Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial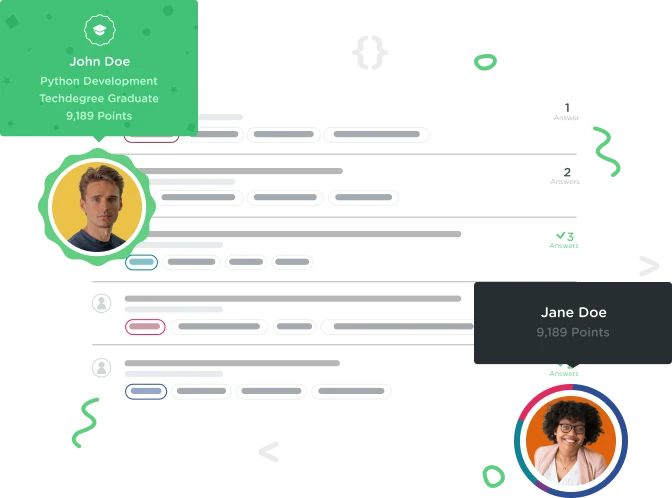
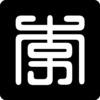
lihaoquan
12,045 PointsImperative vs Declarative
What is the differences between Imperative and Declarative approach for attaching data to HTML nodes?
gregsmith5
32,615 Pointsgregsmith5
32,615 PointsImperative and declarative are two general approaches to programming that you may see outside of DOM manipulation, so keep that in mind while I try to give a good explanation. Also, these examples are contrived and not necessarily best practice.
Imperative programming is what you are already familiar with in JavaScript as it's all we're taught in the beginning. Let's say you have an array full of employee objects:
These need to be output, somehow, to a webpage. Take a moment to think about how you would do this in JavaScript.
With vanilla JavaScript, I might do something like this:
This is imperative because I'm telling the computer all of the steps I want it to follow in order to complete a task I have in mind. What other options do we have? Angular.js has a lot of functions that are already built-in which enable us to perform these actions with very little input.
Depending on how much Angular you know, this may or may not make sense to you. Basically, we're access a collection of objects with ng-repeat, and Angular is copying that middle bit and automagically filling in the information we want - the employee's name and title. This is declarative because I'm not telling the computer what steps to perform in order to do this. I'm just telling Angular what I want done, and where I want it done. Angular takes care of all the rest.
Hope this helps!