Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial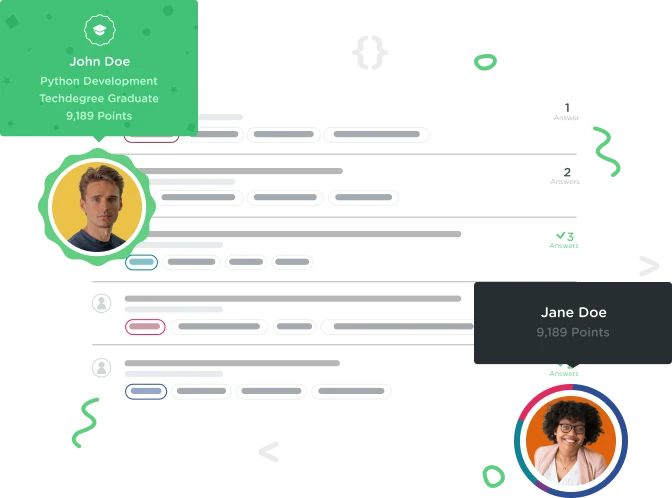
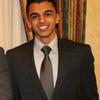
abhisheksharma
6,136 PointsImplementing geotagging in an application?
Hi all,
I wanted to know if there are any good guides out there to implement geotagging within an iOS application.
To be a bit more specific: I just wanted to ask the user if it's okay to use their location, and if they press "Yes", their location would pop up on the map.
I've tried using Parse to follow their guide but I'm getting stuck with that. Google hasn't been of much help either.
Thanks in advance!
1 Answer
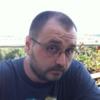
Robert Bojor
Courses Plus Student 29,439 PointsHi Abhishek,
You will need to use the MapKit Framework and you can check the code below, which will show a temp location until the user's location is updated from the GPS.
// ViewController.h
#import <UIKit/UIKit.h>
#import <MapKit/MapKit.h>
@interface ViewController : UIViewController <MKMapViewDelegate>
@property (weak, nonatomic) IBOutlet MKMapView *mapView;
@end
// ViewController.m
#import "ViewController.h"
// Default location until user's location is updated. New York for example
#define NY_LATITUDE 40.754445
#define NY_LONGITUDE -73.977364
// The span and distance for the region setting
#define SPAN_VALUE 1.0f
#define DISTANCE 500
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Show a predefined region until the user location is returned
MKCoordinateRegion region;
region.center.latitude = NY_LATITUDE;
region.center.longitude = NY_LONGITUDE;
region.span.latitudeDelta = SPAN_VALUE;
region.span.longitudeDelta = SPAN_VALUE;
[self.mapView setRegion:region animated:YES];
[self.mapView setDelegate:self];
[self.mapView setShowsUserLocation:YES];
}
- (void)mapView:(MKMapView *)mapView didUpdateUserLocation:(MKUserLocation *)userLocation {
CLLocationCoordinate2D loc = [userLocation coordinate];
MKCoordinateRegion region = MKCoordinateRegionMakeWithDistance(loc, DISTANCE, DISTANCE);
[self.mapView setRegion:region animated:YES];
}
@end
abhisheksharma
6,136 Pointsabhisheksharma
6,136 PointsThank you, Robert! Another question, would this have to be implemented in a different view controller?
Robert Bojor
Courses Plus Student 29,439 PointsRobert Bojor
Courses Plus Student 29,439 PointsYou can implement this in the view controller of your choice, provided there is a map included on that view controller. In the example I gave you, I used a clean view controller for example purposes, but you can use the code where ever you need to.