Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial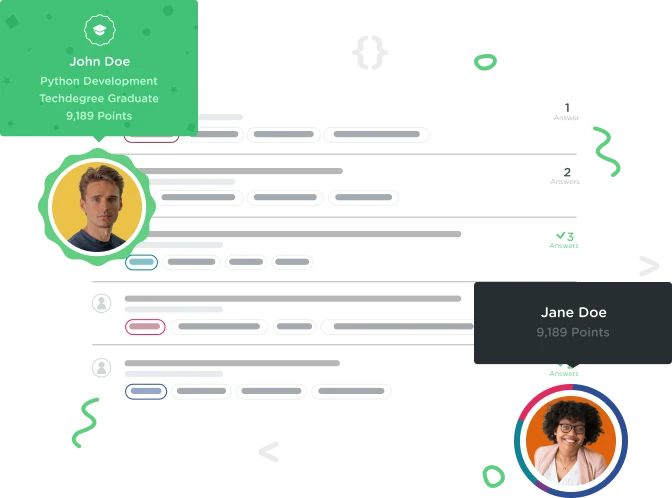

jonasdahms
19,331 PointsImplenting jquery in angular
Greetings! I currently have some problems implenting my jquery in angular. I have an old jquery script of mine I want to use in my current angular project and so far I only have partly found out how to implent it right. Simply requiring the jquery library before the angular library and then implenting the jquery script in one of the Controllers already works pretty fine. But not completly as it should be, the script doesnt work fully as in a non-angular enviroment .
Currently it look like this, I have this template I inject into my html
<div ng-Model="drawing()">
<canvas id="drawing" width="500" height="350"></canvas>
</div>
and the related controller to this is
"use strict";
function drawingCtrl($scope, dataService) {
$scope.drawing = function(){
var curColour = "rgb(255, 0, 0)";
var drawing = false;
var outsideDrawing = false;
var $canvas = $("canvas");
var $body = $("body");
var context = $canvas[0].getContext("2d");
var lastEvent;
$body.mousedown(function() {
outsideDrawing = true;
}).mouseup(function() {
outsideDrawing = false;
})
$canvas.mousedown(function(e) {
drawing = true;
outsideDrawing = true;
context.beginPath();
context.moveTo(e.offsetX,e.offsetY);
context.arc(e.offsetX,e.offsetY,1,0*Math.PI,2*Math.PI)
context.strokeStyle = curColour;
context.lineWidth = 1;
context.stroke();
lastEvent = e;
}).mousemove(function(e) {
if (drawing && outsideDrawing) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = curColour;
context.lineWidth = 3;
context.stroke();
lastEvent = e;
}
}).mouseup(function() {
outsideDrawing = false;
drawing = false;
}).mouseleave(function() {
drawing = false;
}).mouseenter(function(e) {
drawing = true;
lastEvent = e;
});
};
};
module.exports = drawingCtrl;
Some of you might recognize this script, its a modified version of the drawing script in one of the jquery courses. Also yes I know this script can be refined and improved, I will do that once I have got it implented successfully =)
Anyway my current problem are 2 things:
- Iam unsure which ng I should use in the template, currently I use ng-Model which lets the script work 95% fine but leaves me with this nasty error message in the log http://puu.sh/ouZ9T/eda3942a99.png .
- Should I "angularfy" the script? In the sense of that I change all the mouse events I currently have to ng-Mousedown etc. in the html and then rewrite the script so the singular events are bound to the related ng's in the html? If yes, how do I do that? So far all my tries angularfying the script have resulted in the script not working anymore.
Personally I think I just havent found the right ng yet I should use or setup it right, but enlighten me with your ideas please.
Help would be greatly appreciated,
1 Answer
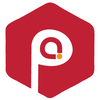
Andrei Petre
14,894 PointsDon't use Angular and jQuery together. Angular comes by default with a minified version of jQuery which contains a bunch of jQuery functions, called jQlite (read Angular docs). If you want to manipulate DOM in Angular, you should use directives. Also, don't export you controller, like you did at the bottom of the file, register it in an angular module.