Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial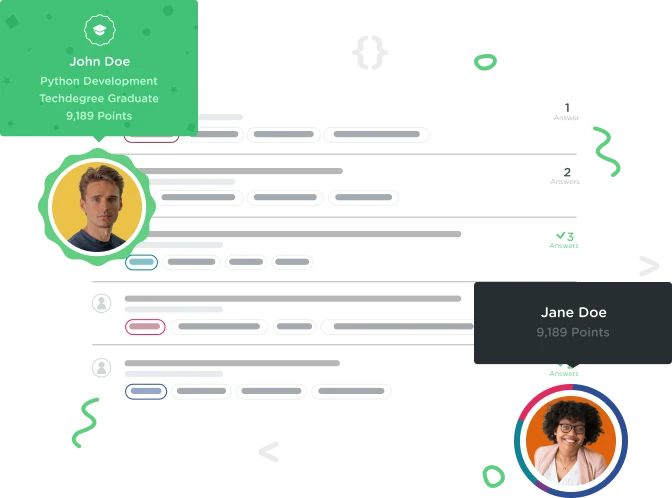
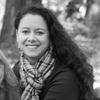
Jennifer Sherwood
896 PointsI'm sure I'm missing something obvious on this disemvowel task.
The program will run but it's returning the same letters it starts with, so the vowels are not being removed but I can't figure out why. I also just realized I need to check for upper and lower case, but none of the vowels are being removed.
I even stepped away and came but but still can't see what's wrong.
def disemvowel(word):
word_list = list(word)
vowels = ["a", "e", "i", "o", "u"]
for letters in word_list:
try:
word_list.remove(vowels)
except ValueError:
pass
return "".join(word_list)
7 Answers
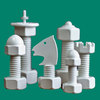
Steven Parker
231,545 PointsThe "remove" method takes a single argument, but in this code it is being passed the entire list of vowels. This is not an error, but it would only remove an item that was also the same entire list of vowels. You'll probably want to check for and remove each vowel separately. Also remember that "remove" only takes away the first match.
It's also not a good idea to alter an iterable while it is controlling a loop, that can cause items to be completely skipped over. One fix is to use a copy of the iterable to control the loop.
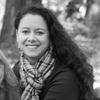
Jennifer Sherwood
896 PointsI'm still not getting it so I'm asking for one more pointer. I've read up on the copy method but it's not clicking for me. I know I could find another way to do it, but I'd like to understand the copy method rather than just working around it.
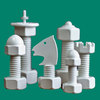
Steven Parker
231,545 PointsThe copy()
method (or the slice) both replicate the list so that the loop will still go through each element while you make changes. Without the copy, when the body of the loop removes an element, the sudden change in index values can cause the next element to be skipped over.
Did that answer your question?
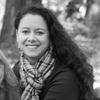
Jennifer Sherwood
896 PointsIt makes sense - I just don't know how to write it. I added the "word_list.copy()" but got the same results. I feel like the copy needs to be named somewhere or I need to tell the function to return the copy instead of the original but I don't know how to write it.
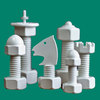
Steven Parker
231,545 PointsThe copy is probably correct. I'd bet you still have some of the other issues to deal with. For more explicit help, show your complete code as it is now.
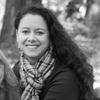
Jennifer Sherwood
896 Pointsimport copy
def disemvowel(word): word_list = list(word) vowels = ["a", "e", "i", "o", "u","A", "E", "I", "O", "U"] for letter in word_list.copy(): if letter in vowels: word_list.remove(letter) return "".join(word_list)
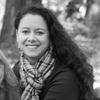
Jennifer Sherwood
896 PointsThat obviously didn't format correctly
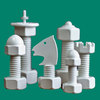
Steven Parker
231,545 PointsUhnless you have an indentation issue which can't be seen without formatting, this new code should pass the challenge (and you don't need to "import copy")
Use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
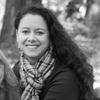
Jennifer Sherwood
896 PointsI got: Bummer: Hmm, got back letters I wasn't expecting! Called disemvowel('zPUxQsCOu') and expected 'zPxQsC'. Got back 'zPUxQsCOu'
I even tried a different browser just in case.
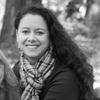
Jennifer Sherwood
896 Pointsdef disemvowel(word):
word_list = list(word)
vowels = ["a", "e", "i", "o", "u","A", "E", "I", "O", "U"]
for letter in word_list.copy():
````if letter in vowels:
````````return "".join(word_list)
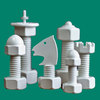
Steven Parker
231,545 PointsThe marks you need for formatting are 3 accents (```), not apostrophes ('''). And they go on lines by themselves before and after the code, but not on or between individual code lines.
And this code does pass the challenge when properly indented.
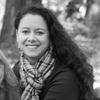
Jennifer Sherwood
896 PointsI thought if there was an error in the indentation, I would get an error to that extent. Therefore when I didn't, I thought the program was saying the indentation was correct. Lesson learned for me.
I just played with the indenting until it worked. I passed. Thanks for your patience and help with learning it. I took notes along the way.
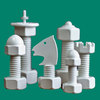
Steven Parker
231,545 PointsIndentation errors might be shown if they make the code invalid. But if the indentation is changing what the program does in a way that is still valid, you will get an incorrect result but no error.
Happy coding!
Jennifer Sherwood
896 PointsJennifer Sherwood
896 PointsFirst paragraph - makes perfect sense. I understand that error.
Second paragraph - I understand the theory of what you're saying but blanking on how to create a copy and update the original. Can I get a clue?
Steven Parker
231,545 PointsSteven Parker
231,545 PointsHere's two ways to iterate with a copy: