Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial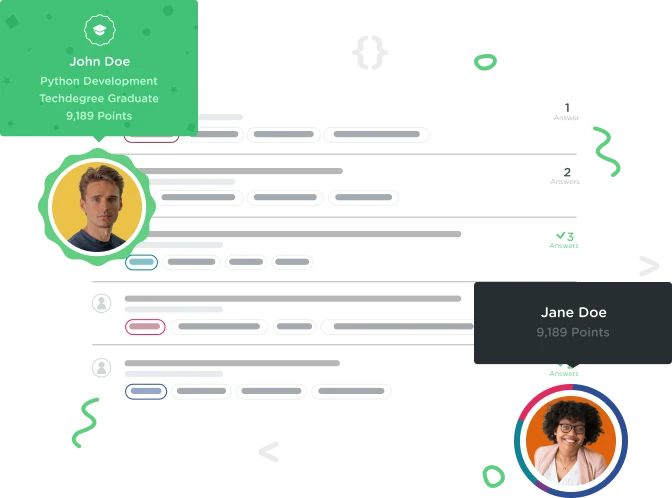
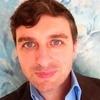
Arsene Lavaux
4,342 PointsImplement Instagram logout on swipe
Hi Treehousers,
I am looking to implement a logout of the current user (logged into Instagram since I use this social API) based on a swipe behavior.
Here is my code:
UITapGestureRecognizer *swipe = [[UITapGestureRecognizer alloc] initWithTarget:self action:@selector(logout)];
[self.view addGestureRecognizer:swipe];
Now I need to implement the logout method in my view controller:
-(void)logout
{ //my code here to logout from Instagram
}
Would anyone know how to achieve this? I couldn't find anything super clear on the web so far.
Thanks!
6 Answers
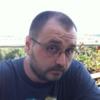
Robert Bojor
Courses Plus Student 29,439 PointsHi Arsene,
You need to implement a swipe gesture like this:
-(void) ViewDidLoad {
[super viewDidLoad];
UISwipeGestureRecognizer *swipeLeft = [[UISwipeGestureRecognizer alloc] initWithTarget:self action:@selector(logout)];
swipeLeft.numberOfTouchesRequired = 1; // The number of touches that must be present for the swipe gesture to be recognized.
swipeLeft.direction = UISwipeGestureRecognizerDirectionLeft;
[self.view addGestureRecognizer:swipeLeft];
}
-(void) logout{
/* Logout logic */
}
You can also send the event to the method you are going to call. This will allow you to gain a bit of control over the gesture.
-(void) ViewDidLoad {
[super viewDidLoad];
UISwipeGestureRecognizer *swipeLeft = [[UISwipeGestureRecognizer alloc] initWithTarget:self action:@selector(logout:)];
swipeLeft.numberOfTouchesRequired = 1; // The number of touches that must be present for the swipe gesture to be recognized.
swipeLeft.direction = UISwipeGestureRecognizerDirectionLeft;
[self.view addGestureRecognizer:swipeLeft];
}
-(void) logout:(UISwipeGestureRecognizer *)event {
if (event.state == UIGestureRecognizerStateBegan) {
/* Do something when the swipe begins */
}
if (event.state == UIGestureRecognizerStateEnded) {
/* Complete logout when its finished. */
}
}
The other states are: UIGestureRecognizerStateCancelled, UIGestureRecognizerStateChanged, UIGestureRecognizerStateFailed, UIGestureRecognizerStatePossible, UIGestureRecognizerStateRecognized.
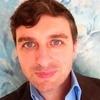
Arsene Lavaux
4,342 PointsHi Robert: Thank you for your great insight.
What I am really interested in is how to logout from Instagram api...
Not so sure how to handle that part.
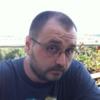
Robert Bojor
Courses Plus Student 29,439 PointsYou should delete the token Instagram creates when you login and redirect the user to the login view.
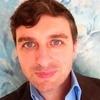
Arsene Lavaux
4,342 PointsNot so sure how to write the code for that. Thanks for your help Robert anyway. I do appreciate it.
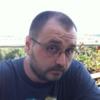
Robert Bojor
Courses Plus Student 29,439 PointsHi Arsene,
It looks like there is someone else who figured this out, right here on Treehouse. Check this solve and see if it works for you:
https://teamtreehouse.com/forum/how-to-log-out-with-simpleauth-photo-bombers
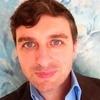
Arsene Lavaux
4,342 PointsHi Robert,
In viewWillAppear, I set up my logout button "X" by calling the following method:
-(void)displayLogoutButtonInNav
{
_logoutButton = [[UIBarButtonItem alloc] initWithTitle:@"X" style:UIBarButtonItemStylePlain target:self action:@selector(logout)];
self.navigationItem.leftBarButtonItem = _logoutButton;
}
Then, I implemented the logout method as per what you kindly shared with me:
- (void)logout
{
NSHTTPCookie *cookie;
NSHTTPCookieStorage *storage = [NSHTTPCookieStorage sharedHTTPCookieStorage];
for (cookie in [storage cookies])
{
NSString* domainName = [cookie domain];
NSRange domainRange = [domainName rangeOfString:@"instagram"];
if(domainRange.length > 0)
{
[storage deleteCookie:cookie];
}
}
}
When I run the app, the build is successful, the X button shows up, when I tap on it I can tell it changes state, perfect. However, the logout doesn't work, I stay logged into Instagram since I can use the other view controllers of my app in the exact same way as when I am logged in.
Would you have any further insight to share with me?
Thanks!