Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial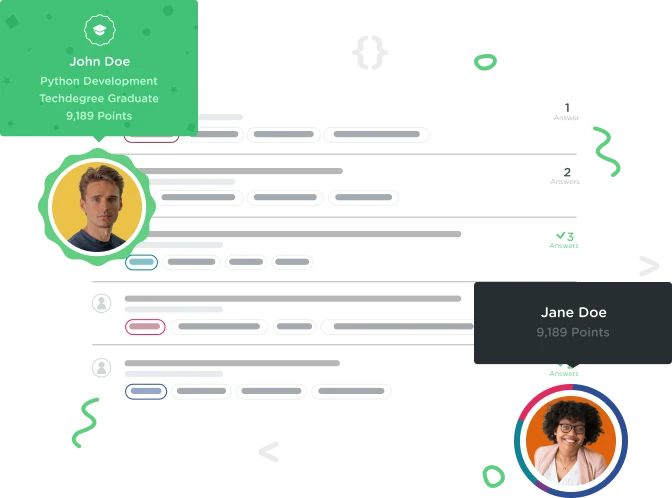
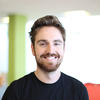
Kristian Woods
23,414 PointsI'm trying to understand the $_GET function in PHP
I'm trying to wrap my head around this $_GET function
1) If you add a question mark at the end of a URL e.g. "?category=books" - the word after the question mark - "category", becomes a variable and the word "books", becomes its value
2) The function takes a string variable name - $_GET["category"]
3) The function isset(), checks for a variable "category" in the URL
4) If the variable exists in the URL, the $_GET function takes the value from the variable - in this case, "books"
5) You can then use a conditional statement to see what the value of "category" is, and run a code block
<?php
if(isset($_GET["category"])) {
if($_GET["category"] == 'books') {
$pageTitle = 'Books';
} else if ($_GET["category"] == 'music') {
$pageTitle = 'Music';
} else if ($_GET["category"] == 'movies') {
$pageTitle = 'Movies';
}
}
?>
is this correct?
thanks
2 Answers

Miguel Canas
Python Development Techdegree Student 11,470 PointsHi Kristian,
You're definitely on the right track but just to clarify a few things:
I'm trying to wrap my head around this $_GET function
-
$_GET
is not a function, it's an associative array.
1) If you add a question mark at the end of a URL e.g. "?category=books" - the word after the question mark - "category", becomes a variable and the word "books", becomes its value
- Yes.
2) The function takes a string variable name - $_GET["category"]
- Looping back to the first statement,
$_GET
is an associative array, not a function. An array holds a collection of variables, where as a function is for executing a block of code.
3) The function isset(), checks for a variable "category" in the URL
- Yes, mostly.
isset(...)
takes any variable you pass to it, and determines if it's value is NULL or not. For example:
/* given the url http://www.mywebsite.com/mypage.php?category=books */
isset($_GET["category"]); // returns true, because category is a parameter that gets passed to the $_GET array
isset($_GET["foobar"]); // returns false, because foobar is not a parameter, so it does not exist in the $_GET array
isset($myVar); // returns false because $myVar is not defined.
4) If the variable exists in the URL, the $_GET function takes the value from the variable - in this case, "books"
- Yep, but to clarify the terminology: "If the parameter exists in the URL, the $_GET array takes the value from the parameter"
5) You can then use a conditional statement to see what the value of "category" is, and run a code block
- Precisely!
Here are some documentation links on the relevant topics:
Hope that's helpful.
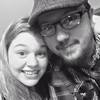
Nicholas Mercer
2,319 PointsKristian,
You're 100% correct and that code will work without any issues! :) If you need help modifying it or adding more, let me know.
-Nick