Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial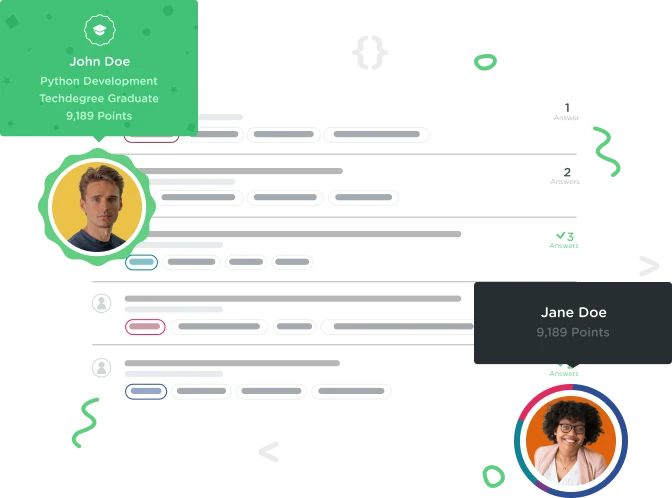
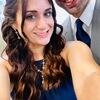
Rocco Cammarere
4,377 PointsI'm trying to create a list from tuples. I put together the code and tested offline but it wont work here
I have the following lists: list1 = [1, 2, 3] list2 = ["a", "b", "c"]
I added the lists together with the following function:
Define function combo which returns tuple
def combo(list1, list2): # Create tuple of each list tuple1 = (list1, list2)
# Create tuple of each element in lists
tuple2 = zip(list1,list2)
return tuple2
tuple2 offline gives the following: [(1, 'a'), (2, 'b'), (3, 'c')]
What am I doing wrong?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
# Define two iterables to pass to function combo
list1 = [1, 2, 3]
list2 = ["a", "b", "c"]
# Define function combo which returns tuple
def combo(list1, list2):
# Create tuple of each list
tuple1 = (list1, list2)
# Create tuple of each element in lists
tuple2 = zip(list1,list2)
return tuple2
# Access functions to get outputs
tuple2 = combo(list1, list2)
2 Answers
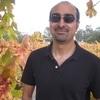
Kourosh Raeen
23,733 PointsHi Rocco - The zip() function returns an iterator of tuples. To create a list of tuples just pass what zip() returns to list():
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(list1, list2):
return list(zip(list1, list2))
You can also do this without the zip function with a loop:
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
# If you use .append(), you'll want to pass it a tuple of new values.
def combo(list1, list2):
my_tuples_list = []
for index in range(len(list1)):
my_tuples_list.append((list1[index], list2[index]))
return my_tuples_list
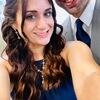
Rocco Cammarere
4,377 PointsThank you Kourosh!
What is the difference between an iterable & list? I thought a list was a sub category of iterable.
In addition, if I make the change you suggested, the challenge is happy, but in IDLE, I see no difference in output from what I had without adding list().
Is there something I'm missing?