Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial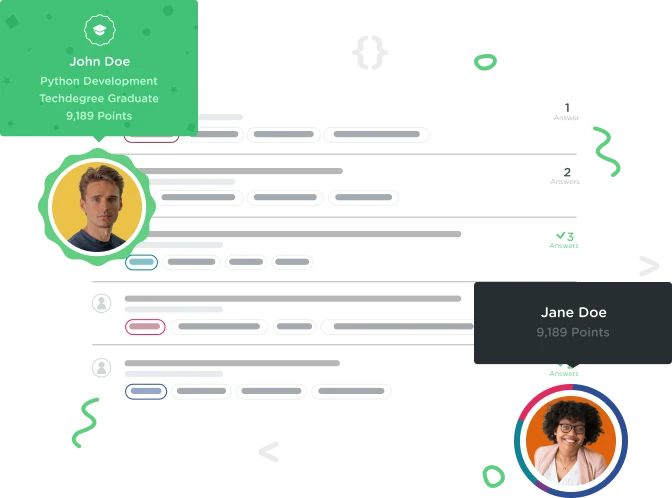

jun cheng wong
17,021 Pointsimage not displayed on html
Pet Images that are added not shown on index.html and pet.html, is there any possible issue?
# models.py
from flask import Flask
from flask_sqlalchemy import SQLAlchemy
import datetime
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///pets.db'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Pet(db.Model):
id = db.Column(db.Integer, primary_key=True)
# timestamp, name it 'created' type is datetime, default value is current time
created = db.Column('Created', db.DateTime, default=datetime.datetime.now)
# remember do not include () after now
name = db.Column('Name', db.String(50))
# inside String(), put in parameter to set a word limit, empty for no limit
age = db.Column('Age', db.String())
breed = db.Column('Breed', db.String())
color = db.Column('Color', db.String())
size = db.Column('Size', db.String())
weight = db.Column('Weight', db.String())
url = db.Column('URL', db.String())
url_tag = db.Column('Alt Tag', db.String())
pet_type = db.Column('Pet Type', db.String())
gender = db.Column('Gender', db.String())
spay = db.Column('Spay', db.String())
house_trained = db.Column('House Trained', db.String())
description = db.Column('description', db.Text())
def __repr__(self):
return f'''<Pet (Name: {self.name}
Age: {self.age}
Breed: {self.breed}
Color: {self.color}
Size: {self.size}
Weight: {self.weight}
URL: {self.url}
URL tag: {self.url_tag}
Pet Type: {self.pet_type}
Gender: {self.gender}
Spay: {self.spay}
House Trained: {self.house_trained}
Description: {self.description}'''
#app.py
from flask import render_template, url_for, request, redirect
from models import db, Pet, app
@app.route('/') # request
def index():
pets = Pet.query.all()
return render_template('index.html', pets=pets) # response/
# go into the template folder and grab a file called index.html and send it to user as a response
@app.route('/addpet/', methods=['GET', 'POST']) # Get the addpet page and post it to the user
def addpet():
if request.form:
new_pet = Pet(name=request.form['name'], age = request.form['age'],
breed=request.form['breed'], color = request.form['color'],
size = request.form['size'], weight=request.form['weight'],
url=request.form['url'], url_tag=request.form['alt'],
pet_type=request.form['pet'], gender=request.form['gender'],
spay=request.form['spay'], house_trained=request.form['housetrained'],
description=request.form['description'])
db.session.add(new_pet)
db.session.commit()
return redirect(url_for('index'))
return render_template('addpet.html')
@app.route('/pet/<id>') # pass id from 1 page to another
def pet(id):
pet = Pet.query.get_or_404(id)
return render_template('pet.html', pet=pet)
@app.route('/pet/edit/<id>', methods=['GET', 'POST'])
def edit_pet(id):
pet = Pet.query.get(id)
if request.form:
pet.name = request.form['name']
pet.age = request.form['age']
pet.breed = request.form['breed']
pet.color = request.form['color']
pet.size = request.form['size']
pet.weight = request.form['weight']
pet.url = request.form['url']
pet.url_tag = request.form['alt']
pet.pet_type = request.form['pet']
pet.gender = request.form['gender']
pet.spay = request.form['spay']
pet.house_trained = request.form['housetrained']
pet.description = request.form['description']
db.session.commit()
return redirect(url_for('index'))
return render_template('editpet.html', pet=pet)
@app.route('/delete/<id>')
def delete_pet(id):
pet = Pet.query.get_or_404(id)
db.session.delete(pet)
db.session.commit()
return redirect(url_for('index'))
@app.errorhandler(404)
def not_found(error):
return render_template('404.html', msg=error), 404
if __name__ == '__main__':
db.create_all()
app.run(debug=True, port=8000, host='127.0.0.1')
# index.html
{% extends 'layout.html' %}
{% block button %}
<a class="add-pet-btn" href="{{ url_for('addpet') }}" alt="Add Pet">
<i class="fas fa-plus"></i>
Pet
</a>
{% endblock %}
{% block content %}
<section id="pet-cards">
{% for pet in pets %}
<a href="{{ url_for('pet', id=pet.id) }}">
<div class="card">
<img src="{{ pet.url }}" alt="{{ pet.url_tag }}">
<h2>{{ pet.name }}</h2>
<h3>{{ pet.age }}</h3>
<p>{{ pet.description }}</p>
</div>
</a>
{% endfor %}
</section>
{% endblock %}
# addpet.html
{% extends 'layout.html' %}
{% block button %}
<a class="back-btn" href="{{ url_for('index') }}">
<i class="fas fa-caret-left"></i>
Back
</a>
{% endblock %}
{% block content %}
<section id="pet-cards">
<form action="{{ url_for('addpet') }}" method='POST'>
<div class="columns">
<div class="col1">
<div class="form-container">
<label for="name">Name</label>
<input id="name" name="name" type="text" placeholder="Fuzzy Wuzzy">
</div>
<div class="form-container">
<label for="age">Age</label>
<input id="age" name="age" type="text" placeholder="6 months">
</div>
<div class="form-container">
<label for="breed">Breed</label>
<input id="breed" name="breed" type="text" placeholder="American Shorthair">
</div>
<div class="form-container">
<label for="url"><i class="fas fa-camera"></i> URL</label>
<input id="url" name="url" type="text" placeholder="Photo link">
</div>
</div>
<div class="col2">
<div class="form-container">
<label for="color">Color</label>
<input id="color" name="color" type="text" placeholder="Grey">
</div>
<div class="form-container">
<label for="size">Size</label>
<input id="size" name="size" type="text" placeholder="Medium">
</div>
<div class="form-container">
<label for="weight">Weight</label>
<input id="weight" name="weight" type="text" placeholder="5lbs">
</div>
<div class="form-container">
<label for="alt">Image Alt Text</label>
<input id="alt" name="alt" type="text" placeholder="This photo is...">
</div>
</div>
</div>
<div class="columns">
<div class="col1">
<fieldset>
<div>
<legend>Pet Type</legend>
<input id="dog" type="radio" name="pet" value="Dog">
<i class="fas fa-check-circle"></i>
<label for="dog">Dog</label>
<input id="cat" type="radio" name="pet" value="Cat">
<i class="fas fa-check-circle"></i>
<label for="cat">Cat</label>
<input id="other" type="radio" name="pet" value="Other">
<i class="fas fa-check-circle"></i>
<label for="other">Other</label>
</div>
</fieldset>
<fieldset>
<div>
<legend>Gender</legend>
<input id="female" type="radio" name="gender" value="Female">
<i class="fas fa-check-circle"></i>
<label for="female">Female</label>
<input id="male" type="radio" name="gender" value="Male">
<i class="fas fa-check-circle"></i>
<label for="male">Male</label>
</div>
</fieldset>
</div>
<div class="col2">
<fieldset>
<div>
<legend>Spayed/Neutered</legend>
<input id="true" type="radio" name="spay" value="Yes">
<i class="fas fa-check-circle"></i>
<label for="true">Yes</label>
<input id="false" type="radio" name="spay" value="No">
<i class="fas fa-check-circle"></i>
<label for="false">No</label>
<input id="na" type="radio" name="spay" value="Unknown">
<i class="fas fa-check-circle"></i>
<label for="na">Unkown</label>
</div>
</fieldset>
<fieldset>
<div>
<legend>Housetrained</legend>
<input id="yes" type="radio" name="housetrained" value="Yes">
<i class="fas fa-check-circle"></i>
<label for="yes">Yes</label>
<input id="no" type="radio" name="housetrained" value="No">
<i class="fas fa-check-circle"></i>
<label for="no">No</label>
<input id="unkown" type="radio" name="housetrained" value="Unknown">
<i class="fas fa-check-circle"></i>
<label for="unkown">Unkown</label>
</div>
</fieldset>
</div>
</div>
<fieldset id="descr">
<div>
<legend>Description</legend>
<label for="description" style="display: none;">Description</label>
<textarea name="description" id="description" cols="30" rows="10" placeholder="This pet is..."></textarea>
</div>
</fieldset>
<div id="form-buttons">
<button type="reset">Cancel</button>
<button type="submit">Submit</button>
</div>
</form>
</section>
<script src='/static/js/app.js'></script>
{% endblock %}
# pet.html
{% extends "layout.html" %}
{% block button %}
<a class="add-pet-btn" href="{{ url_for('addpet') }}" alt="Add Pet">
<i class="fas fa-plus"></i>
Pet
</a>
{% endblock %}
{% block content %}
<section id="pet-data">
<img src="{{ pet.url }}" alt="{{ pet.url_tag }}">
<div id="pet-name">
<h2>Name</h2>
<p>{{ pet.name }}</p>
</div>
<div id="left-col">
<div>
<h2>Age</h2>
<p>{{ pet.age }}</p>
</div>
<div>
<h2>Breed</h2>
<p>{{ pet.breed }}</p>
</div>
<div>
<h2>Color</h2>
<p>{{ pet.size }}</p>
</div>
<div>
<h2>Size</h2>
<p>{{ pet.size }}</p>
</div>
</div>
<div id="right-col">
<div>
<h2>Weight</h2>
<p>{{ pet.weight }}</p>
</div>
<div>
<h2>Gender</h2>
<p>{{ pet.gender }}</p>
</div>
<div>
<h2>Spayed/Neutered</h2>
<p>{{ pet.spray }}</p>
</div>
<div>
<h2>Housetrained</h2>
<p>{{ pet.house_trained }}</p>
</div>
</div>
<div id="description">
<h2>Description</h2>
<p>{{ pet.description }}</p>
</div>
</section>
<section id="edit-delete">
<a href="{{ url_for('edit_pet', id=pet.id) }}">
<i class="far fa-edit"></i>Edit
</a>
<a href="{{ url_for('delete_pet', id=pet.id) }}">
<i class="far fa-trash-alt"></i>Delete
</a>
</section>
{% endblock %}
1 Answer
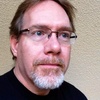
Chris Freeman
Treehouse Moderator 68,457 PointsTry confirming the url paths are what you expected. Can you hard code a path that works? For debug, try this
<p>{{ pet.url }}</p>
<p> add hard coded path to image that works </p>
jun cheng wong
17,021 Pointsjun cheng wong
17,021 PointsHi Chris, I do not know how to hard coding, but I can view the picture if I use online url instead of uploading my own image
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsWhat I mean by “hard coding” is using literal strings for values instead of variable. “Hard” in the sense of unchanging.
So, is there a static string url that points to where the uploaded images reside.
Also checkout this StackOverflow post for ideas.
jun cheng wong
17,021 Pointsjun cheng wong
17,021 Pointsalright thanks