Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial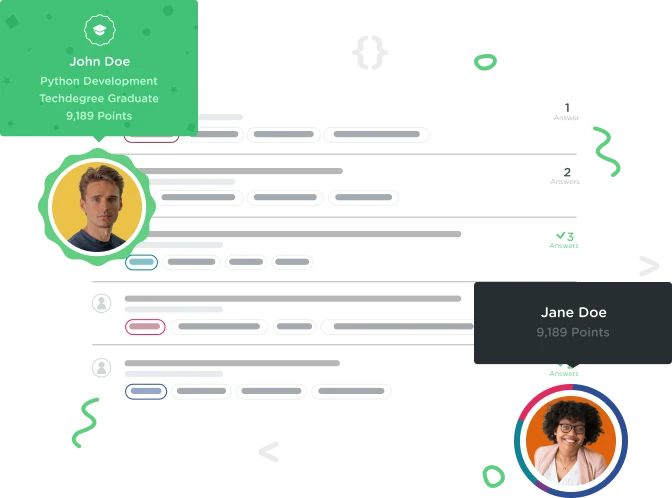
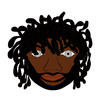
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsI'm working on an app for practice
Hi guys, so I'm still practicing. Recently I asked how to generate a random day. I took it further and generated many random things. Then I took it further and generated a random color and number or random clothing item and random color. here's the code.
const myClothing = [];
const myColors = [];
var html = '';
//This is to capture users clothing items
while ( true ) {
let item = prompt("Enter your clothing items. Type 'finished' to quit");
if ( item === 'finished') {
break;
}
myClothing.push(item);
}
//This is to capture colors the user is wearing
while (true) {
let color = prompt("Enter the colors you're wearing. Type 'finished' to quit.")
if ( color === 'finished') {
break;
}
myColors.push(color);
}
let clothingItem = Math.floor(Math.random() * myClothing.length);
let color = Math.floor(Math.random() * myColors.length);
const allItems = [myColors[color], myClothing[clothingItem]];
let choice = Math.floor(Math.random() * allItems.length);
So this will use the prompt dialog to ask users to enter the clothing items want and then store it in an array and the same thing for colors.
The app will then generate a random clothing item and a random color then that will be stored in another array and then finally print either a random color or clothing. I got that to work no problem.
I remembered we did a course where we had to generate a random color, so I wanted to generate a random color instead of text. I got it to do that but I added a div to the page with the random color and each time you click the button it adds another div. I wanted to first remove the first div and replace it with the newly generated div. Can't figure out how to do that. Please help.
Here's the code.
const myClothing = [];
const myColors = [];
var html = '';
//This is to capture users clothing items
while ( true ) {
let item = prompt("Enter your clothing items. Type 'finished' to quit");
if ( item === 'finished') {
break;
}
myClothing.push(item);
}
//This is to capture colors the user is wearing
while (true) {
let color = prompt("Enter the colors you're wearing. Type 'finished' to quit.")
if ( color === 'finished') {
break;
}
myColors.push(color);
}
var dice = {
myClothing: myClothing.length,
myColors: myColors.length,
item: function () {
let randomItem = Math.floor(Math.random() * myClothing.length);
return myClothing[randomItem];
},
color: function () {
let red = Math.floor(Math.random() * 256);
let green = Math.floor(Math.random() * 256);
let blue = Math.floor(Math.random() * 256);
let rgb = 'rgb(' + red + ',' + green + ',' + blue + ')';
html += '<div style="background-color:' + rgb + '; height:100px; width: 100px; "></div>';
return html;
}
}
This app collects and generates a random item and color.
function printNumber(number) {
var placeholder = document.querySelector(".item-placeholder");
placeholder.innerHTML = number;
}
function printColor(color) {
var placeholder = document.querySelector(".color-placeholder");
placeholder.innerHTML = color;
}
var itemButton = document.querySelector(".item-button");
var colorButton = document.querySelector(".color-button");
itemButton.onclick = function() {
var item = dice.item();
printNumber(item);
};
let colorPlaceholder = document.querySelector("p.color-placeholder");
let div = colorPlaceholder.children;
colorButton.onclick = function() {
var color = dice.color();
printColor(color);
};
I tried this at one point.
function printNumber(number) {
var placeholder = document.querySelector(".item-placeholder");
placeholder.innerHTML = number;
}
function printColor(color) {
var placeholder = document.querySelector(".color-placeholder");
// placeholder.style.backgroundColor = 'red';
// placeholder.innerHTML = color;
placeholder.innerHTML = '<div style="background-color:blue; height:100px; width: 100px; display:block "></div>';
}
var itemButton = document.querySelector(".item-button");
var colorButton = document.querySelector(".color-button");
itemButton.onclick = function() {
var item = dice.item();
printNumber(item);
};
let colorPlaceholder = document.querySelector("p.color-placeholder");
let div = colorPlaceholder.children;
colorButton.onclick = function() {
// if (colorPlaceholder.value < 1) {
var color = dice.color();
printColor(color);
colorPlaceholder.firstElementChild.display = 'block';
if (colorPlaceholder.firstElementChild.display == 'block') {
colorPlaceholder.removeChild(div);
}
// }
};
Here's the html
<html>
<head>
<title>Dice Simulator 2015</title>
<link rel="stylesheet" href="css/styles.css">
</head>
<body>
<p class="item-placeholder">
</p>
<button class="item-button">Roll Dice</button>
<p class="color-placeholder">
</p>
<button class="color-button">Roll Dice</button>
<script src="js/dice-strip-tease.js"></script>
<script src="js/my-ui-strip-tease.js"></script>
</body>
</html>
and the CSS
* {
font-family: Helvetica, Arial, sans-serif;
text-align: center;
}
button {
background-color: rgb(82, 186, 179);
border-radius: 6px;
border: none;
color: rgb(255, 255, 255);
padding: 10px;
text-transform: uppercase;
width: 200px;
cursor: pointer;
}
.item-placeholder,
.color-placeholder {
height: 100px;
width: 100px;
padding: 50px;
margin: 50px auto;
border: 1px solid gray;
border-radius: 10px;
font-size:2.5em;
text-align: center;
}
This is from the dice simulator course I changed to work with my app.
1 Answer
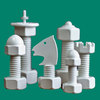
Steven Parker
231,007 Points Hi there! I got alerted by your tag.
The reason the div's build up is that the "html" is being appended instead of replaced:
html += '<div style="background-color:' + rgb + '; height:100px; width: 100px; "></div>';
// ^ use a normal "=" instead of "+=" to replace the div instead of add on a new one
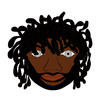
SAMUEL LAWRENCE
Courses Plus Student 8,447 PointsHi Luc de Brouwer and Steven Parker I was able to figure it out this morning. Luc your reply really helped. I sat for a long while looking at the code and the html trying to figure out what I really wanted to change. It turns out I didn't really needed to add the html div because there is already a <p> element as a placeholder. All I really wanted was to style the <p> element with the random color generated. In my printColor function the placeholder I targeted the innerHTML which is a text element. So I changed it to target the placeholder background-color property instead. It turns out that was all I needed to do.
function printColor(color) {
var placeholder = document.querySelector(".color-placeholder");
// placeholder.innerHTML = rub; this is a text element so it was showing me the text
placeholder.style.background = color;
Steven as for the += I think you helped me with a problem like this some time ago also where I was getting a console error because I used += when I should have used just an =. Also I didn't need to add the div since I wanted the entire background styled with the random color. So I removed the html div. Your solution also works but I didn't need it. So I deleted that line of code.
Thanks so much guys.
var dice = {
myClothing: myClothing.length,
// myColors: myColors.length,
item: function () {
let randomItem = Math.floor(Math.random() * myClothing.length);
return myClothing[randomItem];
},
color: function () {
let red = Math.floor(Math.random() * 256);
let green = Math.floor(Math.random() * 256);
let blue = Math.floor(Math.random() * 256);
let rgb = 'rgb(' + red + ',' + green + ',' + blue + ')';
return rgb;
}
}
I also rewrote the code to use the colors the user entered to generate a random color from instead of just a random color generator. So it captures a color keyword and styles the background with it.
const myClothing = [];
const myColors = [];
var colorPlaceholder = document.querySelector(".color-placeholder");
//This is to capture users clothing items
while ( true ) {
let item = prompt("Enter your clothing items. Type 'finished' to quit");
if ( item === 'finished') {
break;
}
myClothing.push(item);
}
//This is to capture colors the user is wearing
while (true) {
let color = prompt("Enter the colors you're wearing. Type 'finished' to quit.")
if ( color === 'finished') {
break;
}
myColors.push(color);
}
var dice = {
item: function () {
let randomItem = Math.floor(Math.random() * myClothing.length);
return myClothing[randomItem];
},
color: function () {
let randomColor = Math.floor(Math.random() * myColors.length);
return myColors[randomColor];
}
}
Check out the changes to see the effect I was going for.
Luc de Brouwer
Full Stack JavaScript Techdegree Student 17,939 PointsLuc de Brouwer
Full Stack JavaScript Techdegree Student 17,939 PointsHi Samuel, first of all, amazing what you've achieved so far! You did a great job exploring the possibilities and trying the code out. However, I am not able to help you with javascript further than the basics because that's where my knowledge in JS ends.
If I would try to remove a div from javascript I'd ask myself what I would need to achieve that. e.g. "what syntax requires removing elements in JS", "how do I target my div", "Is it unique, do I target the div by ID, Class or its inner content". Questions like these should be able to help you figure out how to solve the challenge you are facing right now.