Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial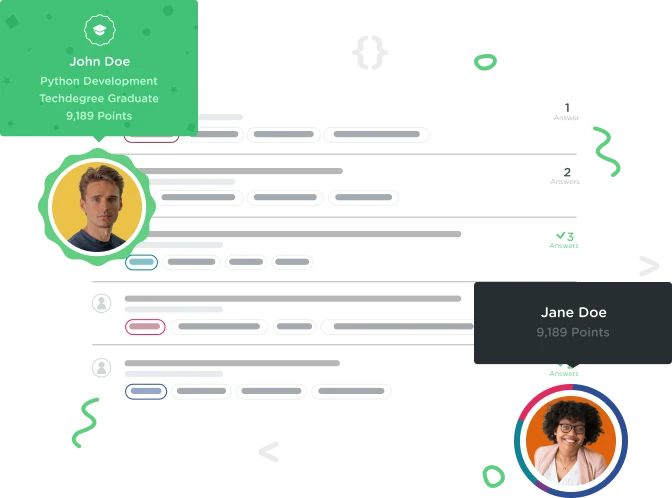

Daniel Salvatori
2,658 PointsImproving my random number guessing game
I created a code which will require a user to guess a random number between 1 and 5.
If they get it wrong I want the console to tell them to guess again, and keep track of how many guesses it took them.
Right now it works but i need to call the function each time.
Note this is for an assignment so it must be a function and must be done all in the console,.
Is there a better way to do this?
var randomNum = (Math.floor(Math.random()*5) +1);
var guesses = 1;
function guess(numberGuessed) {
if (numberGuessed != randomNum){
console.log('Sorry, keep guessing');
guesses += 1;
} else {
console.log("You are correct! It took you " + guesses + ' guesses.');
}
}
guess();
Thanks

Daniel Salvatori
2,658 PointsHi Gustavo,
I thought to use a loop but I dont know how I can do this without bringing up a prompt (which I don't want to do), i keep running into an endless loop.
Do I need to prompt the user in the "do" part? Or is there another way?
1 Answer

Collin Halliday
Full Stack JavaScript Techdegree Student 17,491 PointsDaniel,
Why don't you want to use a prompt? Although it is possible for a user to interact with your program as is without the use of a prompt (or other input mechanism), a prompt is a much cleaner and simpler option for your user. Also, if your user does not know javascript or how to interact with the browser's console, they will not be able to play your game.
Assuming your user does have the requisite knowledge, they could definitely play your guessing game through the console. However, you will want to make a change to your code for this to work. Simply remove your call to the guess function at the end of your js file. Otherwise, the user will be cheated out of their first guess as the guess function automatically runs when the page loads. That's it. Once you do that, preview your code in the browser, open up the browser's console, and for each guess, call the guess function directly inside the console, passing a different number as its argument each time (i.e. guess(2);). You can do this repeatedly and your program will keep track of the total number of guesses and print them to the console when you guess the number correctly.
If you choose to prompt the user, as Gustavo aptly pointed out, you want to use a do-while loop to keep your code running repeatedly until the user guesses the random number. In order for this to work, you'll need a guess variable to store the value of the user's guess. Right now you have a guess function that takes a numberGuessed as its argument, but no numberGuessed variable declared anywhere in your code.
If you choose not to prompt your user for a guess, you won't want to use a loop, because, as you have already experienced, that will result in an endless loop. When using a do-while loop in conjunction with a prompt, the loop will run until the while portion's conditional expression is true. The prompt allows the user the chance to keep trying to make that expression true with each guess. Without a prompt, your function will endlessly test the numberGuessed parameter of the guess function against the randomNum variable, unless the guess is correct on the first try.
One more comment. Due to your current code structure, each time you run your program initially, a random number will be stored in the randomNum variable and it will stay the same until you refresh your browser or close the program and run it again. If you want your random number to change after each guess, move your randomNum variable declaration and initialization to the top line inside of your guess function.
I hope that helps. Best of luck!

Daniel Salvatori
2,658 PointsThanks Collin,
Great, clear explanation, and i understand why in most cases a prompt and do while function would be better.
In this case, based on the parameters of my assignment I will keep it as is but your method is the better way going forward.
Gustavo Winter
Courses Plus Student 27,382 PointsGustavo Winter
Courses Plus Student 27,382 PointsHi Daniel, yes, there is a better way.
You need to put your function into a loop. The loop will start and just end when the condition match, that's in your case when the number match with the response.
You can do that with Do / While loop.
"Do" is for you start the loop and executing the function.
"While" is for you stay in the looping until the condition matchs.
This article can help you.
It's what you need to do this, but if you need help with the code, just let me know, and i'll help you.
Happy coding.