Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial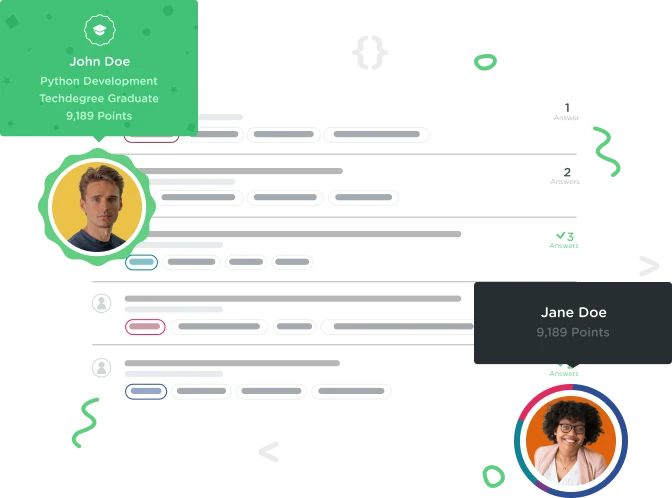
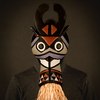
Giorgi Kiknadze
Courses Plus Student 2,768 PointsI'm trying to make weather app with Node.JS but it doesn't work!
I'm trying to get API from http://api.openweathermap.org/ but I have some problem and can you help me?
Here's app.js
//Problem: Make a weather app that will recive current wether with country and postal code
//Solve: Get API from http://openweathermap.org/ and make interactive search for weather using console
var http = require('http');
//Print message
function printWeather(city, weather) {
var message = 'In ' + city + ', there is ' + weather + ' degrees.';
console.log(message);
}
//Print out error messages
function printError(error) {
console.error(error.message);
}
//Connect to the API URL api.openweathermap.org/data/2.5/weather?q={city name},{country code}
function get(city)
var request = http.get('api.openweathermap.org/data/2.5/weather?q='+ city + '&units=metric', function(response) {
var body = "";
//Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if (response.statusCode === 200) {
try {
//Parse the data
var weatherAPI = JSON.parse(body);
//Print the data
printWeather(weatherAPI.name, weatherAPI.sys.country, weatherAPI.id);
} catch(error) {
//Parse error
printError(error);
}
} else {
//Status Code error
printError({message: 'There was an error getting the weather from ' + city + '. (' + http.STATUS_CODES[response.statusCode] + ')'});
}
})
});
//Connection error
request.on('error', printError);
}
module.exports.get = get;
and here weathe.js
var getWeather = require('./app.js');
1 Answer
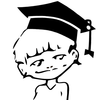
simhub
26,543 Pointshi Giogri, do not forget [http://] on [var request=http.get('http://....) ]
Also, since you provided a 'city'- parameter on your function.
You could change the export module a bit.
app.js
//Problem: Make a weather app that will recive current wether with country and postal code
//Solve: Get API from http://openweathermap.org/ and make interactive search for weather using console
var http = require('http');
//Print message
function printWeather(city, weather) {
var message = 'In ' + city + ', there is ' + weather + ' degrees.';
console.log(message);
}
//Print out error messages
function printError(error) {
console.error(error.message);
}
//Connect to the API URL api.openweathermap.org/data/2.5/weather?q={city name},{country code}
module.exports =function get(city){
var request = http.get('http://api.openweathermap.org/data/2.5/weather?q='+ city + '&units=metric', function(response) {
var body = '';
//Read the data
response.on('data', function(chunk) {
body += chunk;
});
response.on('end', function() {
if (response.statusCode === 200) {
try {
//Parse the data
var weatherAPI = JSON.parse(body);
//Print the data
printWeather(weatherAPI.name, weatherAPI.main.temp);
} catch(error) {
//Parse error
printError(error);
}
} else {
//Status Code error
printError({message: 'There was an error getting the weather from ' + city + '. (' + http.STATUS_CODES[response.statusCode] + ')'});
}
})
});
//Connection error
request.on('error', function (err) {
printError(err);
});
};
weather.js
var getWeather = require('./app.js')('London');