofferson
18,846 PointsWhat Liam Clarke said but also you should put public static final int MAX_CHARS = 140; above public Tweet(String text). Also, you don't need the {} for it because it is not a method.
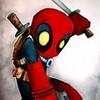
Alex Medalla
1,028 Pointsso where am I supposed to put this new line of code now?
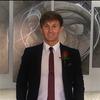
Liam Clarke
19,938 PointsI forgot to mention that part. Variables are usually declared at the top so put it next to the other variable which is already declared.
public static final int MAX_CHARS = 140;
private String text;
Don't get confused with the word constant, it is just a variable like you have been using, a constant is a variable that's value does not change, I hope this clears things up for you.
Apologies for the markup, I'm replying on my phone.
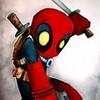
Alex Medalla
1,028 Pointsso should it look like this or do i need to put something in between the variables
'''public static final int MAX_CHARS = 140; private String text; public class Tweet { private String text;
public Tweet(String text) { this.text = text; }
public String getText() { return text; }
public void setText(String text) { this.text = text; } } '''
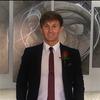
Liam Clarke
19,938 PointsThe correct answer to the challenge is:
public class Tweet {
public static final int MAX_CHARS = 140;
private String text;
public Tweet(String text) {
this.text = text;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public int getRemainingCharacterCount() {
return MAX_CHARS - text.length();
}
}
I'd recommend re-watching the previous 2 videos just to clarify the difference between methods and constants, i think you was mixing them up.
Good luck