Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial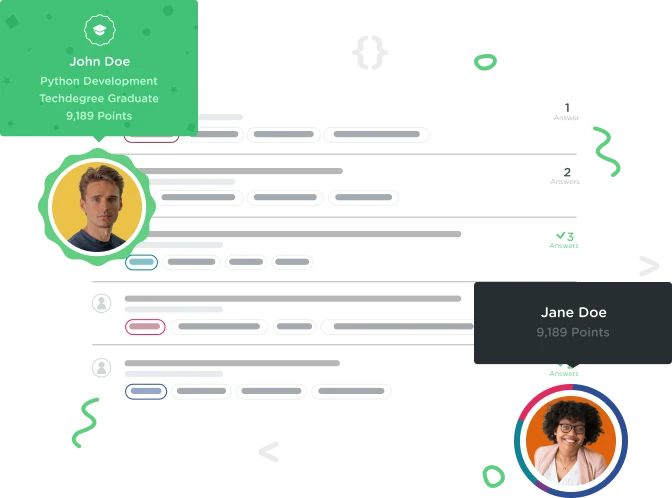

Matthew Walavich
176 PointsImage sequence
Looking to be able to scroll through a sequence of image using JQuery while the mouse is over the image. I have 61 images in a container div and have all of them hidden except one. I would like to use jquery to be able to scroll through the images only when the mouse is over the image.
<div id="container">
<img src="frames/noindex_basset_02_img000.jpg" class="animated">
<img src="frames/noindex_basset_02_img001.jpg" class="animated">
<img src="frames/noindex_basset_02_img002.jpg" class="animated">
<img src="frames/noindex_basset_02_img003.jpg" class="animated">
<img src="frames/noindex_basset_02_img004.jpg" class="animated">
<img src="frames/noindex_basset_02_img005.jpg" class="animated">
<img src="frames/noindex_basset_02_img006.jpg" class="animated">
<img src="frames/noindex_basset_02_img007.jpg" class="animated">
<img src="frames/noindex_basset_02_img008.jpg" class="animated">
<img src="frames/noindex_basset_02_img009.jpg" class="animated">
<img src="frames/noindex_basset_02_img010.jpg" class="animated">
<img src="frames/noindex_basset_02_img011.jpg" class="animated">
<img src="frames/noindex_basset_02_img012.jpg" class="animated">
<img src="frames/noindex_basset_02_img013.jpg" class="animated">
<img src="frames/noindex_basset_02_img014.jpg" class="animated">
<img src="frames/noindex_basset_02_img015.jpg" class="animated">
<img src="frames/noindex_basset_02_img016.jpg" class="animated">
<img src="frames/noindex_basset_02_img017.jpg" class="animated">
<img src="frames/noindex_basset_02_img018.jpg" class="animated">
<img src="frames/noindex_basset_02_img019.jpg" class="animated">
<img src="frames/noindex_basset_02_img020.jpg" class="animated">
<img src="frames/noindex_basset_02_img021.jpg" class="animated">
<img src="frames/noindex_basset_02_img022.jpg" class="animated">
<img src="frames/noindex_basset_02_img023.jpg" class="animated">
<img src="frames/noindex_basset_02_img024.jpg" class="animated">
<img src="frames/noindex_basset_02_img025.jpg" class="animated">
<img src="frames/noindex_basset_02_img026.jpg" class="animated">
<img src="frames/noindex_basset_02_img027.jpg" class="animated">
<img src="frames/noindex_basset_02_img028.jpg" class="animated">
<img src="frames/noindex_basset_02_img029.jpg" class="animated">
<img src="frames/noindex_basset_02_img030.jpg" class="animated">
<img src="frames/noindex_basset_02_img031.jpg" class="animated">
<img src="frames/noindex_basset_02_img032.jpg" class="animated">
<img src="frames/noindex_basset_02_img033.jpg" class="animated">
<img src="frames/noindex_basset_02_img034.jpg" class="animated">
<img src="frames/noindex_basset_02_img035.jpg" class="animated">
<img src="frames/noindex_basset_02_img036.jpg" class="animated">
<img src="frames/noindex_basset_02_img037.jpg" class="animated">
<img src="frames/noindex_basset_02_img038.jpg" class="animated">
<img src="frames/noindex_basset_02_img039.jpg" class="animated">
<img src="frames/noindex_basset_02_img040.jpg" class="animated">
<img src="frames/noindex_basset_02_img041.jpg" class="animated">
<img src="frames/noindex_basset_02_img042.jpg" class="animated">
<img src="frames/noindex_basset_02_img043.jpg" class="animated">
<img src="frames/noindex_basset_02_img044.jpg" class="animated">
<img src="frames/noindex_basset_02_img045.jpg" class="animated">
<img src="frames/noindex_basset_02_img046.jpg" class="animated">
<img src="frames/noindex_basset_02_img047.jpg" class="animated">
<img src="frames/noindex_basset_02_img048.jpg" class="animated">
<img src="frames/noindex_basset_02_img049.jpg" class="animated">
<img src="frames/noindex_basset_02_img050.jpg" class="animated">
<img src="frames/noindex_basset_02_img051.jpg" class="animated">
<img src="frames/noindex_basset_02_img052.jpg" class="animated">
<img src="frames/noindex_basset_02_img053.jpg" class="animated">
<img src="frames/noindex_basset_02_img054.jpg" class="animated">
<img src="frames/noindex_basset_02_img055.jpg" class="animated">
<img src="frames/noindex_basset_02_img056.jpg" class="animated">
<img src="frames/noindex_basset_02_img057.jpg" class="animated">
<img src="frames/noindex_basset_02_img058.jpg" class="animated">
<img src="frames/noindex_basset_02_img059.jpg" class="animated">
<img src="frames/noindex_basset_02_img060.jpg" class="animated">
</div>
#container {
position: fixed;
overflow: hidden;
}
.animated {
position: absolute;
display: none;
top: 0;
left: 0;
}
$(document).ready(function () {
var pictureCount = $('#container img').length;
var scrollResolution = 70;
animateImage();
function animateImage() {
var currentScrollPosition = window.pageYOffset;
var imageIndex = Math.round(currentScrollPosition / scrollResolution);
if (imageIndex >= pictureCount) {
imageIndex = pictureCount - 1; // Select last image
}
$("#container img").hide();
$("#container img").eq(imageIndex).show();
}
$(window).on('scroll', function() {
animateImage();
}); ```

Matthew Walavich
176 PointsPosted my code and yes I have done the lightbox course
3 Answers
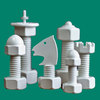
Steven Parker
230,274 PointsFor some reason this didn't work for me until I removed the "position: absolute;
" setting. It made sense that it didn't need it, as only one photo is visible at a time. But I still don't understand why having it on made nothing visible. Without access to the actual image files, I had to make up some but I wouldn't expect that to affect the operation.
Anyway, I was able to limit the scroll to only when the mouse was over the image container by adding this:
$("#container").on("mouseover", _=> document.body.style.overflow = "scroll");
$("#container").on("mouseout", _=> document.body.style.overflow = "hidden");

Matthew Walavich
176 PointsThat solves part of my problem and thank you. I am still looking for it to only scroll through the pictures when the mouse is over the image, not scroll the page.
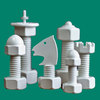
Steven Parker
230,274 PointsBut your selection mechanism is based on window.pageYOffset
. Isn't the page scroll how your scroll through the images now?

Matthew Walavich
176 PointsThat's the part I can't figure out and I'm sure it is an easy fix and right under my thumb. I don't want a page scroll but a scroll within the container div to scroll the pictures
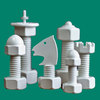
Steven Parker
230,274 PointsAre all the photos the same size? If so, you could do this in CSS with no JavaScript needed:
#container {
position: fixed;
overflow-y: scroll;
width: /* photo width + 20px for scroll bar */;
height: /* photo height */;
}
The container will have it's own scroll bar but be fixed on the screen.

Matthew Walavich
176 PointsYes they are all the same size
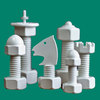
Steven Parker
230,274 PointsThat simple CSS should do the trick, then.
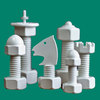
Steven Parker
230,274 PointsMatthew Walavich — so did that work?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsPlease show your code or link to a snapshot, and provide a link to the course page if the question relates to a course.
Have you already done the course where you construct a "lightbox"?