Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial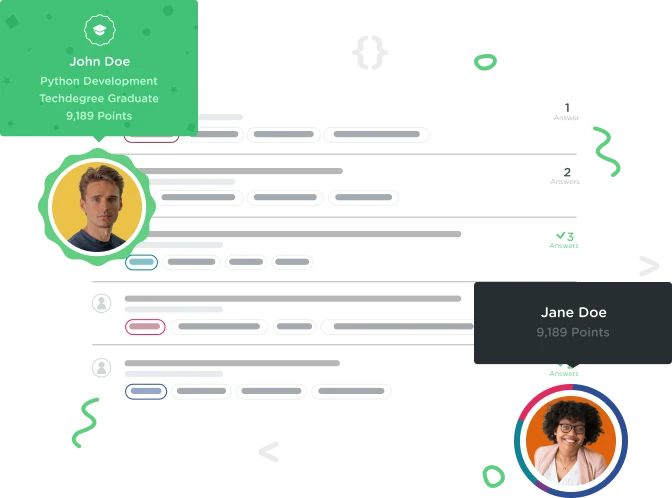

Conor Kelly
Courses Plus Student 223 PointsI'm stuck trying to define a function to return a value. How would you create a function to return a value?
def square(number):
number(5 * 5)
return
1 Answer
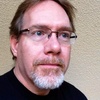
Chris Freeman
Treehouse Moderator 68,423 PointsHey Conor Kelly, the return, statement will return the result of an expression. If no expression is provided, a default None
is returned.
So if number
was set to a result of, say, 5 * 5
then the return statement could be return number
, or more directly, return 5 * 5
Post back if you need more help. Good luck!!!
Conor Kelly
Courses Plus Student 223 PointsConor Kelly
Courses Plus Student 223 PointsOkay, but say the return value is asking for it to be passed through. What do they mean by that?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsIn the phrase “* return the square of the value that was passed in.*”, the value passed in is the value passed through the parameter
number
.A refresher on the terminology:
Given a function definition
And a function call:
The following terminology is correct:
func
is called with the argumentarg1
arg1
is passed into the functionfunc
param1
receives the argumentarg1
In reality
param1
andarg1
are simply labels that point to objects in Python memory. When the function call is made,param1
is simply pointed to the same object thatarg1
points to.In the case of Treehouse code challenges, the challenge checker is exercising your code and is calling (and passing in) arguments to your function. You only need to know that the parameter you’ve defined (for example
number
) will receive arguments passed in by the checker.Post back if you need more help. Good luck!!!