Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial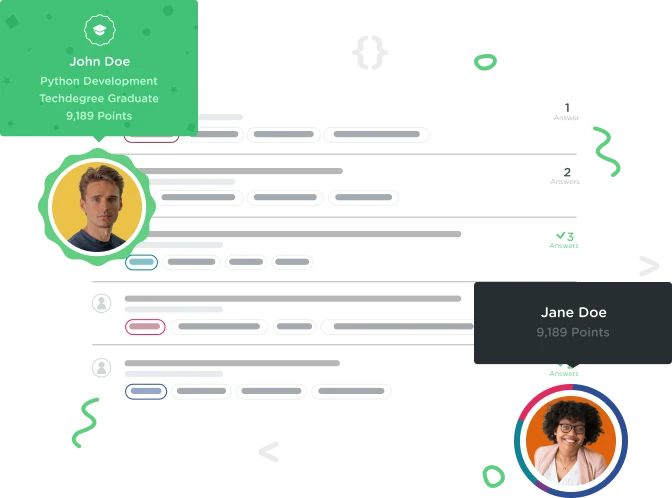

Christopher Mayfield
19,928 PointsI'm totally stuck on the swift closures challenge.
Not sure what to do next, can someone give me a code chunk so i can understand better.
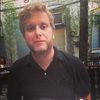
Alexander Bussey
11,162 PointsI'm still confused why you wouldn't just pass the integers along to the differencebetweenNumbers function in the first place...

Daniel Rosensweig
5,074 PointsNathan --
Your code works in XCode but not at the challenge because it is in fact valid code. It runs without errors, but it doesn't satisfy the requirements of the challenge. Your new function is supposed to return the result of the inner function. So, just add: -> Int to your function declaration, just before the curly brace, and add the word 'return' to the code inside your function.
Alexander -- There's essentially no reason in this case to pass the whole function instead of just running the inner function. The reason is for you to learn how to do it. However, there is value in the concept as applied in other situations, like the filter() function Pasan shows us. Do you understand why it's useful there?
3 Answers
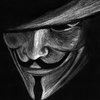
jack daniels
Courses Plus Student 8,079 Pointsfunc differenceBetweenNumbers(a: Int, b:Int) -> (Int) {
return a - b
}
// this is our code
//challenge 1
func mathOperation(differenceBetweenNumbers: (Int, Int) -> Int, a: Int, b: Int) -> Int{
return differenceBetweenNumbers(a,b)
}
//challenge 2
let difference = mathOperation(differenceBetweenNumbers, 4,8)
Drew Carver
5,189 PointsI don't understand why I can't get through this challenge. I have the same code as jack daniels above and it compiles fine in Xcode:
func differenceBetweenNumbers(a: Int, b: Int) -> (Int) {
return a - b
}
func mathOperation(mathFunction: (Int, Int) -> (Int), a: Int, b: Int) -> (Int) {
return mathFunction(a, b)
}
let difference = mathOperation(differenceBetweenNumbers, 1, 2)

Daniel Rosensweig
5,074 PointsDrew, I see just one difference between your code and Jack Daniels'. You have parentheses around (Int) --- the one where you tell what the inner function returns, inside mathOperation. Also where you tell what the outer function returns.
I'm not honestly positive why this is a problem here and NOT a problem in the top differenceBetweenNumbers function. I'd have thought you just weren't supposed to put the return type in parentheses, the way I've always done it, except that they did that at the top without errors. It might have something to do with higher order vs. regular functions? One way or the other, though, if I cut and paste your code in, and then remove those parentheses, the challenge works.

Nate Bird
7,999 PointsFYI, in Xcode 9.2 (Swift 2.1) the compiler is asking for the parameters to be named like so:
let difference = mathOperation(differenceBetweenNumbers, a: 4, b: 8)
Note the ‘a’ and ‘b’ in the parameter list.

nopcoder
8,605 PointsThanks for this hint, i was struggling with this way too long =)
Nathan Bivens
14,460 PointsNathan Bivens
14,460 PointsThis is what I have right now in the code challenge. It's telling me I have it wrong, but it's not telling me why. I have checked the Developer Docs that they gave as a link. I have ran it through Xcode, and it works fine. It's returning any two numbers I put in when calling the mathOperation function, and then I call the above function as the first parameter, then any two numbers I can think up into the second and third parameters.
So I am stuck here wondering what the issue is as well.
// This is what they gave at the beginning. //
func differenceBetweenNumbers(a: Int, b: Int) -> (Int) { return a - b }
// Newly created function //
func mathOperation(mathFunc: (Int, Int) -> Int, a:Int, b:Int) { mathFunc(a, b) }
I took the advice from the Developer Docs for the return type on that second function to include "a" & "b".
Any help would be great!