Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial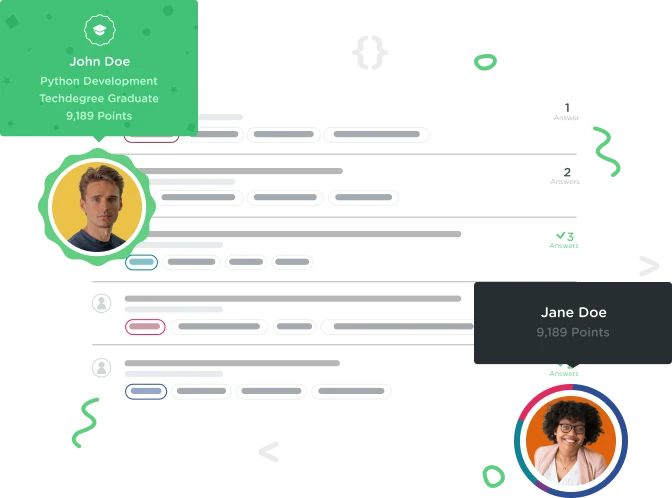
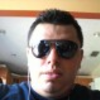
Eddie Flores
9,110 PointsImport Random Code Challenge
This is my code:
import random
user_list = [1,2,3,4,5,6]
def random_member(user_list):
num = random.randint(0,len(user_list)-1))
return user_list(num)
Got: "Task 1 is no longer passing".
also tried
import random
def random_member(user_list):
user_list = [1,2,3,4,5,6]
num = random.randint(0,len(user_list)-1)
return user_list(num)
I get "Bummer! TypeError: 'list' object is not callable"
I've tried different combinations and nothing works. The closest thing I've gotten it to work is by it saying WTF?!?
I tried someone else's code that is nearly identical in the track I went and it worked. I don't get why. The indentation I thought was fine. Please advise. I technically got the points for it, but I want to pass it on my own merit, not because I tested someone else's code.
It might be a flaw in the challenge or something, but on top of it not being specific enough and if you didn't have some knowledge of python prior I wouldn't have known what to do. Maybe this challenge should be reworded or something with more specific criteria.
2 Answers
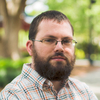
Kenneth Love
Treehouse Guest TeacherYour solution looks good except for one problem.
List indexes are done with square brackets [ ]
, not parentheses ( )
. Your last line basically says that user_list
is a function that's being called instead of a list that's being accessed.
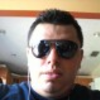
Eddie Flores
9,110 PointsI just went back and checked and I was able to reduce the code even further. I guess the list didn't need to be populated, just called on. I am sorry for giving you so much grief, I was just frustrated over such a silly mistake that took up so much of my time.
I managed to pass the challenge with just this amount of code below:
import random
def random_member(user_list):
num = random.randint(0,len(user_list)-1)
return user_list[num]
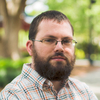
Kenneth Love
Treehouse Guest TeacherHa, sorry for the frustration. At least you'll be way more likely to remember this in the future! That's a good solution with one small style problem. You should always have a space after a comma, so random.randint(0, len(user_list)-1)
.
Eddie Flores
9,110 PointsEddie Flores
9,110 PointsWow... Thanks Kenneth. I have enjoyed the tutorial thus far. I hope to see your next one soon.
I've also done tutorials with python before, and I can definitely see the differences between 2.7 and 3.0 in python. I just want to say my thanks.
Although my constructive criticism about explaining the challenge a bit more would be taken into consideration.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherEddie Flores I am more than happy to reword the challenge. Any specific suggestions?
Eddie Flores
9,110 PointsEddie Flores
9,110 PointsWell through your tutorial, I've gone through it backwards and forwards the last few days making sure that with each coding challenge I learned everything I would need to get through it. There were some holes in the process.
For example, in the functions code challenge, I don't believe you mentioned anything about the built-in code for python. I have a friend on here that was taking this tutorial (which encouraged me to take it) that couldn't get passed that point. He didn't know that sum() was a built-in tool, and had no idea that it could be used, let alone necessary to be used for the challenge. I know we can do it in a for loop, but if you can at least to doing it that way people without experience won't have to get so frustrated. At least in the wording of the question reference to it.
(i.e.) Make a function named add_list that adds all of the items in a passed-in list together and returns the total. Assume the list contains only numbers.
to
Make a function named add_list that adds all of the items in a passed-in list together and returns the total. Assume the list contains only numbers. Use a for loop to pass it through the list to get your total.
That at least tells me to use a for loop and assign it to the variable total in order to get it through. I didn't even consider using a for loop to finish that challenge until I saw another post on the forum and it used the sum like I did, but you stated in the comments area that you rather it not use sum() but a for loop. I went back and did it, and it works. It's just a lot more work, not much explained, and it forces the students to read between imaginary lines that were not in any specific context.
As in this challenge for the importing.
Could be worded as:
Because if this challenge is anything like the last one, I would be assuming that the system will populate the list. One of my toughest parts of the challenge was realizing that I had to populate the list myself. That was.. until it kept giving me errors like 0 items detected or some thing. I have also thought that it might have been due to the error that I created, that it might have required a populated list, and still didn't work because it was in parenthesis and not brackets. I honestly haven't gone back and tried it with an empty list, but it would be nice to know if it were a bit more clear.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherThanks for the suggestions, Eddie Flores. I'll read through them more, but I wanted to point out that
sum()
isn't required and, really, isn't even expected to be use to complete the function.I've also left out some of these instructions, like "use a for loop" or "length of the list (minus one)" so that you're required to come up with those steps yourself. I want you to be thinking and I want things to be a little hard :). But I should probably go back and add a bit more explanation to some of these.
Thanks again!