Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial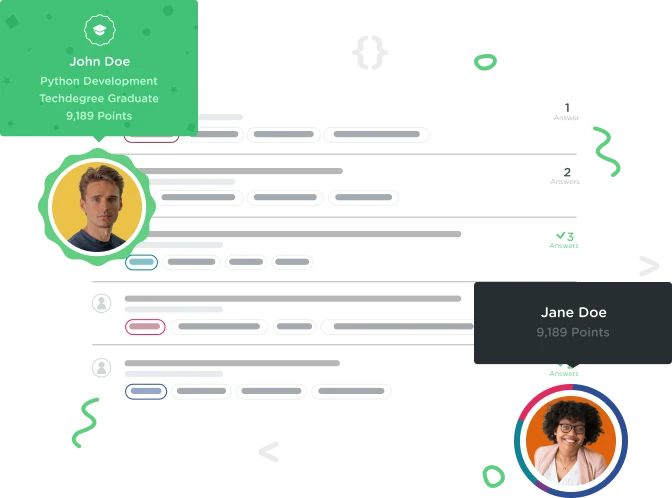

sarahmoore4
6,980 PointsI'm super stuck and I have no idea what to do!!! I would love to see someone's code with this
// Display the prompt dialogue while the value assigned to `secret` is not equal to "sesame"
let secret = prompt("What is the secret password?");
// This should run after the loop is done executing
alert("You know the secret password. Welcome!");
do {
secret !== "sesame";
} while (secret === "sesame")
2 Answers
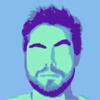
Cameron Childres
11,820 PointsHey Sarah~
First I'll add some comments to your code to detail what it's doing, then I'll show you my solution.
// Shows a prompt for the password and sets variable `secret` to the user supplied value
let secret = prompt("What is the secret password?");
// Shows an alert that they know the password
alert("You know the secret password. Welcome!");
// Runs the code inside do{} once, then continues to run as long as secret is 'sesame'
do {
secret !== "sesame";
} while (secret === "sesame")
Notice that no matter what the user sets "secret" to, the alert will always show. Following that the code inside of do{} is comparing "secret" to the password, but doesn't actually DO anything. Keep your comparisons inside of while() and put the code you want to run inside of do{}.
Here is my solution:
// Declare `secret` variable in global scope so the do...while function has something to set
let secret;
// Shows a prompt for the password once and sets variable `secret` to the user supplied value.
// Then checks if `secret` is NOT exactly the same as "sesame". If `secret` does not match, restart loop.
// If `secret` DOES match, while() evaluates as false, and the loop will end.
do {
secret = prompt("What is the secret password?");
} while (secret !=='sesame')
// Shows an alert that they know the password. Runs only after the do...while has ended.
alert("You know the secret password. Welcome!");
Let me know if you have any questions :)
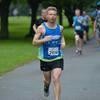
Rupert Travers
4,652 PointsExcellent, that was a little 'loopy' to get one's head around, thanks for sharing!
sarahmoore4
6,980 Pointssarahmoore4
6,980 PointsThanks so much this really cleared up a bunch for me I really appreciate the insight and help!