Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial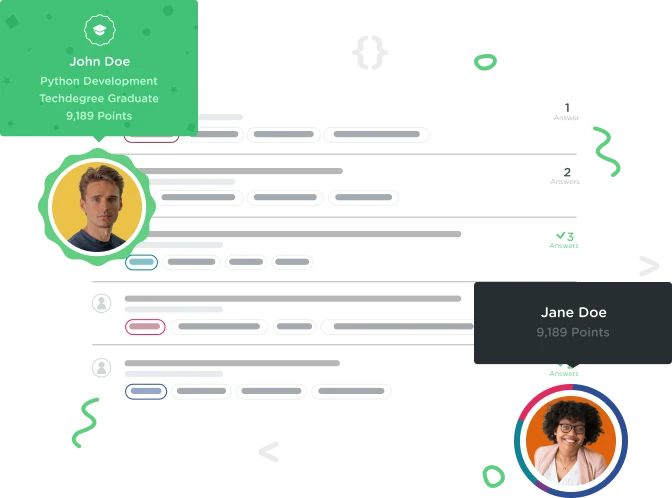
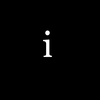
Furquan Ahmad
5,148 PointsI'm stuck on this login system, if i enter valid login details it won't redirect me to the right page.
<?php
require("config.php");
$submitted_username = '';
if(!empty($_POST)){
$query = "
SELECT
id,
username,
password,
salt,
email
FROM users
WHERE
username = :username
";
$query_params = array(
':username' => $_POST['username']
);
try{
$stmt = $db->prepare($query);
$result = $stmt->execute($query_params);
}
catch(PDOException $ex){ die("Failed to run query: " . $ex->getMessage()); }
$login_ok = false;
$row = $stmt->fetch();
if($row){
$check_password = hash('sha256', $_POST['password'] . $row['salt']);
for($round = 0; $round < 65536; $round++){
$check_password = hash('sha256', $check_password . $row['salt']);
}
if($check_password === $row['password']){
$login_ok = true;
}
}
if($login_ok){
unset($row['salt']);
unset($row['password']);
$_SESSION['user'] = $row;
header("Location: main.php");
die("Redirecting to: main.php");
}
else{
print("Login Failed.");
$submitted_username = htmlentities($_POST['username'], ENT_QUOTES, 'UTF-8');
}
}
?>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Bootstrap Tutorial</title>
<meta name="description" content="Bootstrap Tab + Fixed Sidebar Tutorial with HTML5 / CSS3 / JavaScript">
<meta name="author" content="Untame.net">
<script src="http://ajax.googleapis.com/ajax/libs/jquery/2.0.0/jquery.min.js"></script>
<script src="assets/bootstrap.min.js"></script>
<link href="assets/bootstrap.min.css" rel="stylesheet" media="screen">
<style type="text/css">
/*body { background: url(assets/bglight.png); } */
.hero-unit { background-color: #fff; }
.center { display: block; margin: 0 auto; }
</style>
</head>
<body>
<div class="navbar navbar-fixed-top navbar-inverse">
<div class="navbar-inner">
<div class="container">
<a class="btn btn-navbar" data-toggle="collapse" data-target=".nav-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</a>
<a class="brand">Grade Manager</a>
<div class="nav-collapse collapse">
<ul class="nav pull-right">
<li><a href="contact_form.php">Contact Us</a></li>
<li class="divider-vertical"></li>
<li class="dropdown">
<a class="dropdown-toggle" href="#" data-toggle="dropdown">Log In <strong class="caret"></strong></a>
<div class="dropdown-menu" style="padding: 15px; padding-bottom: 0px;">
<form action="main.php" method="post">
Username:<br />
<input type="text" name="username" value="<?php echo $submitted_username; ?>" />
<br /><br />
Password:<br />
<input type="password" name="password" value="" />
<br /><br />
<input type="submit" class="btn btn-info" value="Login" />
</form>
</div>
</li>
</ul>
</div>
</div>
</div>
</div>
</div>
<div class="container hero-unit">
<h1>INVITE ONLY</h1>
<h3>Contact Us To Get Invited</h3>
</div>
</body>
</html>
2 Answers
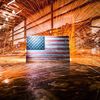
Andrew Shook
31,709 PointsCheck the end of your config.php file and make sure there isn't any whitespace or new lines at the end of the file. If there is, php will send that as content to the browser. Once php starts sending content to the browser, you won't be able to redirect the page response. As a rule of thumb, if you have a php file that is only being included in other file, like a config file, you should leave out the closing php tag:
?>
This will help prevent unintentional whitespace.
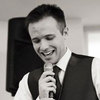
Jeremy Menicucci
19,227 PointsHello,
First, I would suggest a more secure session than just using the stock function "session_start".
Below is a pretty common secure session. You can find it on wikihow http://www.wikihow.com/Create-a-Secure-Login-Script-in-PHP-and-MySQL.
function start_session() {
$session_name = 'sec_session_id'; // Set a custom session name
$secure = SECURE;
// This stops JavaScript being able to access the session id.
$httponly = true;
// Forces sessions to only use cookies.
if (ini_set('session.use_only_cookies', 1) === FALSE) {
header("Location: ../error.php?err=Could not initiate a safe session (ini_set)");
exit();
}
// Gets current cookies params.
$cookieParams = session_get_cookie_params();
session_set_cookie_params($cookieParams["lifetime"],
$cookieParams["path"],
$cookieParams["domain"],
$secure,
$httponly);
// Sets the session name to the one set above.
session_name($session_name);
session_start(); // Start the PHP session
session_regenerate_id(); // regenerated the session, delete the old one.
}
That way, instead of calling "session_start()" just call "start_session()" as defined above for a more secure session.
I reproduced your code and it seems like the problem is in this line of code right here:
for($round = 0; $round < 65536; $round++){
$check_password = hash('sha256', $check_password . $row['salt']);
}
I'm not exactly sure what the effect of this is supposed to do, but you've essentially taken the password the user has entered and hashed it successfully before this code but then rehashed it 65,536 times. The resulting $check_password variable will always be different than the hashed password stored in your database and the hashed password that the user has entered. That essentially will always prevent any actual user from logging in. Once I removed that code I was able to "login".
Bare in mind I haven't really troubleshooted the rest of the code but I would start by removing this "for" loop. Let me know what happens next and I take a closer look at the code if you're still having problems.
EDIT: Also keep in mind that you're currently posting to the "main.php" page with your form and you're redirecting to the "main.php" page. I'm sure you'd want to change that so that you'd post to a login script and then redirect to the page your intending to access if the login is successful.
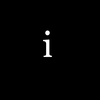
Furquan Ahmad
5,148 PointsOk thank you, going to try that today. Will let you know how it goes
Furquan Ahmad
5,148 PointsFurquan Ahmad
5,148 PointsHi, this is the configuration file
Andrew Shook
31,709 PointsAndrew Shook
31,709 PointsYou need to remove :
echo "Connected successfully";
If you echo anything out before you try to redirect with a header you will not be able to redirect. This is because php will automatically send a header when it echoes something out.